


Exploration and practice of optimization strategies for Go language website access speed
Go language, as a high-performance, high-concurrency programming language, has become more and more popular among developers in recent years. However, for Go language websites, optimization of access speed is still an important issue. This article will explore and practice some optimization strategies to help us improve the access speed of Go language websites.
1. Reduce HTTP requests
HTTP requests are one of the main causes of performance bottlenecks in web applications. Reducing HTTP requests can effectively improve the response speed of the website. We can reduce the number of HTTP requests by merging and compressing CSS and JavaScript files. The following is a sample code:
package main import ( "bytes" "compress/gzip" "encoding/base64" "fmt" "io/ioutil" "net/http" ) func main() { http.HandleFunc("/combine", combineHandler) http.ListenAndServe(":8080", nil) } func combineHandler(w http.ResponseWriter, r *http.Request) { var combinedFile bytes.Buffer // 读取CSS文件 cssContent, err := ioutil.ReadFile("style.css") if err != nil { http.Error(w, "Error reading CSS file", http.StatusInternalServerError) return } // 读取JavaScript文件 jsContent, err := ioutil.ReadFile("script.js") if err != nil { http.Error(w, "Error reading JavaScript file", http.StatusInternalServerError) return } // 合并CSS和JavaScript文件 combinedFile.Write(cssContent) combinedFile.Write(jsContent) // Gzip压缩合并后的文件 gzipBuffer := bytes.Buffer{} gzipWriter := gzip.NewWriter(&gzipBuffer) gzipWriter.Write(combinedFile.Bytes()) gzipWriter.Close() // 将压缩后的文件进行Base64编码 encodedContent := base64.StdEncoding.EncodeToString(gzipBuffer.Bytes()) // 返回合并、压缩和编码后的文件给客户端 w.Header().Set("Content-Encoding", "gzip") fmt.Fprintf(w, "<script src="data:text/javascript;base64,%s"></script>", encodedContent) }
2. Use caching
Let browsers and proxy servers cache static resources is another effective strategy to speed up the website. We can inform the browser and proxy server of the validity period of the resource by setting the Cache-Control and Expires fields of the response header. The following is a sample code:
package main import ( "net/http" "time" ) func main() { http.HandleFunc("/", imageHandler) http.ListenAndServe(":8080", nil) } func imageHandler(w http.ResponseWriter, r *http.Request) { http.ServeFile(w, r, "image.jpg") // 设置响应头的Cache-Control和Expires字段 w.Header().Set("Cache-Control", "public, max-age=86400") w.Header().Set("Expires", time.Now().Add(time.Hour*24).Format(http.TimeFormat)) }
3. Concurrent processing of requests
The Go language inherently supports concurrency and can easily implement concurrent processing of requests, thereby improving the throughput and response speed of the website. The following is a sample code:
package main import ( "fmt" "net/http" "sync" "time" ) func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) } func handler(w http.ResponseWriter, r *http.Request) { var wg sync.WaitGroup for i := 0; i < 10; i++ { wg.Add(1) go func() { defer wg.Done() // 执行一些耗时操作 time.Sleep(time.Second) fmt.Fprintln(w, "Hello, World!") }() } wg.Wait() }
Through the above optimization strategy, we can significantly improve the access speed of the Go language website. Of course, depending on the specific application scenario, we can also adopt other strategies, such as using CDN acceleration, using lightweight frameworks, etc. The most important thing is that we need to constantly try and optimize according to the actual situation in order to achieve the best performance and user experience.
The above is the detailed content of Exploration and practice of optimization strategies for Go language website access speed. For more information, please follow other related articles on the PHP Chinese website!
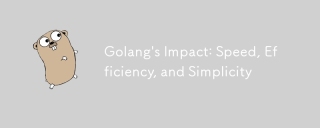
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
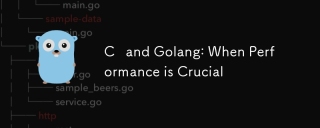
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
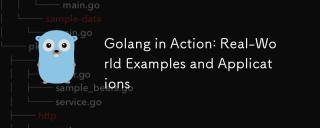
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
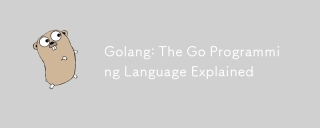
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
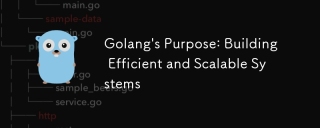
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
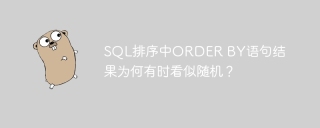
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
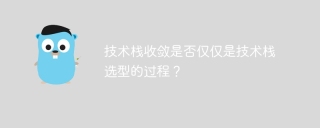
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
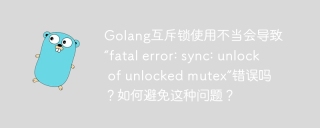
Golang ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
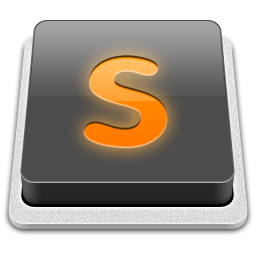
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
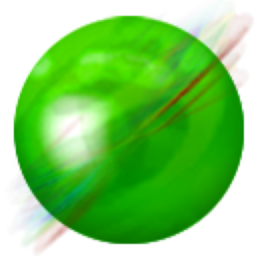
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment