


How to perform identity authentication and authorization for Java function development
How to perform identity authentication and authorization for Java function development
In the modern Internet era, identity authentication and authorization are a very important part of software development. Whether it is a website, mobile application or other type of software, the user's identity needs to be authenticated to ensure that only legitimate users can access and use relevant functions. This article will introduce how to use Java to develop identity authentication and authorization functions, and attach code examples.
1. Identity Authentication
Identity authentication is the process of verifying the user's identity to ensure that the identity credentials (such as user name and password) provided by the user are correct. Common identity authentication methods include basic authentication, form authentication, and third-party authentication.
- Basic Authentication
Basic authentication is the simplest form of identity authentication. It base64 encodes the user's account and password and authenticates them stored on the server. Information is compared. The following is an example of using basic authentication:
import java.io.IOException; import java.nio.charset.StandardCharsets; import java.util.Base64; public class BasicAuthenticationExample { public boolean authenticate(String username, String password) { // 模拟从服务器端获取用户存储的账号和密码 String storedUsername = "admin"; String storedPassword = "password"; // 对用户提供的账号和密码进行Base64编码 String encodedUsername = Base64.getEncoder().encodeToString(username.getBytes(StandardCharsets.UTF_8)); String encodedPassword = Base64.getEncoder().encodeToString(password.getBytes(StandardCharsets.UTF_8)); // 比对用户提供的账号和密码与服务器端存储的认证信息 return encodedUsername.equals(Base64.getEncoder().encodeToString(storedUsername.getBytes(StandardCharsets.UTF_8))) && encodedPassword.equals(Base64.getEncoder().encodeToString(storedPassword.getBytes(StandardCharsets.UTF_8))); } public static void main(String[] args) throws IOException { BasicAuthenticationExample example = new BasicAuthenticationExample(); // 模拟用户提供的账号和密码 String username = "admin"; String password = "password"; boolean authenticated = example.authenticate(username, password); System.out.println("身份认证结果:" + authenticated); } }
- Form authentication
Form authentication means that after the user enters the account and password on the login page, the authentication information is entered in the form. The form is submitted to the server for validation. The following is an example of using form authentication:
import java.io.IOException; public class FormAuthenticationExample { public boolean authenticate(String username, String password) { // 模拟从服务器端获取用户存储的账号和密码 String storedUsername = "admin"; String storedPassword = "password"; // 比对用户提供的账号和密码与服务器端存储的认证信息 return username.equals(storedUsername) && password.equals(storedPassword); } public static void main(String[] args) throws IOException { FormAuthenticationExample example = new FormAuthenticationExample(); // 模拟用户提供的账号和密码 String username = "admin"; String password = "password"; boolean authenticated = example.authenticate(username, password); System.out.println("身份认证结果:" + authenticated); } }
- Third-party authentication
Third-party authentication refers to using a third-party platform (such as Google, Facebook, etc.) to verify users identity. Normally, users choose to use a third-party platform to log in in the application, and then pass the obtained authorization information to the server for verification. The following is an example of using Google third-party authentication:
(see official documentation for sample code)
2. Identity authorization
Identity authorization is performed on authenticated users The process of permission management controls the user's access to specific functions based on the user's identity and operation permissions. Common identity authorization methods include role authorization, resource-based authorization and RBAC model.
- Role Authorization
Role authorization refers to assigning users to different roles, and each role has different permissions. The following is an example of using role authorization:
(see official documentation for sample code)
- Resource-based authorization
Resource-based authorization refers to Control based on user access to specific resources. The following is an example of using resource-based authorization:
(see official documentation for sample code)
- RBAC model
RBAC (Role-Based Access Control) model is a common identity authorization model that clearly defines and manages the relationship between users, roles, and permissions. The following is an example of using the RBAC model for identity authorization:
(See official documentation for sample code)
Identity authentication and authorization are an essential part of software development. Through the introduction of this article, I believe readers can understand how to use Java to develop identity authentication and authorization functions, and be able to choose appropriate identity authentication and authorization methods according to specific needs. Code examples can help readers better understand and practice related functional development.
The above is the detailed content of How to perform identity authentication and authorization for Java function development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
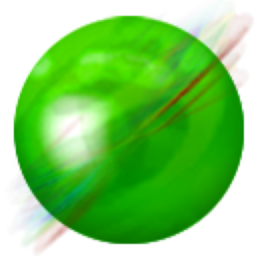
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
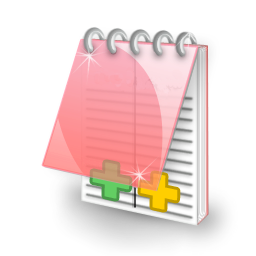
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
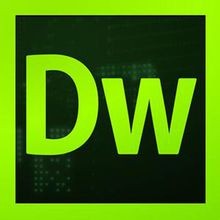
Dreamweaver CS6
Visual web development tools