How to use Go language for message queue processing
How to use Go language for message queue processing
Message queue is a commonly used information transmission and processing method, used to achieve asynchronous communication and decoupling between systems. As a high-performance, concise programming language, the Go language also provides good support for message queue processing. This article will introduce how to use Go language for message queue processing and provide corresponding code examples.
First of all, we need to choose a suitable message queue system. Currently commonly used message queue systems include RabbitMQ, Kafka, NSQ, etc., each of which has its own characteristics and applicable scenarios. When choosing, we need to take into account the actual needs of the system and the expected performance.
Assuming we choose RabbitMQ as the message queue system, next we need to install RabbitMQ and the corresponding Go language client library. To install RabbitMQ, you can refer to the official documentation. To install the Go language client library, you can use the go get command:
go get github.com/streadway/amqp
After the installation is complete, we can start writing code to implement message queue processing. First, we need to establish a connection with RabbitMQ. The code example is as follows:
package main import ( "log" "github.com/streadway/amqp" ) func main() { conn, err := amqp.Dial("amqp://guest:guest@localhost:5672/") if err != nil { log.Fatalf("Failed to connect to RabbitMQ: %s", err) } defer conn.Close() // TODO: 进一步处理消息队列 }
After establishing the connection, we can create a channel (Channel) for sending and receiving messages. The code example is as follows:
channel, err := conn.Channel() if err != nil { log.Fatalf("Failed to open a channel: %s", err) } defer channel.Close()
Next, we can create a message queue and set the corresponding properties. The sample code is as follows:
queue, err := channel.QueueDeclare( "my_queue", // 队列名称 false, // 是否持久化 false, // 是否具有排他性 false, // 是否自动删除 false, // 是否优先级队列 nil, // 其他属性 ) if err != nil { log.Fatalf("Failed to declare a queue: %s", err) }
After creating the queue, we can use the channel.Publish method to send messages to the queue. The sample code is as follows:
body := []byte("Hello, RabbitMQ!") err = channel.Publish( "", // 目标交换机名称 queue.Name, // 目标队列名称 false, // 是否等待交换机确认 false, // 是否等待结果返回 amqp.Publishing{ ContentType: "text/plain", Body: body, }, ) if err != nil { log.Fatalf("Failed to publish a message: %s", err) }
The process of receiving messages is also very simple. We can use the channel.Consume method to set a callback function to process the received messages. The sample code is as follows:
msgs, err := channel.Consume( queue.Name, // 队列名称 "", // 消费者名称,为空代表自动生成 true, // 是否自动确认 false, // 是否独占消费者 false, // 是否阻塞等待 false, // 额外的属性 ) if err != nil { log.Fatalf("Failed to register a consumer: %s", err) } go func() { for msg := range msgs { log.Printf("Received a message: %s", msg.Body) } }()
The above is the basic process and code example of using Go language for message queue processing. Through the concise and efficient Go language and powerful message queue system, we can achieve flexible and reliable communication and decoupling between systems.
It should be noted that in actual applications, we also need to handle abnormal situations, ensure the reliability and efficiency of messages, and perform performance optimization and monitoring. However, the sample code provided in this article has covered the basic functions and usage, and can be used as a starting point for learning and practice.
References:
- Go language official documentation: https://golang.org/
- RabbitMQ official documentation: https://www.rabbitmq.com /documentation.html
- RabbitMQ Go client library documentation: https://godoc.org/github.com/streadway/amqp
The above is the detailed content of How to use Go language for message queue processing. For more information, please follow other related articles on the PHP Chinese website!
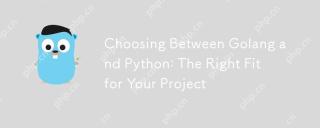
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
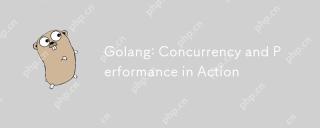
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
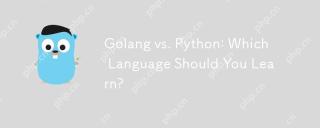
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
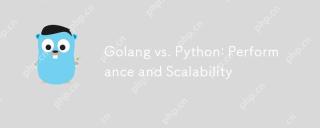
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
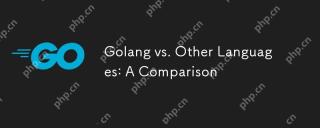
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
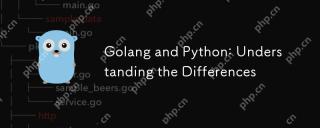
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
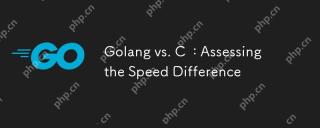
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
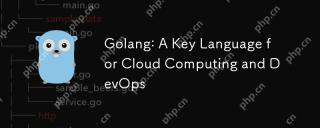
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
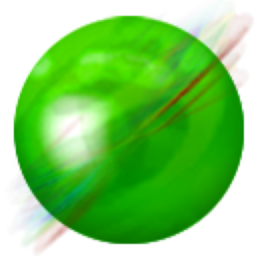
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
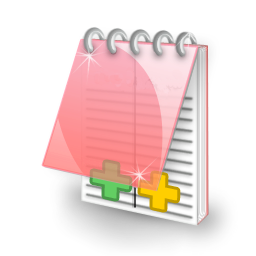
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
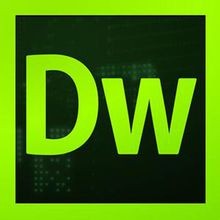
Dreamweaver CS6
Visual web development tools