


In-depth study of the coroutine scheduling mechanism of Swoole development functions
Introduction:
In recent years, the PHP language has greatly improved in terms of performance and concurrent processing capabilities. At the same time, Swoole, as a high-performance PHP extension, provides developers with powerful functions and tools. Among them, the coroutine scheduling mechanism is one of the important features of Swoole. This article will conduct an in-depth study of the coroutine scheduling mechanism of Swoole development functions, and illustrate its usage and effects through code examples.
1. What is the coroutine scheduling mechanism?
Coroutine is a more lightweight concurrent processing method than threads. The traditional multi-thread concurrency model requires thread switching and resource scheduling through the operating system's scheduler, which will bring significant overhead. Coroutines are lightweight threads implemented at the user level that can switch between different tasks without relying on operating system scheduling.
Swoole's coroutine scheduling mechanism allows developers to use coroutines in PHP to implement asynchronous programming and improve the performance and efficiency of concurrent processing. By using the coroutine API provided by Swoole, we can easily create and manage coroutines and implement concurrent processing and scheduling of tasks.
2. The usage and effects of coroutines
The following uses a code example to illustrate the usage and effects of coroutines. Suppose we need to send multiple HTTP requests concurrently and wait for all requests to return results before proceeding to the next step.
<?php use SwooleCoroutineHttpClient; // 创建一个协程 go(function () { // 创建多个HTTP协程客户端 $urls = [ 'https://www.example.com/', 'https://www.google.com/', 'https://www.baidu.com/' ]; $results = []; foreach ($urls as $url) { go(function () use ($url, &$results) { // 创建一个HTTP客户端 $client = new Client($url); // 发送GET请求并接收响应 $client->get('/'); $response = $client->getBody(); // 存储请求结果 $results[$url] = $response; // 关闭HTTP客户端连接 $client->close(); }); } // 等待所有协程执行完毕 while (count($results) < count($urls)) { SwooleCoroutine::sched_yield(); } // 打印请求结果 foreach ($results as $url => $response) { echo "URL: {$url}, Response: {$response} "; } }); // 启动Swoole事件循环 SwooleEvent::wait();
In the above code, we use the go keyword to create a coroutine. In the coroutine, we create multiple HTTP coroutine clients, send GET requests, and store the response results in the $results array. Then, we wait for all coroutines to finish executing by using a while loop and the SwooleCoroutine::sched_yield() function. Finally, we traverse the $results array and output the request results.
Through the coroutine scheduling mechanism, we can process multiple time-consuming IO tasks concurrently to improve overall processing performance and efficiency. Moreover, coroutine switching and scheduling are implemented at the user level, which has less overhead than traditional thread switching.
3. Further applications of coroutines
In addition to processing concurrent HTTP requests, the coroutine scheduling mechanism can also be used in other scenarios, such as database connection pools, task queues, scheduled tasks, etc. In these scenarios, we can use the coroutine component provided by Swoole, combined with the coroutine scheduling mechanism, to achieve high performance and efficient concurrent processing.
4. Conclusion
Swoole's coroutine scheduling mechanism is a powerful function that can greatly improve the performance and concurrent processing capabilities of PHP programs. By using coroutines, we can easily implement asynchronous programming and concurrent processing, improving the throughput and response speed of the system.
In actual development, we should make full use of the coroutine API provided by Swoole and combine it with the coroutine scheduling mechanism to optimize the efficiency and performance of concurrent processing. Of course, when using coroutines, you also need to pay attention to the switching and scheduling overhead of coroutines, and avoid excessive creation and switching of coroutines to avoid negative impacts on system performance.
I hope this article will help you understand and apply Swoole's coroutine scheduling mechanism, thank you for reading!
Reference link:
- Swoole official documentation: https://www.swoole.com/
- Swoole GitHub repository: https://github.com/ swoole/swoole-src
The above is the detailed content of In-depth study of the coroutine scheduling mechanism of swoole development function. For more information, please follow other related articles on the PHP Chinese website!
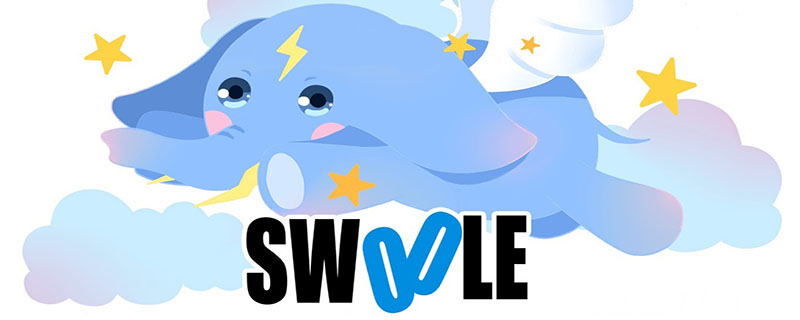
怎么在docker中搭建swoole环境?下面本篇文章给大家介绍一下用docker搭建swoole环境的方法,希望对大家有所帮助!
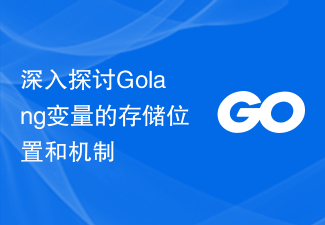
标题:深入探讨Golang变量的存储位置和机制随着Go语言(Golang)在云计算、大数据和人工智能领域的应用逐渐增多,深入了解Golang变量的存储位置和机制变得尤为重要。在本文中,我们将详细探讨Golang中变量的内存分配、存储位置以及相关的机制。通过具体代码示例,帮助读者更好地理解Golang变量在内存中是如何存储和管理的。1.Golang变量的内存
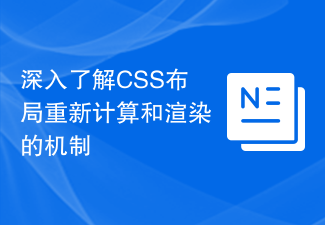
CSS回流(reflow)和重绘(repaint)是网页性能优化中非常重要的概念。在开发网页时,了解这两个概念的工作原理,可以帮助我们提高网页的响应速度和用户体验。本文将深入探讨CSS回流和重绘的机制,并提供具体的代码示例。一、CSS回流(reflow)是什么?当DOM结构中的元素发生可视性、尺寸或位置改变时,浏览器需要重新计算并应用CSS样式,然后重新布局
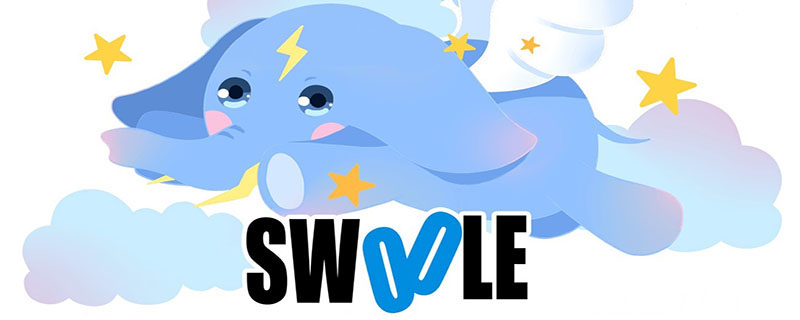
为什么要在 Swoole 上运行 Laravel?因为使用 Swoole 可以加速 Laravel 应用。下面本篇文章就来带大家聊聊怎么在Swoole上使用Laravel,希望对大家有所帮助!
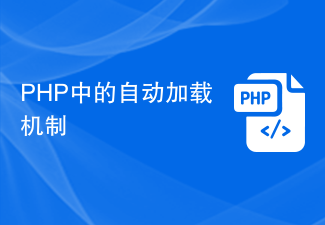
随着PHP语言越来越受欢迎,开发人员需要使用越来越多的类和函数。当项目规模扩大时,手动引入所有依赖项将变得不切实际。这时候就需要一种自动加载机制来简化代码开发和维护过程。自动加载机制是一种PHP语言的特性,可以在运行时自动载入所需的类和接口,并减少手动的类文件引入。这样,程序员可以专注于开发代码,减少因繁琐的手动类引入而产生的错误和时间浪费。在PHP中,一般
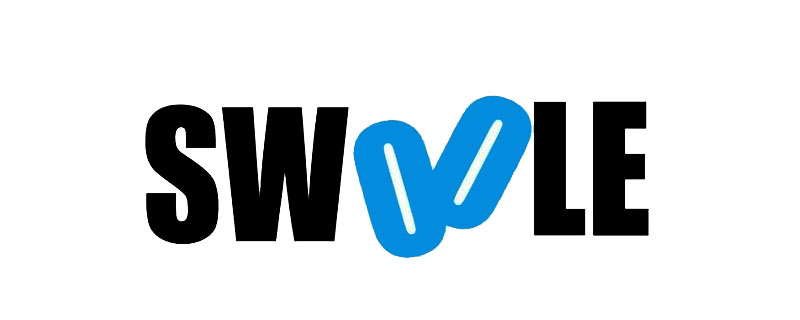
swoole采用的架构模式:多线程Reactor+多进程Worker,因为reactor是基于epoll的,所以不难看出每个reactor,它可以用来处理无数个连接请求。 如此,swoole就轻松的实现了高并发的处理。
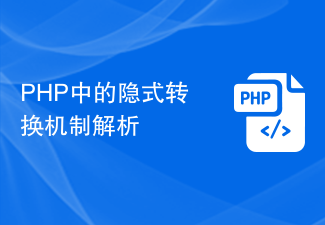
PHP中的隐式转换机制解析在PHP编程中,隐式转换是指在不显式指定类型转换的情况下,PHP自动将一个数据类型转换为另一个数据类型的过程。隐式转换机制在编程中非常常见,但也容易造成一些意想不到的bug,因此了解隐式转换机制的原理和规则对于编写稳健的PHP代码非常重要。1.整型与浮点型之间的隐式转换在PHP中,整型和浮点型之间的隐式转换是非常常见的。当一个整型
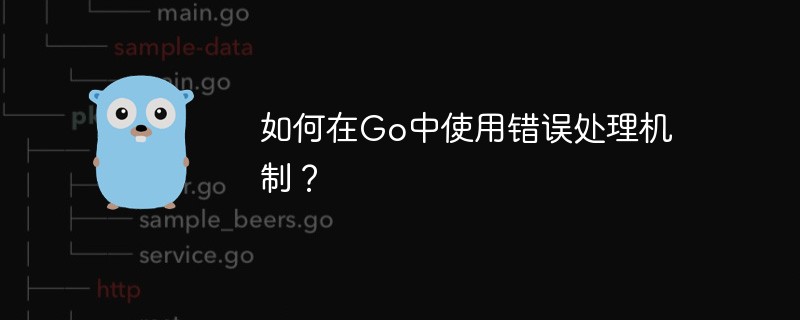
Go语言作为一门强类型、高效、现代化的编程语言,在现代软件开发中得到了越来越广泛的应用。其中,错误处理机制是Go语言值得关注的一个方面,而Go语言的错误处理机制相比于其他编程语言也有很大的不同和优越性。本文将介绍Go语言的错误处理机制的基本概念、实现方式以及最佳实践,以帮助读者更好地理解和使用Go语言中的错误处理机制。一、Go语言错误处理机制的基本概念在Go


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
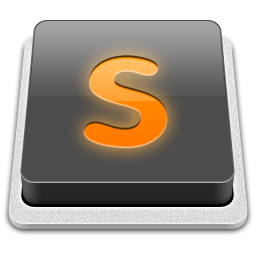
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor
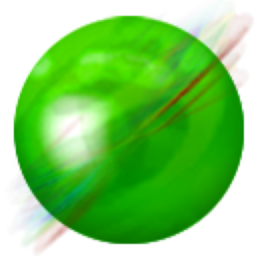
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
