


PHP data processing tips: How to use the array_filter function to filter elements in an array
PHP data processing skills: How to use the array_filter function to filter elements in an array
In PHP development, processing arrays is one of the most common tasks. Sometimes, we need to filter out elements that meet specific conditions from an array. In this case, we can use PHP's built-in array_filter function to achieve this. This article will introduce how to use the array_filter function to filter elements in an array and provide some practical code examples.
The array_filter function is a very flexible and powerful function. It accepts an array as a parameter and returns a new array that only contains elements that meet the specified conditions. Let’s take a look at the basic syntax of the array_filter function:
array array_filter ( array $array [, callable $callback [, int $flag = 0 ]] )
$array: the array to filter .
$callback: (optional) callback function for filter conditions.
$flag: (optional) Control the behavior in the callback function.
Next, I will show the use of array_filter function through several specific examples.
Example 1: Filter out the odd elements in the array
$numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; $filteredNumbers = array_filter($numbers, function($value) { return $value % 2 == 1; }); print_r($filteredNumbers);
Output result:
Array ( [0] => 1 [2] => 3 [4] => 5 [6] => 7 [8] => 9 )
In the above example, we use the array_filter function to filter out the odd elements in the array element. We pass an anonymous function as a callback function to determine whether the element is an odd number.
Example 2: Filter out strings with a length greater than 5 in the array
$names = ['John', 'Peter', 'Alice', 'David', 'Sarah']; $filteredNames = array_filter($names, function($value) { return strlen($value) > 5; }); print_r($filteredNames);
Output results:
Array ( [2] => Alice [3] => David )
In the above example, we use the array_filter function to filter out String length greater than 5 in the array. We pass an anonymous function as the callback function and use the strlen function to calculate the length of the string.
Example 3: Filter array using callback function class method
class Filter { public function isPositive($value) { return $value > 0; } } $numbers = [-1, 2, -3, 4, -5]; $filter = new Filter(); $filteredNumbers = array_filter($numbers, [$filter, 'isPositive']); print_r($filteredNumbers);
Output result:
Array ( [1] => 2 [3] => 4 )
In the above example, we defined a Filter class, which contains an isPositive Method used to determine whether a number is positive. We pass the callback function to the array_filter function by passing the class instance and method name.
Summary:
The array_filter function is a very useful function in PHP, which can help us filter elements in an array conveniently. When the filtering conditions are complex, we can use anonymous functions or class methods as callback functions. I hope this article will help you understand and use the array_filter function.
The above is the detailed content of PHP data processing tips: How to use the array_filter function to filter elements in an array. For more information, please follow other related articles on the PHP Chinese website!
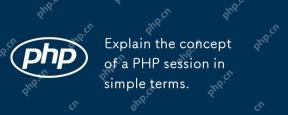
PHPsessionstrackuserdataacrossmultiplepagerequestsusingauniqueIDstoredinacookie.Here'showtomanagethemeffectively:1)Startasessionwithsession_start()andstoredatain$_SESSION.2)RegeneratethesessionIDafterloginwithsession_regenerate_id(true)topreventsessi
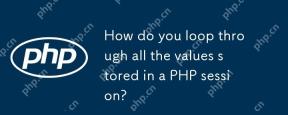
In PHP, iterating through session data can be achieved through the following steps: 1. Start the session using session_start(). 2. Iterate through foreach loop through all key-value pairs in the $_SESSION array. 3. When processing complex data structures, use is_array() or is_object() functions and use print_r() to output detailed information. 4. When optimizing traversal, paging can be used to avoid processing large amounts of data at one time. This will help you manage and use PHP session data more efficiently in your actual project.
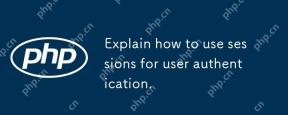
The session realizes user authentication through the server-side state management mechanism. 1) Session creation and generation of unique IDs, 2) IDs are passed through cookies, 3) Server stores and accesses session data through IDs, 4) User authentication and status management are realized, improving application security and user experience.
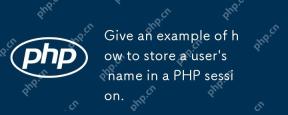
Tostoreauser'snameinaPHPsession,startthesessionwithsession_start(),thenassignthenameto$_SESSION['username'].1)Usesession_start()toinitializethesession.2)Assigntheuser'snameto$_SESSION['username'].Thisallowsyoutoaccessthenameacrossmultiplepages,enhanc
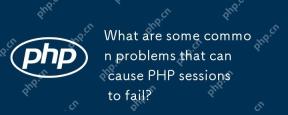
Reasons for PHPSession failure include configuration errors, cookie issues, and session expiration. 1. Configuration error: Check and set the correct session.save_path. 2.Cookie problem: Make sure the cookie is set correctly. 3.Session expires: Adjust session.gc_maxlifetime value to extend session time.
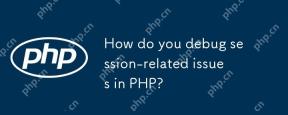
Methods to debug session problems in PHP include: 1. Check whether the session is started correctly; 2. Verify the delivery of the session ID; 3. Check the storage and reading of session data; 4. Check the server configuration. By outputting session ID and data, viewing session file content, etc., you can effectively diagnose and solve session-related problems.
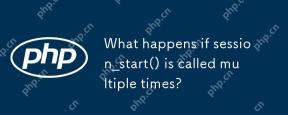
Multiple calls to session_start() will result in warning messages and possible data overwrites. 1) PHP will issue a warning, prompting that the session has been started. 2) It may cause unexpected overwriting of session data. 3) Use session_status() to check the session status to avoid repeated calls.
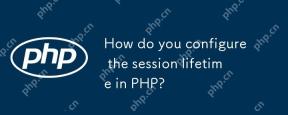
Configuring the session lifecycle in PHP can be achieved by setting session.gc_maxlifetime and session.cookie_lifetime. 1) session.gc_maxlifetime controls the survival time of server-side session data, 2) session.cookie_lifetime controls the life cycle of client cookies. When set to 0, the cookie expires when the browser is closed.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
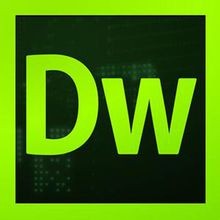
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use
