


Implement complex user interfaces using the new JavaFX layout components in Java 13
Use the new JavaFX layout components in Java 13 to implement complex user interfaces
In software development, the user interface is a very important part. A good user interface can improve the user experience and increase the ease of use and attractiveness of the software. JavaFX is a Java library for building rich interactive applications. It provides a set of powerful layout components that can help developers implement complex user interfaces.
JavaFX has become part of Java 13, which includes a number of new layout components that make it easier to build complex user interfaces.
In this article, we will introduce two new layout components in JavaFX 13: FlowPane and GridPane, and demonstrate how to use them to implement complex user interfaces.
First, let’s learn about FlowPane. FlowPane is an autolayout component that automatically adjusts the size and position of child components to fit the size of the container.
The following is a simple example using FlowPane:
import javafx.application.Application; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.FlowPane; import javafx.stage.Stage; public class FlowPaneExample extends Application { @Override public void start(Stage primaryStage) { FlowPane flowPane = new FlowPane(); flowPane.setPadding(new Insets(20)); flowPane.setVgap(10); flowPane.setHgap(10); Button button1 = new Button("Button 1"); Button button2 = new Button("Button 2"); Button button3 = new Button("Button 3"); flowPane.getChildren().addAll(button1, button2, button3); Scene scene = new Scene(flowPane, 400, 300); primaryStage.setScene(scene); primaryStage.setTitle("FlowPane Example"); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
The above example is a simple application that uses FlowPane to lay out three buttons. FlowPane automatically adjusts the button's position and size based on the size of the container.
Next, let us introduce another new layout component in JavaFX 13: GridPane. GridPane is a table layout component that places child components in different cells in the grid.
The following is a simple example of using GridPane:
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.ColumnConstraints; import javafx.scene.layout.GridPane; import javafx.scene.layout.RowConstraints; import javafx.stage.Stage; public class GridPaneExample extends Application { @Override public void start(Stage primaryStage) { GridPane gridPane = new GridPane(); gridPane.setHgap(10); gridPane.setVgap(10); ColumnConstraints column1 = new ColumnConstraints(100); ColumnConstraints column2 = new ColumnConstraints(100); RowConstraints row1 = new RowConstraints(50); RowConstraints row2 = new RowConstraints(50); gridPane.getColumnConstraints().addAll(column1, column2); gridPane.getRowConstraints().addAll(row1, row2); Button button1 = new Button("Button 1"); Button button2 = new Button("Button 2"); Button button3 = new Button("Button 3"); Button button4 = new Button("Button 4"); gridPane.add(button1, 0, 0); gridPane.add(button2, 1, 0); gridPane.add(button3, 0, 1); gridPane.add(button4, 1, 1); Scene scene = new Scene(gridPane, 400, 300); primaryStage.setScene(scene); primaryStage.setTitle("GridPane Example"); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
The above example is a simple application that uses GridPane to lay out four buttons. The GridPane automatically places buttons at the appropriate location and size, and the grid can be resized by setting constraints on columns and rows.
The above demonstrates two new layout components in JavaFX 13, which can help developers implement complex user interfaces. These layout components provide more flexibility and power, making it easier to build complex user interfaces.
I hope the examples in this article can help you understand how to use JavaFX layout components to implement complex user interfaces. Get started and design an attractive user interface!
The above is the detailed content of Implement complex user interfaces using the new JavaFX layout components in Java 13. For more information, please follow other related articles on the PHP Chinese website!
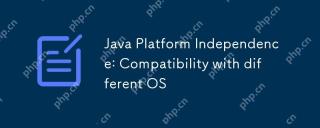
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
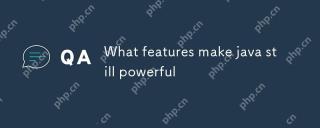
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
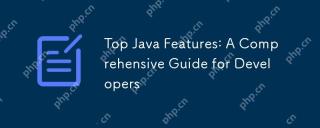
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.
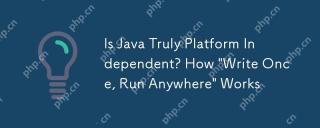
JavaisnotentirelyplatformindependentduetoJVMvariationsandnativecodeintegration,butitlargelyupholdsitsWORApromise.1)JavacompilestobytecoderunbytheJVM,allowingcross-platformexecution.2)However,eachplatformrequiresaspecificJVM,anddifferencesinJVMimpleme
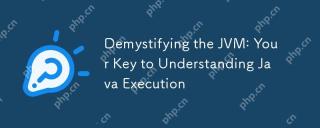
TheJavaVirtualMachine(JVM)isanabstractcomputingmachinecrucialforJavaexecutionasitrunsJavabytecode,enablingthe"writeonce,runanywhere"capability.TheJVM'skeycomponentsinclude:1)ClassLoader,whichloads,links,andinitializesclasses;2)RuntimeDataAr
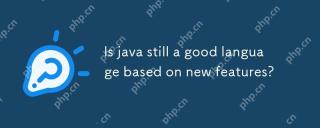
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
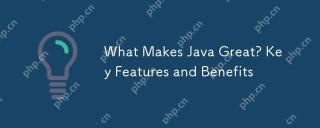
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
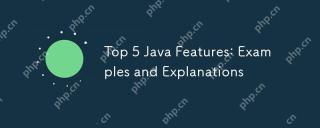
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
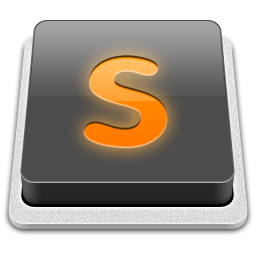
SublimeText3 Mac version
God-level code editing software (SublimeText3)
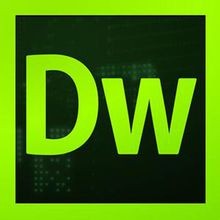
Dreamweaver CS6
Visual web development tools
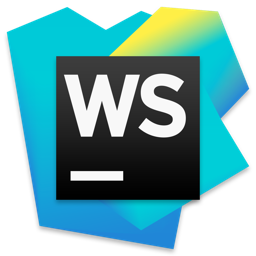
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
