How to achieve thread synchronization using lock mechanism in Java?
How to use the lock mechanism in Java to achieve thread synchronization?
In multi-threaded programming, thread synchronization is a very important concept. When multiple threads access and modify shared resources at the same time, data inconsistency or race conditions may result. Java provides a locking mechanism to solve these problems and ensure thread-safe access to shared resources.
The locking mechanism in Java is provided by the synchronized keyword and the Lock interface. Next, we'll learn how to use these two mechanisms to achieve thread synchronization.
Example of using the synchronized keyword to implement thread synchronization:
class Counter { private int count = 0; public synchronized void increment() { count++; } public synchronized int getCount() { return count; } } class IncrementThread extends Thread { private Counter counter; public IncrementThread(Counter counter) { this.counter = counter; } public void run() { for (int i = 0; i < 1000; i++) { counter.increment(); } } } public class SynchronizedExample { public static void main(String[] args) throws InterruptedException { Counter counter = new Counter(); IncrementThread thread1 = new IncrementThread(counter); IncrementThread thread2 = new IncrementThread(counter); thread1.start(); thread2.start(); thread1.join(); thread2.join(); System.out.println("Final count: " + counter.getCount()); } }
In the above example, the Counter class has a count variable used to represent the value of the counter. The increment() method is modified with the synchronized keyword, which means that only one thread can access and modify the count variable at any time. The getCount() method is also modified with the synchronized keyword to ensure thread safety when obtaining the counter value.
The IncrementThread class is a thread class that accepts a Counter object as a constructor parameter and calls the increment() method in the run() method to increase the value of the counter.
In the main program, we create two IncrementThread threads and pass them to two thread instances respectively. We then start these two threads and wait for them to complete using the join() method. Finally, we print out the final counter value.
Example of using the Lock interface to implement thread synchronization:
class Counter { private int count = 0; private Lock lock = new ReentrantLock(); public void increment() { lock.lock(); try { count++; } finally { lock.unlock(); } } public int getCount() { lock.lock(); try { return count; } finally { lock.unlock(); } } } class IncrementThread extends Thread { private Counter counter; public IncrementThread(Counter counter) { this.counter = counter; } public void run() { for (int i = 0; i < 1000; i++) { counter.increment(); } } } public class LockExample { public static void main(String[] args) throws InterruptedException { Counter counter = new Counter(); IncrementThread thread1 = new IncrementThread(counter); IncrementThread thread2 = new IncrementThread(counter); thread1.start(); thread2.start(); thread1.join(); thread2.join(); System.out.println("Final count: " + counter.getCount()); } }
In the above example, the Lock interface is used in the increment() and getCount() methods of the Counter class to implement thread synchronization. We create a ReentrantLock instance to acquire and release the lock at the beginning and end of the method respectively.
The code for the IncrementThread class and the main program is the same as in the previous example. Just use the Lock interface instead of the synchronized keyword in the Counter class to achieve thread synchronization.
Summary:
In multi-threaded programming, thread synchronization is an important concept. Java provides the synchronized keyword and Lock interface to achieve thread synchronization. No matter which mechanism is used, it can be guaranteed that only one thread can access and modify shared resources at any time, thereby ensuring thread-safe access.
The above is the sample code for using the lock mechanism in Java to achieve thread synchronization. By understanding and studying these examples, we can better apply thread synchronization to ensure the correctness and performance of multi-threaded programs.
The above is the detailed content of How to achieve thread synchronization using lock mechanism in Java?. For more information, please follow other related articles on the PHP Chinese website!
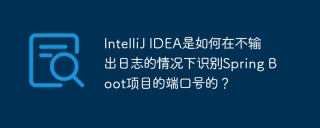
Start Spring using IntelliJIDEAUltimate version...
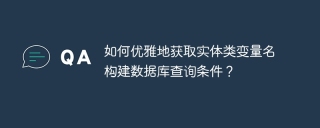
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
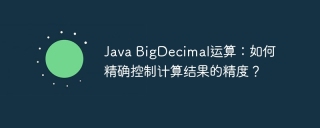
Java...
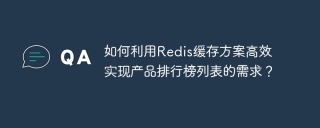
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
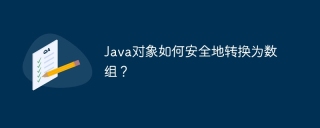
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
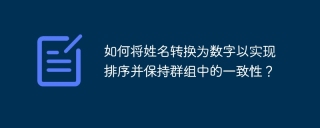
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
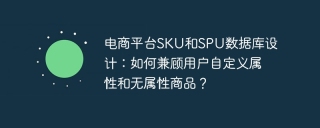
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
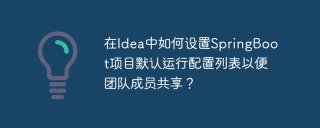
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
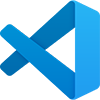
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software