PHP and PDO: How to handle BLOB and CLOB data types
Overview:
Using PDO (PHP Data Object) as a tool for the database access layer in PHP can easily connect and operate the database. Special handling is required when processing columns containing binary data (BLOB) or long text data (CLOB). This article will introduce how to use PDO to process BLOB and CLOB type data and provide code examples.
PDO Introduction:
PDO is a PHP extension that provides a unified API to access different types of databases. It provides a set of object-oriented methods to perform database operations. Through PDO, we can use the same code to access MySQL, SQLite, PostgreSQL and other databases.
Handling BLOB data type:
BLOB (Binary Large Object) is a database column type used to store binary data. When we need to store binary files such as images, audio, and video, we can use the BLOB type. When using PDO to process BLOB data, we need to use the prepare statement to pass binary data through placeholders.
The following is an example that shows how to save a picture as a BLOB type through PDO, read it from the database and display it:
// 连接数据库 $dsn = "mysql:host=localhost;dbname=test"; $user = "username"; $password = "password"; try { $pdo = new PDO($dsn, $user, $password); } catch (PDOException $e) { echo "数据库连接失败: " . $e->getMessage(); exit; } // 保存图片为BLOB $imagePath = 'path/to/image.jpg'; $imageData = file_get_contents($imagePath); $stmt = $pdo->prepare("INSERT INTO images (data) VALUES (:data)"); $stmt->bindParam(':data', $imageData, PDO::PARAM_LOB); $stmt->execute(); // 从数据库读取并显示图片 $stmt = $pdo->query("SELECT data FROM images LIMIT 1"); $row = $stmt->fetch(PDO::FETCH_ASSOC); $imageData = $row['data']; header("Content-type: image/jpeg"); echo $imageData;
Processing CLOB data type:
CLOB ( Character Large Object) is a database column type used to store character data. When we need to store long text, rich text and other character data, we can use the CLOB type. When using PDO to process CLOB data, we can use the bindValue method to pass long text data to the placeholder.
The following is an example showing how to save long text as CLOB type through PDO, read it from the database and display it:
// 连接数据库 $dsn = "mysql:host=localhost;dbname=test"; $user = "username"; $password = "password"; try { $pdo = new PDO($dsn, $user, $password); } catch (PDOException $e) { echo "数据库连接失败: " . $e->getMessage(); exit; } // 保存长文本为CLOB $textContent = "This is a long text"; $stmt = $pdo->prepare("INSERT INTO texts (content) VALUES (:content)"); $stmt->bindValue(':content', $textContent, PDO::PARAM_STR); $stmt->execute(); // 从数据库读取并显示长文本 $stmt = $pdo->query("SELECT content FROM texts LIMIT 1"); $row = $stmt->fetch(PDO::FETCH_ASSOC); $textContent = $row['content']; echo $textContent;
Conclusion:
Use PDO to process BLOB and CLOB type data require special processing methods. For BLOB type data, we can use the bindParam method to transfer binary data; for CLOB type data, we can use the bindValue method to transfer character data. The above example code shows how to save and read BLOB and CLOB type data through PDO. Readers can make corresponding adjustments and expansions according to the actual situation.
The above is the detailed content of PHP and PDO: How to deal with BLOB and CLOB data types. For more information, please follow other related articles on the PHP Chinese website!
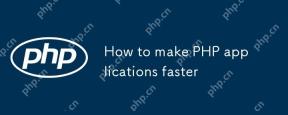
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
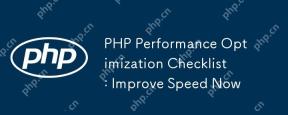
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
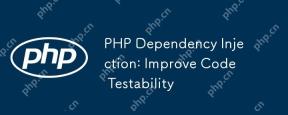
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
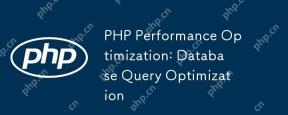
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
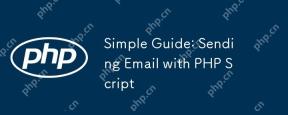
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
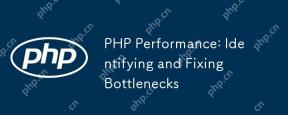
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
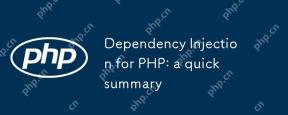
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
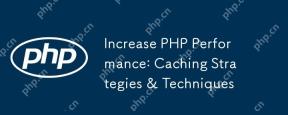
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
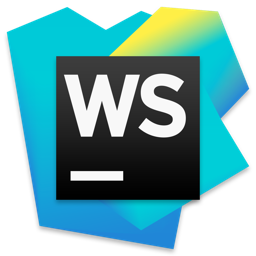
WebStorm Mac version
Useful JavaScript development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
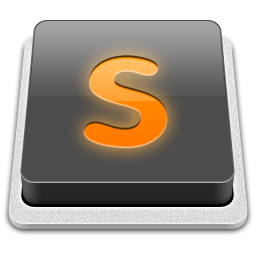
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
