


How to use PHP extensions to enhance the functionality of your application
How to use PHP extensions to enhance the functionality of your application
Abstract: PHP is a scripting language widely used in website development. Although PHP itself provides many useful features, sometimes we may need more powerful features to meet specific needs. In this article, we will explore how to use PHP extensions to enhance the functionality of your application and provide some code examples.
- What is a PHP extension?
PHP extension is a dynamic link library written in C that can be added to PHP's function library by enabling it in the PHP configuration file or compiling and installing it. PHP extensions can provide many new features and performance optimizations to applications, and these features can be used through PHP function calls. -
Installing and enabling PHP extensions
To use a PHP extension, you first need to compile and install it into PHP. Most common extensions can be installed through PECL (PHP Extension Library). The following is an example of using PECL to install an extension:$ pecl install example_extension
After the installation is complete, you need to enable the extension in the PHP configuration file. You can edit the php.ini file and add the following line:
extension=example_extension.so
- Examples of using PHP extensions
The following are two common PHP extension examples that can help us enhance the functionality of our applications.
3.1. GD extension
GD extension is a library for processing images that can provide our applications with the functionality to create, edit and manipulate images. The following is an example using the GD extension to create a thumbnail:
<?php $original_image = imagecreatefromjpeg("original.jpg"); $thumbnail_image = imagecreatetruecolor(200, 200); imagecopyresampled($thumbnail_image, $original_image, 0, 0, 0, 0, 200, 200, imagesx($original_image), imagesy($original_image)); imagejpeg($thumbnail_image, "thumbnail.jpg"); imagedestroy($original_image); imagedestroy($thumbnail_image); ?>
The above code uses the functions provided by the GD library to create a copy of the original image and scale it to a size of 200x200 pixels, Finally save it as a thumbnail. This is a basic example of a GD extension. You can also find more about the functions and usage of the GD library in the PHP manual.
3.2. PDO extension
PDO extension is the database abstraction layer of PHP. It allows developers to access many different types of databases in a unified way and provides a set of object-oriented APIs. The following is an example of using the PDO extension to connect to a MySQL database and execute a query:
<?php $pdo = new PDO("mysql:host=localhost;dbname=test", "username", "password"); $query = $pdo->query("SELECT * FROM users"); while ($row = $query->fetch(PDO::FETCH_ASSOC)) { echo $row['name'] . "<br>"; } ?>
The above code creates a PDO object and uses the object to connect to the MySQL database named "test". We then executed a query and output the "name" column for each row in the result set. The PDO extension provides a series of functions for executing SQL queries and operating databases.
- Conclusion
Using PHP extensions can provide many new features and performance optimizations for our applications. In this article we introduce two common extension examples: GD and PDO. Of course, there are many other extensions available for PHP, and you can choose the appropriate extension to enhance your application according to your needs. Remember to install, enable and learn the related functions and usage before using the extension. I hope this article will help you understand and use PHP extensions!
The above is the detailed content of How to use PHP extensions to enhance the functionality of your application. For more information, please follow other related articles on the PHP Chinese website!
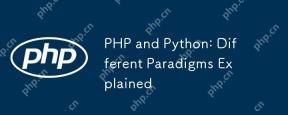
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
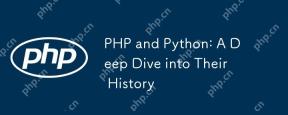
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
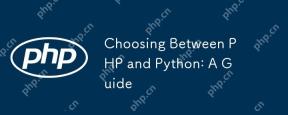
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
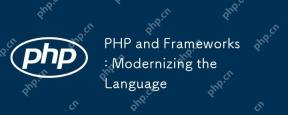
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
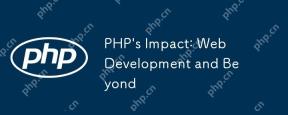
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
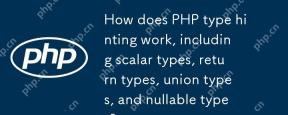
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
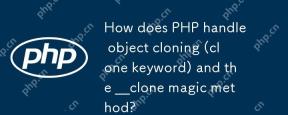
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
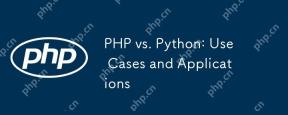
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
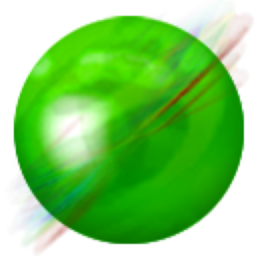
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
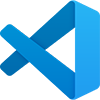
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.