


How to implement data model correlation functionality in Ruby on Rails using MySQL
How to use MySQL to implement data model correlation function in Ruby on Rails
In Ruby on Rails development, database design and correlation are a very important part. MySQL is a commonly used relational database with powerful functions and flexible query language. It is one of the commonly used databases in Ruby on Rails. This article will introduce in detail how to use MySQL to implement the data model correlation function in Ruby on Rails, and provide code examples.
- Data model design
Before using MySQL to implement the data model association function, we first need to design the relationship between the database table structure and the model. In MySQL, there are three commonly used relationships: one-to-one, one-to-many and many-to-many.
- One-to-one association: There is only one corresponding relationship between the two tables, such as user (User) and ID card (IDCard). One user only corresponds to one ID card, and one ID card only corresponds to Corresponds to a user.
- One-to-many association: Records in one table can correspond to multiple records in another table, such as User and Order. A user can have multiple orders, but one order can only Can belong to a user.
- Many-to-many association: There are multiple correspondences between the two tables, such as user (User) and role (Role). A user can have multiple roles, and a role can also be owned by multiple users. .
- Create models and database migrations
In Ruby on Rails, we use the command line to create models and database migrations to define and create database tables and models. The following is a sample code for how to create a model and database migration for three relationships:
- One-to-one association:
# 创建用户模型 rails generate model User name:string # 创建身份证模型 rails generate model IDCard number:integer # 编辑迁移文件 class CreateIDCards < ActiveRecord::Migration[6.1] def change create_table :id_cards do |t| t.integer :number t.references :user # 添加用户外键 t.timestamps end end end # 运行数据库迁移 rails db:migrate # 编辑用户模型 class User < ApplicationRecord has_one :id_card # 声明一对一关联关系 end # 编辑身份证模型 class IDCard < ApplicationRecord belongs_to :user # 声明一对一关联关系 end
- One-to-many association:
# 创建用户模型 rails generate model User name:string # 创建订单模型 rails generate model Order number:integer user:references # 编辑迁移文件 class CreateOrders < ActiveRecord::Migration[6.1] def change create_table :orders do |t| t.integer :number t.references :user # 添加用户外键 t.timestamps end end end # 运行数据库迁移 rails db:migrate # 编辑用户模型 class User < ApplicationRecord has_many :orders # 声明一对多关联关系 end # 编辑订单模型 class Order < ApplicationRecord belongs_to :user # 声明一对多关联关系 end
- Many-to-many association:
# 创建用户模型 rails generate model User name:string # 创建角色模型 rails generate model Role name:string # 编辑迁移文件 class CreateRolesUsers < ActiveRecord::Migration[6.1] def change create_table :roles_users, id: false do |t| t.references :role t.references :user end end end # 运行数据库迁移 rails db:migrate # 编辑用户模型 class User < ApplicationRecord has_and_belongs_to_many :roles # 声明多对多关联关系 end # 编辑角色模型 class Role < ApplicationRecord has_and_belongs_to_many :users # 声明多对多关联关系 end
- Data association operation
After the database association relationship is established, We can perform data association operations, such as creating related data, querying related data, updating related data, etc. The following is sample code for operating three types of associations:
- One-to-one association:
# 创建用户和身份证 user = User.create(name: "John") id_card = IDCard.create(number: 123456, user: user) # 查询用户的身份证 user.id_card # 查询身份证的用户 id_card.user
- One-to-many association:
# 创建用户和订单 user = User.create(name: "John") order1 = Order.create(number: 1, user: user) order2 = Order.create(number: 2, user: user) # 查询用户的订单 user.orders # 查询订单的用户 order1.user order2.user
- Many-to-many association:
# 创建用户和角色 user1 = User.create(name: "John") user2 = User.create(name: "Tom") role1 = Role.create(name: "Admin") role2 = Role.create(name: "User") # 建立用户和角色的关联 user1.roles << role1 user1.roles << role2 user2.roles << role2 # 查询用户的角色 user1.roles user2.roles # 查询角色的用户 role1.users role2.users
Through the above code example, we can see how to use MySQL to implement the data model association function in Ruby on Rails. Whether it is a one-to-one, one-to-many or many-to-many relationship, it can be realized through the concise and powerful syntax provided by Rails. By properly designing the relationship between database table structures and models, and correctly operating associated data, we can help us build more efficient and flexible applications.
The above is the detailed content of How to implement data model correlation functionality in Ruby on Rails using MySQL. For more information, please follow other related articles on the PHP Chinese website!
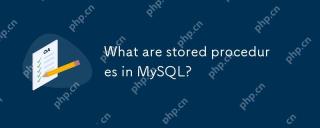
Stored procedures are precompiled SQL statements in MySQL for improving performance and simplifying complex operations. 1. Improve performance: After the first compilation, subsequent calls do not need to be recompiled. 2. Improve security: Restrict data table access through permission control. 3. Simplify complex operations: combine multiple SQL statements to simplify application layer logic.
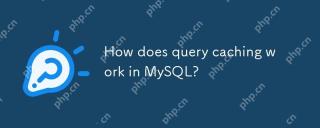
The working principle of MySQL query cache is to store the results of SELECT query, and when the same query is executed again, the cached results are directly returned. 1) Query cache improves database reading performance and finds cached results through hash values. 2) Simple configuration, set query_cache_type and query_cache_size in MySQL configuration file. 3) Use the SQL_NO_CACHE keyword to disable the cache of specific queries. 4) In high-frequency update environments, query cache may cause performance bottlenecks and needs to be optimized for use through monitoring and adjustment of parameters.
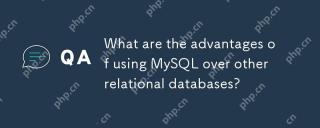
The reasons why MySQL is widely used in various projects include: 1. High performance and scalability, supporting multiple storage engines; 2. Easy to use and maintain, simple configuration and rich tools; 3. Rich ecosystem, attracting a large number of community and third-party tool support; 4. Cross-platform support, suitable for multiple operating systems.
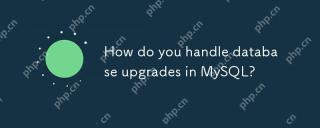
The steps for upgrading MySQL database include: 1. Backup the database, 2. Stop the current MySQL service, 3. Install the new version of MySQL, 4. Start the new version of MySQL service, 5. Recover the database. Compatibility issues are required during the upgrade process, and advanced tools such as PerconaToolkit can be used for testing and optimization.
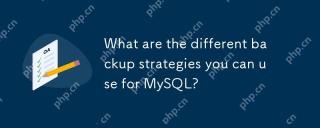
MySQL backup policies include logical backup, physical backup, incremental backup, replication-based backup, and cloud backup. 1. Logical backup uses mysqldump to export database structure and data, which is suitable for small databases and version migrations. 2. Physical backups are fast and comprehensive by copying data files, but require database consistency. 3. Incremental backup uses binary logging to record changes, which is suitable for large databases. 4. Replication-based backup reduces the impact on the production system by backing up from the server. 5. Cloud backups such as AmazonRDS provide automation solutions, but costs and control need to be considered. When selecting a policy, database size, downtime tolerance, recovery time, and recovery point goals should be considered.
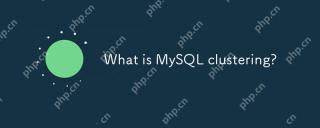
MySQLclusteringenhancesdatabaserobustnessandscalabilitybydistributingdataacrossmultiplenodes.ItusestheNDBenginefordatareplicationandfaulttolerance,ensuringhighavailability.Setupinvolvesconfiguringmanagement,data,andSQLnodes,withcarefulmonitoringandpe
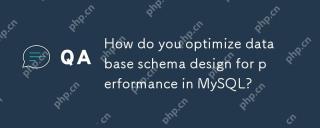
Optimizing database schema design in MySQL can improve performance through the following steps: 1. Index optimization: Create indexes on common query columns, balancing the overhead of query and inserting updates. 2. Table structure optimization: Reduce data redundancy through normalization or anti-normalization and improve access efficiency. 3. Data type selection: Use appropriate data types, such as INT instead of VARCHAR, to reduce storage space. 4. Partitioning and sub-table: For large data volumes, use partitioning and sub-table to disperse data to improve query and maintenance efficiency.
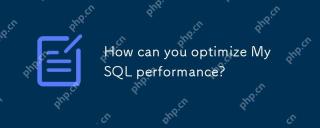
TooptimizeMySQLperformance,followthesesteps:1)Implementproperindexingtospeedupqueries,2)UseEXPLAINtoanalyzeandoptimizequeryperformance,3)Adjustserverconfigurationsettingslikeinnodb_buffer_pool_sizeandmax_connections,4)Usepartitioningforlargetablestoi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
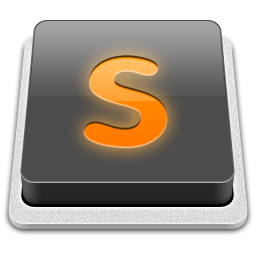
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
