


Master the key skills for optimizing website access speed in Go language
Master the key skills of Go language website access speed optimization
In today's era of information explosion, users' patience has become increasingly limited, and they are unwilling to wait for the website to load for too long. Therefore, optimizing website access speed has become one of the important tasks for developers and operation and maintenance personnel. In view of the high performance and concurrency characteristics of Go language, some key tips will be introduced below to help developers optimize the access speed of websites written in Go language.
- Using concurrent processing
The Go language inherently supports concurrent programming and can take full advantage of the performance advantages of multi-core processors. In website development, we can use goroutine and channel to achieve concurrent processing. When processing operations that require time, you can put them into a goroutine for execution to avoid blocking other operations. In addition, using channels to coordinate data transfer between multiple goroutines can improve the response speed of the website.
The following is a simple sample code that demonstrates how to use goroutine and channel to handle multiple requests concurrently:
package main import ( "fmt" "net/http" ) func fetch(url string, ch chan<- string) { resp, err := http.Get(url) if err != nil { ch <- fmt.Sprintf("Error: %s", err) return } defer resp.Body.Close() ch <- fmt.Sprintf("%s: %d", url, resp.StatusCode) } func main() { urls := []string{"http://www.example.com", "http://www.google.com", "http://www.baidu.com"} ch := make(chan string) for _, url := range urls { go fetch(url, ch) } for range urls { fmt.Println(<-ch) } }
- Using connection pool
In Go language , the http package provides a connection pool by default, which can reuse connections to improve performance. However, the default connection pool size is limited. If the website has many concurrent requests, there may be insufficient connections. You can increase the size of the connection pool by setting the MaxIdleConnsPerHost parameter of Transport.
The following is a sample code that demonstrates how to modify the size of the connection pool:
package main import ( "fmt" "net/http" "time" ) func main() { transport := &http.Transport{ MaxIdleConnsPerHost: 100, IdleConnTimeout: 30 * time.Second, } client := &http.Client{ Transport: transport, } resp, err := client.Get("http://www.example.com") if err != nil { fmt.Printf("Error: %s ", err) return } defer resp.Body.Close() // 处理响应... }
- Static resource optimization
For static resources in the website, such as pictures, CSS and JavaScript files can be optimized by using a CDN (Content Delivery Network). CDN can distribute these static resources to the server closest to the user to improve the transmission speed.
In addition, in website development, static resources can be placed on a separate server, and web servers such as Nginx can be used to process and cache static resources. This can reduce the burden on the Go language server and improve the access speed of the website.
- Database query optimization
For large websites, database queries are often one of the performance bottlenecks. You can reduce the number of database queries by using caching. In the Go language, in-memory databases such as Redis can be used as caches to cache popular data or query results to avoid repeated database queries.
The following is a sample code that demonstrates how to use Redis to cache query results:
package main import ( "fmt" "time" "github.com/go-redis/redis" ) func expensiveQuery() string { // 模拟一个耗时的数据库查询操作 time.Sleep(1 * time.Second) return "result" } func main() { client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", DB: 0, }) result, err := client.Get("query_result").Result() if err == redis.Nil { result = expensiveQuery() client.Set("query_result", result, 10*time.Minute) fmt.Println("Query result from database") } else if err != nil { fmt.Printf("Error: %s ", err) return } else { fmt.Println("Query result from cache") } fmt.Printf("Result: %s ", result) }
By mastering the above key skills, developers can optimize the access speed of websites written in Go language . Of course, these are just some basic techniques, and actual optimization work needs to be refined according to specific circumstances. However, by using these techniques, developers can improve the user experience while maintaining the quality of the website.
The above is the detailed content of Master the key skills for optimizing website access speed in Go language. For more information, please follow other related articles on the PHP Chinese website!
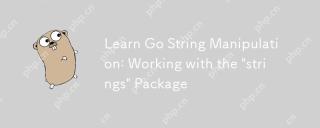
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
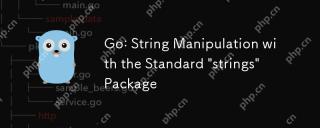
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
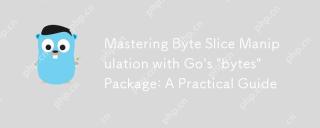
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
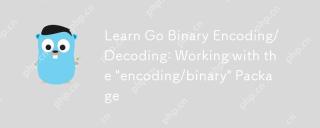
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
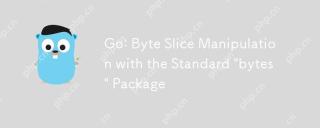
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
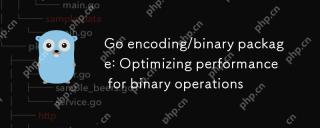
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
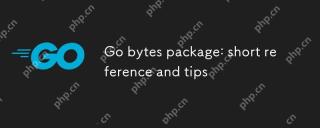
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
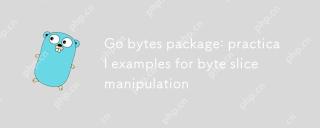
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
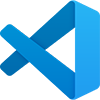
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
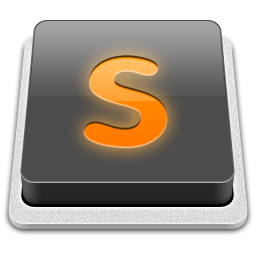
SublimeText3 Mac version
God-level code editing software (SublimeText3)
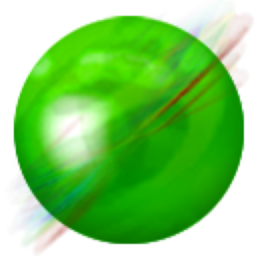
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
