


Practical case of mutual translation between Chinese and Indonesian using Java Baidu Translation API
A practical case of Java Baidu Translation API realizing mutual translation between Chinese and Indonesian
Overview
With the development of globalization and the increase of transnational exchanges, the demand for language translation is also increasing. Baidu Translation API is a powerful tool that can help us translate between different languages quickly and easily. This article will introduce how to use Java programming language to achieve mutual translation between Chinese and Indonesian through Baidu Translation API.
Implementation steps
- Register Baidu developer account
First, we need to register a Baidu developer account and apply to obtain the relevant key of the translation API so that it can be called in Java code API. On the Baidu Developer Platform, select Translation API and register an account to obtain the APP ID and key. - Import dependent libraries
In Java code, we need to use related classes such as HttpURLConnection and BufferedReader to interact with the API. Therefore, relevant dependent libraries need to be imported into the project. Add the following dependencies in the pom.xml file:
<dependencies> <dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>httpclient</artifactId> <version>4.5.13</version> </dependency> </dependencies>
- Writing API requests
In the Java code, we need to construct a URL to send the HTTP request and add the parameters and password The key is passed to the API. We can use the URLConnection class to achieve:
import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; import java.net.URLEncoder; public class TranslationAPI { public static void main(String[] args) { String sourceText = "中文文本"; // 需要翻译的中文文本 String appId = "your_app_id"; // 替换为你的APP ID String appKey = "your_app_key"; // 替换为你的APP Key try { String encodedText = URLEncoder.encode(sourceText, "UTF-8"); String urlStr = "http://api.fanyi.baidu.com/api/trans/vip/translate?q=" + encodedText + "&from=zh&to=id&appid=" + appId + "&salt=1435660288&sign=" + generateSign(sourceText, appId, appKey); URL url = new URL(urlStr); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); connection.setRequestMethod("GET"); BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream())); StringBuilder response = new StringBuilder(); String line; while ((line = reader.readLine()) != null) { response.append(line); } reader.close(); System.out.println(response.toString()); } catch (Exception e) { e.printStackTrace(); } } private static String generateSign(String sourceText, String appId, String appKey) { String sign = appId + sourceText + "1435660288" + appKey; MD5 md5 = new MD5(); return md5.getMD5(sign); } }
- Parse API response
API response is a JSON string that contains translation results and other related information. We can use the JSON library to parse this string and extract the translation results we need:
import com.alibaba.fastjson.JSON; import com.alibaba.fastjson.JSONArray; import com.alibaba.fastjson.JSONObject; public class TranslationAPI { // ... private static String parseTranslationResult(String response) { JSONObject jsonResult = JSON.parseObject(response); JSONArray translationArray = jsonResult.getJSONArray("trans_result"); StringBuilder translationResult = new StringBuilder(); for (int i = 0; i < translationArray.size(); i++) { JSONObject translationItem = translationArray.getJSONObject(i); String translatedText = translationItem.getString("dst"); translationResult.append(translatedText).append(" "); } return translationResult.toString(); } }
- Run the code
Using the Java code written in the above steps, we will Can realize mutual translation between Chinese and Indonesian. Call the translation API in the main method and output the result:
public class TranslationAPI { // ... public static void main(String[] args) { String sourceText = "中文文本"; // 替换为你的中文文本 // ... String translationResult = parseTranslationResult(response.toString()); System.out.println("翻译结果:" + translationResult); } }
The above is a practical case of using Java to realize mutual translation between Chinese and Indonesian through Baidu Translation API. Through this case, we can learn how to call the API to send requests, parse API responses and obtain translation results. I hope this article will help you learn Java and implement multi-language translation!
The above is the detailed content of Practical case of mutual translation between Chinese and Indonesian using Java Baidu Translation API. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor
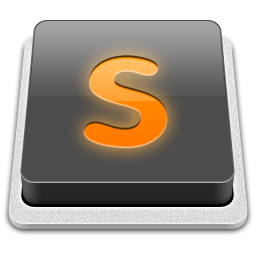
SublimeText3 Mac version
God-level code editing software (SublimeText3)