Group and unmarshal data objects using the new Records class in Java 13
Use the new Records class in Java 13 to marshal and unmarshal data objects
As Java continues to evolve, each new version will introduce some new features and improvements. In Java 13, a new Records class was introduced, which provides us with a concise way to declare and use immutable data objects. In this article, we will introduce the usage of the Records class and demonstrate through some sample code how to use the Records class to marshal and unmarshal data objects.
First, let us understand the concept of Records class. The Records class is a new type that is both a class and an interface and is used to declare immutable data objects. The Records class provides default implementations, including methods such as equals(), hashCode(), and toString(). In addition, the Records class automatically creates a constructor for initializing records. Unlike ordinary classes, the Records class cannot be extended and is not allowed to define its own fields and methods.
Let us give a simple example to illustrate the usage of Records class. Suppose we have a Person object that contains name and age.
public record Person(String name, int age) {}
The above code defines a Person class, which is declared using the Records class. The Person class has two fields: name and age. Now we can create a Person object and access its fields.
Person person = new Person("Alice", 30); System.out.println(person.name()); // 输出:Alice System.out.println(person.age()); // 输出:30
As shown above, we can obtain the field value of the Person object through the accessor methods (name() and age()). In addition, the Records class also provides a default toString() method so that we can print the Person object directly.
Next, let’s look at a more complex example. Suppose we have a Student object that extends the Person object and adds the school field.
public record Student(String name, int age, String school) implements Person {}
The above code defines a Student class, which inherits from the Person class and adds a school field. At the same time, we use the implements keyword to specify that the Student class is the implementation class of the Person interface. Objects declared using the Records class can undergo class inheritance and interface implementation just like ordinary objects.
Now, let’s take a look at how to marshal and unmarshal data objects using the Records class. Suppose we want to convert the Person object to a JSON string and save it to a file.
import com.fasterxml.jackson.databind.ObjectMapper; public class PersonSerialization { public static void main(String[] args) throws Exception { Person person = new Person("Alice", 30); // 编组为JSON字符串 ObjectMapper mapper = new ObjectMapper(); String jsonString = mapper.writeValueAsString(person); // 保存为文件 FileWriter writer = new FileWriter("person.json"); writer.write(jsonString); writer.close(); System.out.println("Person对象已编组为JSON并保存到文件中"); } }
The above code uses the ObjectMapper class in the Jackson library to marshal Person objects into JSON strings and save them to a file. By calling the mapper.writeValueAsString(person) method, we can convert the Person object into a JSON string. We then use the FileWriter class to write the JSON string to the file.
The process of unmarshaling is the opposite of marshalling. Suppose we read a JSON string from a file and unmarshal it into a Person object.
import com.fasterxml.jackson.databind.ObjectMapper; public class PersonDeserialization { public static void main(String[] args) throws Exception { // 从文件中读取JSON字符串 String jsonString = Files.readString(Path.of("person.json")); // 解组为Person对象 ObjectMapper mapper = new ObjectMapper(); Person person = mapper.readValue(jsonString, Person.class); System.out.println("JSON已解组为Person对象:" + person); } }
The above code uses the Files class to read JSON strings from files, and uses the ObjectMapper class to unmarshal the JSON strings into Person objects. By calling the mapper.readValue(jsonString, Person.class) method, we can convert the JSON string into a Person object.
In summary, we have learned about the usage of the new Records class in Java 13, and demonstrated through sample code how to use the Records class to marshal and unmarshal data objects. The Records class provides us with a concise way to declare and use immutable data objects, making the code more readable and reliable. If you are using Java 13 or higher, give the Records class a try and apply it to your project.
The above is the detailed content of Group and unmarshal data objects using the new Records class in Java 13. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor
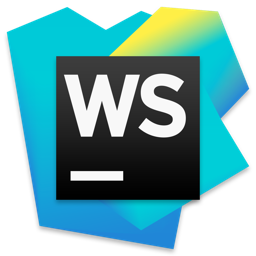
WebStorm Mac version
Useful JavaScript development tools
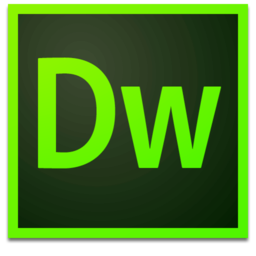
Dreamweaver Mac version
Visual web development tools
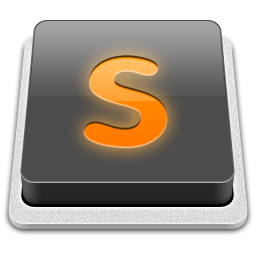
SublimeText3 Mac version
God-level code editing software (SublimeText3)