


Stream API in Java 8: How to filter elements in a collection using filter() method
Stream API in Java 8: How to use the filter() method to filter elements in a collection
Introduction:
Java 8 introduces the Stream API, which provides a more concise way for us to process collections , a more efficient way. Stream is a new abstraction layer provided by Java 8, which allows us to process various data in a functional programming way. In the Stream API, the filter() method is one of the very useful methods, which can be used to filter elements in the collection. In this article, we will take a deeper look at the filter() method and explore its usage and examples.
1. What is the filter() method
The filter() method is an intermediate operation method in the Stream API. It receives a Predicate functional interface as a parameter, which is used to filter the elements in the collection. The Predicate interface defines a test(T t) method, which is used to conditionally judge the given parameters and return a Boolean value. The filter() method passes each element in the collection as a parameter to the test() method of Predicate. If true is returned, the element is retained, otherwise it is discarded.
2. How to use the filter() method
To use the filter() method, you first need to create a collection and then convert it to a Stream. Then call the filter() method and use the Predicate functional interface as parameter. The filter() method will return a new Stream containing elements that meet the criteria. Finally, process this new Stream by using the terminal operation method.
The following is a sample code showing the use of the filter() method:
import java.util.ArrayList; import java.util.List; import java.util.stream.Collectors; public class FilterExample { public static void main(String[] args) { List<Integer> numbers = new ArrayList<>(); numbers.add(1); numbers.add(2); numbers.add(3); numbers.add(4); numbers.add(5); // 使用filter()方法筛选偶数 List<Integer> evenNumbers = numbers.stream() .filter(n -> n % 2 == 0) .collect(Collectors.toList()); System.out.println("偶数列表:" + evenNumbers); } }
In the above example, we first create a List
The output result is: "Even list: [2, 4]"
3. Application scenarios of the filter() method
The filter() method has many applications in actual development Scenes. Some common scenarios are listed below:
- Filter out elements that meet a certain condition. For example, filter out all students older than 18 years old from a student list.
List<Student> adults = students.stream() .filter(s -> s.getAge() > 18) .collect(Collectors.toList());
- Filter out a range of elements. For example, filter out all numbers greater than or equal to 10 and less than or equal to 20 from a list of numbers.
List<Integer> range = numbers.stream() .filter(n -> n >= 10 && n <= 20) .collect(Collectors.toList());
- Filter out non-empty elements. For example, filter out all non-empty strings from a list of strings.
List<String> nonEmptyStrings = strings.stream() .filter(s -> s != null && !s.isEmpty()) .collect(Collectors.toList());
Summary: The
filter() method is a very commonly used method in the Stream API, which can help us efficiently filter elements in the collection. By using the filter() method, we can process data in a concise and elegant way. I hope this article will help you understand the use of the filter() method and can be used flexibly in actual projects.
The above is the detailed content of Stream API in Java 8: How to filter elements in a collection using filter() method. For more information, please follow other related articles on the PHP Chinese website!
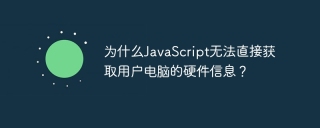
Discussion on the reasons why JavaScript cannot obtain user computer hardware information In daily programming, many developers will be curious about why JavaScript cannot be directly obtained...
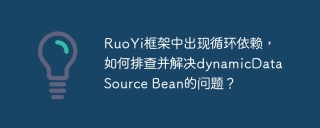
RuoYi framework circular dependency problem troubleshooting and solving the problem of circular dependency when using RuoYi framework for development, we often encounter circular dependency problems, which often leads to the program...

About SpringCloudAlibaba microservices modular development using SpringCloud...
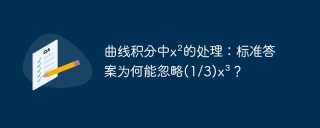
Questions about a curve integral This article will answer a curve integral question. The questioner had a question about the standard answer to a sample question...
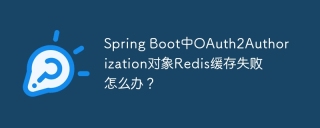
In SpringBoot, use Redis to cache OAuth2Authorization object. In SpringBoot application, use SpringSecurityOAuth2AuthorizationServer...
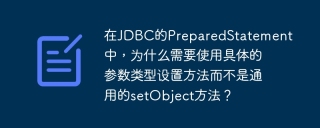
JDBC...
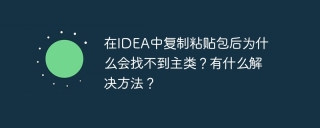
Why can't the main class be found after copying and pasting the package in IDEA? Using IntelliJIDEA...
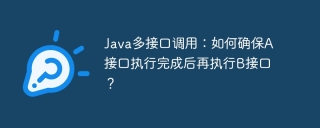
State synchronization between Java multi-interface calls: How to ensure that interface A is called after it is executed? In Java development, you often encounter multiple calls...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
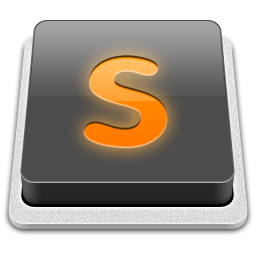
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.