How to use Go language for code modularization practice
How to use Go language for code modularization practice
Introduction:
In software development, code modularization is a common development methodology. By dividing the code into reusable modules, you can Improve code maintainability, testability and reusability. This article will introduce how to use Go language to practice code modularization and provide corresponding code examples.
1. Advantages of modularization
- Improve code maintainability: Modularization divides the code into independent functional modules, each module is responsible for specific tasks, making the code clearer and easy to modify.
- Improve code testability: Independent modules can make unit testing easier, reducing testing difficulty and workload.
- Improve code reusability: Modularized code can be easily referenced and reused by other projects.
2. Code modularization in Go language
The Go language itself supports modular development and provides some key mechanisms to achieve code reusability and organizational structure.
- Package (package)
The package in Go language is the basic unit of code modularization. A package consists of a set of related Go source files that together provide a related set of functionality. Each package has an individual name that can be referenced in other code.
The following is the directory structure and code example of a package:
└── mypackage ├── main.go ├── module1.go └── module2.go
In the module1.go
file, a file named Module1# is defined ##'s structure and an externally accessible method
Module1Func:
package mypackage type Module1 struct { // ... } func (m *Module1) Module1Func() { // ... }In the
module2.go file, a file named
Module2# is defined ##'s structure and an externally accessible method Module2Func
: <pre class='brush:php;toolbar:false;'>package mypackage
type Module2 struct {
// ...
}
func (m *Module2) Module2Func() {
// ...
}</pre>
In the
file, you can reference and use mypackage
Modules in the package: <pre class='brush:php;toolbar:false;'>package main
import (
"fmt"
"mypackage"
)
func main() {
module1 := &mypackage.Module1{}
module1.Module1Func()
module2 := &mypackage.Module2{}
module2.Module2Func()
}</pre>
- In the Go language, naming conventions are used to determine whether identifiers (variables, functions, structures, etc.) in the package can be External code access.
In the above example,
Module1 and Module2
are externally visible identifiers that can be referenced and used in other code. The Module1Func
and Module2Func
are private and can only be used inside the mypackage
package. 3. Modularization practice example
Suppose we need to develop a calculator program that contains two functional modules: addition and subtraction.
- First, create a package directory named
- calculator
, and createaddition.go
andsubtraction.go
Two source files. Writing the addition module - In the
addition.go
file, define a structureAddition
and an externally accessible structure used to implement the addition function Addition methodAdd
:<pre class='brush:php;toolbar:false;'>package calculator type Addition struct { // ... } func (a *Addition) Add(x, y int) int { return x + y }</pre>
Writing subtraction module - In the
subtraction.go
file, define a subtraction function The structureSubtraction
and an externally accessible subtraction methodSubtract
:<pre class='brush:php;toolbar:false;'>package calculator type Subtraction struct { // ... } func (s *Subtraction) Subtract(x, y int) int { return x - y }</pre>
Refer to and use the module in the main program - In
main.go
, you can reference and use the module in thecalculator
package:<pre class='brush:php;toolbar:false;'>package main import ( "calculator" "fmt" ) func main() { adder := &calculator.Addition{} result := adder.Add(5, 3) fmt.Println("Addition:", result) subtracter := &calculator.Subtraction{} result = subtracter.Subtract(5, 3) fmt.Println("Subtraction:", result) }</pre>
Run the above example code, the output will be as follows Result:
Addition: 8 Subtraction: 2
Conclusion:
Through the package and visibility mechanism of the Go language, we can easily modularize the code and encapsulate and share data and functions between multiple functional modules. This helps improve code maintainability, testability, and reusability. At the same time, reasonable module division can make the code clearer and easier to read. I believe that by studying the content and examples of this article, you will be able to better use the Go language to practice code modularization.The above is the detailed content of How to use Go language for code modularization practice. For more information, please follow other related articles on the PHP Chinese website!
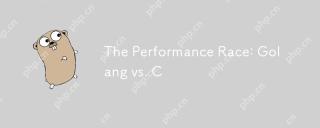
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
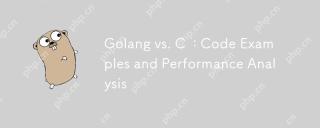
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
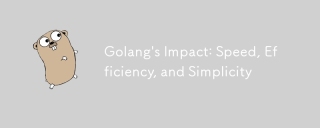
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
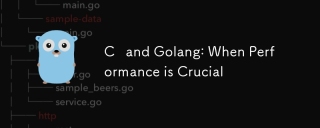
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
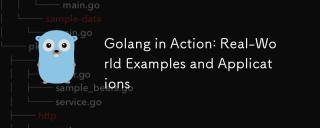
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
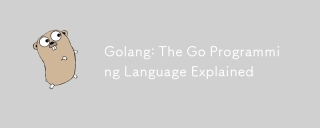
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
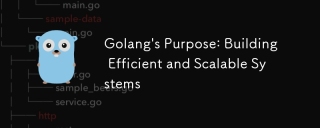
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
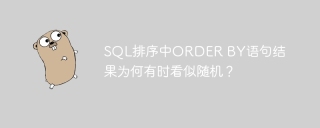
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor