How to use Go language for code portability assessment
How to use Go language to evaluate code portability
Introduction:
With the development of software development, code portability has gradually become an important issue that program developers are concerned about. In the process of software development, in order to improve efficiency, reduce costs, and cope with multi-platform requirements, we often need to migrate code to different target environments. For Go language developers, some features of the Go language make it an ideal choice because the Go language has excellent portability and scalability. This article will introduce how to use Go language to conduct code portability assessment, and attach some code examples.
1. What is code portability?
Code portability refers to the ability to move code from one platform to another. This often involves resolving differences in different operating systems, hardware, or software environments. Code portability assessment is the process of evaluating whether code can run successfully on different platforms.
2. Methods for evaluating code portability
- Compilation test
Compilation test is one of the most basic code portability evaluation methods. In the Go language, we can test how the code compiles on different platforms by using different compilers and compilation parameters. For example, we can use thego build
command to compile the code and observe whether there are errors or warning messages. If errors or warnings appear during compilation, the code may be incompatible with the target environment.
Example:
package main import "fmt" func main() { fmt.Println("Hello, World!") }
Run the go build
command in the command line to compile:
go build main.go
If the compilation is successful, a programmable file will be generated. executable file. If an error occurs during compilation, an error message will be displayed.
- Test Cases
Writing test cases can help us evaluate the portability of the code. By writing a series of test cases, we can simulate different environments and check how the code runs in different environments. If the test case can pass and produce the same results on different platforms, it means that the code has good portability.
Example:
package main import "testing" func TestAdd(t *testing.T) { result := Add(2, 3) if result != 5 { t.Errorf("Add(2, 3) = %d; want 5", result) } } func Add(a, b int) int { return a + b }
Run the go test
command in the command line to test:
go test -v
If the test passes, the test case will be displayed execution results. If the test fails, an error message will be displayed.
3. Code Example
The following is a simple example showing how to use Go language to evaluate code portability.
package main import "fmt" func main() { if IsWindows() { fmt.Println("This code is running on Windows.") } else if IsLinux() { fmt.Println("This code is running on Linux.") } else { fmt.Println("This code is running on an unknown platform.") } } func IsWindows() bool { // 判断是否为Windows平台的代码 } func IsLinux() bool { // 判断是否为Linux平台的代码 }
By writing IsWindows
and IsLinux
functions for different platforms, we can judge the current running environment of the code based on the characteristics of different platforms. For example, we can use runtime.GOOS
in the IsWindows
function to determine whether it is a Windows platform. Similarly, we can use runtime in the
IsLinux function. GOOS
to determine whether it is a Linux platform. In this way, we can execute different code logic according to specific platform conditions.
Conclusion:
Through the above introduction, we can understand that using Go language to evaluate the portability of code is a relatively simple but very important task. By compiling tests and test cases, we can evaluate how the code runs on different platforms and identify potential compatibility issues. In actual development, we should try our best to design and implement code with good portability so that code migration can be carried out quickly and stably.
The above is the detailed content of How to use Go language for code portability assessment. For more information, please follow other related articles on the PHP Chinese website!
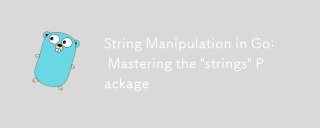
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
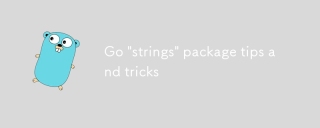
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
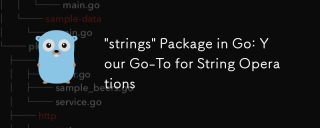
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
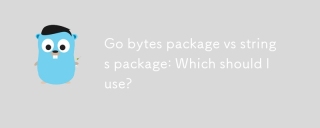
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
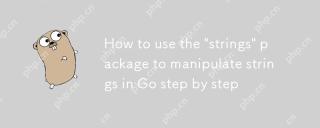
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
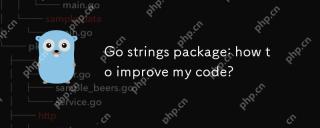
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
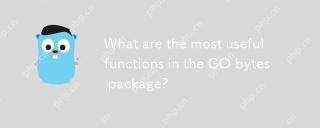
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
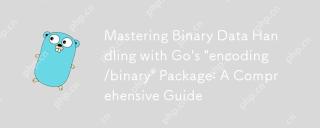
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
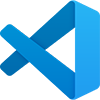
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
