


Application of Redis in JavaScript development: How to cache and speed up web page loading
Application of Redis in JavaScript development: How to cache and speed up web page loading
Introduction:
With the popularity of the Internet, the loading speed of web pages is becoming more and more important. In JavaScript development, we often encounter situations where we need to load a large amount of data, which not only increases the user's waiting time, but also consumes server resources. In order to solve this problem, we can use Redis to cache data and speed up the loading of web pages. This article will introduce the application of Redis in JavaScript development and how to use Redis to cache and speed up web page loading.
1. Introduction to Redis
Redis (Remote Dictionary Server) is an open source in-memory data storage system that can be used as a database, cache and message middleware. It supports a variety of data structures, such as strings, hash tables, lists, sets, etc., and has persistence, replication, transactions and other functions. Since Redis stores data in memory, it has very fast read and write speeds and is suitable for handling a large number of read and write operations.
2. Installation and use of Redis
- Installation of Redis
The installation of Redis is very simple. You can download and install it from the Redis official website (https://redis.io/) . After the installation is complete, run the Redis server. -
Connecting to Redis
In JavaScript development, you can use the node_redis library to connect to the Redis server. First you need to install the node_redis library:npm install redis
Then you can use the following code in the code to connect to the Redis server:
var redis = require("redis"); var client = redis.createClient();
-
Store data to Redis
When loading a web page, We can store the data that needs to be loaded into Redis for next time use. For example, we have user information that needs to be read from the database. This information can be stored in Redis:var user = { id: 1, name: "John", age: 25 }; client.set("user:1", JSON.stringify(user));
-
Reading data from Redis
Load the web page next time When, we can first read the data from Redis, use it directly if it exists, and read it from the database if it does not exist:client.get("user:1", function(err, reply) { if (reply) { var user = JSON.parse(reply); // 使用用户信息 } else { // 从数据库中读取用户信息 } });
-
Set the cache expiration time
In order to prevent After the cached data expires, the old data is still used. We can set an expiration time for the cache. For example, we can set the cache of user information for one day:client.setex("user:1", 86400, JSON.stringify(user));
-
Cache data update
When the data changes, the cached data needs to be updated. For example, when user information changes, we need to update the user information in the cache:client.set("user:1", JSON.stringify(updatedUser));
3. Use Redis to cache and accelerate web page loading
In actual web development, We can use Redis to cache some reused data to speed up the loading of web pages. The following is an example to illustrate how to use Redis to cache and speed up web page loading.
Example: Website article list
Suppose we develop a blog website and need to display a list of the latest published articles. Every time a web page is loaded, we can get the latest list of articles from the database and store it in Redis. The next time we load a web page, we can first try to read the article list from Redis. If it exists, use it directly. If it does not exist, get it from the database and store it in Redis. Here is the sample code:
// 从Redis中读取文章列表 client.get("articles:list", function(err, reply) { if (reply) { var articles = JSON.parse(reply); // 使用文章列表 renderArticles(articles); } else { // 从数据库中获取文章列表 getArticles(function(articles) { // 存储文章列表到Redis中,并设置过期时间为一小时 client.setex("articles:list", 3600, JSON.stringify(articles)); // 使用文章列表 renderArticles(articles); }); } }); // 从数据库中获取文章列表的函数 function getArticles(callback) { // 从数据库中获取文章列表 // ... var articles = [/* 最新的文章列表 */]; callback(articles); } // 渲染文章列表的函数 function renderArticles(articles) { // 渲染文章列表 }
In the above example, we first try to read the list of articles from Redis. If the Redis cache exists, the cached data is used directly without obtaining it from the database; if the Redis cache does not exist, the latest article list is obtained from the database, stored in Redis, and the expiration time is set. In this way, when the web page is loaded again within an hour, the article list cached by Redis can be used directly, eliminating the time of obtaining data from the database.
Conclusion:
By using Redis to cache data, we can speed up the loading speed of web pages and improve user experience. In JavaScript development, we can use the node_redis library to connect to the Redis server, use the set and get methods to store and read data, and use the setex method to set the expiration time of cached data. By rationally using Redis cache, you can effectively reduce the load on the server and improve the performance of web pages.
Through the above examples, we learned about the application of Redis in JavaScript development and how to use Redis to cache and accelerate web page loading. I hope this article will help you understand and apply Redis in JavaScript development!
The above is the detailed content of Application of Redis in JavaScript development: How to cache and speed up web page loading. For more information, please follow other related articles on the PHP Chinese website!
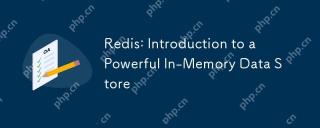
Redisisahigh-performancein-memorydatastructurestorethatexcelsinspeedandversatility.1)Itsupportsvariousdatastructureslikestrings,lists,andsets.2)Redisisanin-memorydatabasewithpersistenceoptions,ensuringfastperformanceanddatasafety.3)Itoffersatomicoper
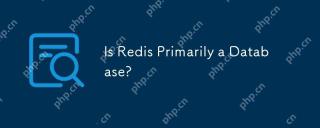
Redis is primarily a database, but it is more than just a database. 1. As a database, Redis supports persistence and is suitable for high-performance needs. 2. As a cache, Redis improves application response speed. 3. As a message broker, Redis supports publish-subscribe mode, suitable for real-time communication.
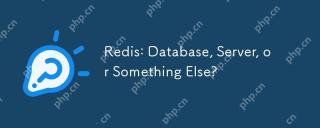
Redisisamultifacetedtoolthatservesasadatabase,server,andmore.Itfunctionsasanin-memorydatastructurestore,supportsvariousdatastructures,andcanbeusedasacache,messagebroker,sessionstorage,andfordistributedlocking.
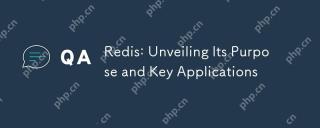
Redisisanopen-source,in-memorydatastructurestoreusedasadatabase,cache,andmessagebroker,excellinginspeedandversatility.Itiswidelyusedforcaching,real-timeanalytics,sessionmanagement,andleaderboardsduetoitssupportforvariousdatastructuresandfastdataacces
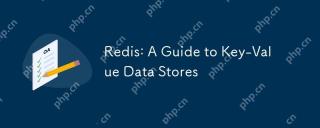
Redis is an open source memory data structure storage used as a database, cache and message broker, suitable for scenarios where fast response and high concurrency are required. 1.Redis uses memory to store data and provides microsecond read and write speed. 2. It supports a variety of data structures, such as strings, lists, collections, etc. 3. Redis realizes data persistence through RDB and AOF mechanisms. 4. Use single-threaded model and multiplexing technology to handle requests efficiently. 5. Performance optimization strategies include LRU algorithm and cluster mode.
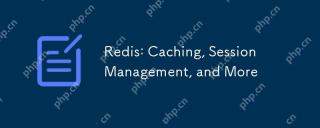
Redis's functions mainly include cache, session management and other functions: 1) The cache function stores data through memory to improve reading speed, and is suitable for high-frequency access scenarios such as e-commerce websites; 2) The session management function shares session data in a distributed system and automatically cleans it through an expiration time mechanism; 3) Other functions such as publish-subscribe mode, distributed locks and counters, suitable for real-time message push and multi-threaded systems and other scenarios.
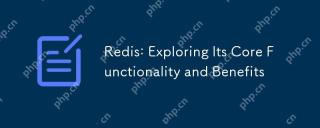
Redis's core functions include memory storage and persistence mechanisms. 1) Memory storage provides extremely fast read and write speeds, suitable for high-performance applications. 2) Persistence ensures that data is not lost through RDB and AOF, and the choice is based on application needs.
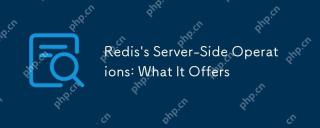
Redis'sServer-SideOperationsofferFunctionsandTriggersforexecutingcomplexoperationsontheserver.1)FunctionsallowcustomoperationsinLua,JavaScript,orRedis'sscriptinglanguage,enhancingscalabilityandmaintenance.2)Triggersenableautomaticfunctionexecutionone


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
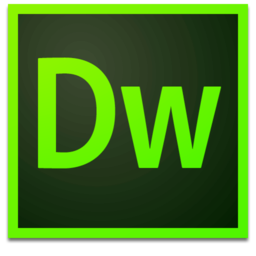
Dreamweaver Mac version
Visual web development tools
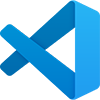
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
