


How to use the collections module for advanced data structure operations in Python 3.x
Introduction:
In Python programming, it is often necessary to process various data structures, such as lists, dictionaries, etc. However, in some specific scenarios, we may need more advanced data structures to better organize and manage data. Fortunately, Python's collections module provides some powerful data structures to help us manipulate data more efficiently. This article will introduce the common data structures of the collections module and how to use them, with code examples attached.
1. deque (double-ended queue)
The deque in the collections module is a thread-safe, variable-length double-ended queue. Its characteristic is that data can be inserted and deleted at both ends of the queue. We can use deque to implement efficient queues, stacks and other data structures.
The following is a sample code using deque:
from collections import deque queue = deque() # 创建一个空的双端队列 # 入队操作 queue.append('A') queue.append('B') queue.append('C') # 出队操作 print(queue.popleft()) # 输出:A print(queue.popleft()) # 输出:B
In the above code, we first create an empty double-ended queue, then perform the enqueue operation, and finally perform it twice Dequeue operation. The popleft() method of deque can pop an element from the left side of the queue.
2. defaultdict (default dictionary)
The defaultdict in the collections module is a dictionary with default values. It allows us to directly return a default value when accessing a non-existent key without throwing a KeyError exception. This is very convenient for some specific application scenarios, such as statistical frequency, group aggregation, etc.
The following is a sample code using defaultdict:
from collections import defaultdict # 创建一个默认值为0的字典 frequency = defaultdict(int) data = ['apple', 'banana', 'apple', 'orange', 'apple', 'banana'] # 统计每个水果的频率 for fruit in data: frequency[fruit] += 1 print(frequency) # 输出:defaultdict(<class 'int'>, {'apple': 3, 'banana': 2, 'orange': 1})
In the above code, we create a dictionary frequency with a default value of 0. Then, we loop through a fruit list data and use frequency[fruit] = 1 to count the frequency of each fruit. If a certain fruit does not exist in the dictionary, the default value 0 will be automatically returned and incremented.
3. Counter (Counter)
Counter in the collections module is a tool class used to count frequencies. It can accept any iterable object as input and produce a dictionary where the keys represent elements and the values represent the number of occurrences of that element.
The following is a sample code using Counter:
from collections import Counter data = ['apple', 'banana', 'apple', 'orange', 'apple', 'banana'] # 统计每个水果的频率 frequency = Counter(data) print(frequency) # 输出:Counter({'apple': 3, 'banana': 2, 'orange': 1}) # 获取前两个出现频率最高的水果 top2 = frequency.most_common(2) print(top2) # 输出:[('apple', 3), ('banana', 2)]
In the above code, we use Counter to count the frequency of a fruit list data and output the results. At the same time, we use the most_common() method to get the top two elements with the highest frequency.
Conclusion:
Python's collections module provides some powerful data structures that can help us operate data more efficiently. This article introduces three commonly used data structures: deque, defaultdict, and Counter, and demonstrates their use through code examples. I hope that through the introduction of this article, readers can use the collections module to perform data operations more flexibly and improve programming efficiency.
The above is the detailed content of How to use the collections module for advanced data structure operations in Python 3.x. For more information, please follow other related articles on the PHP Chinese website!
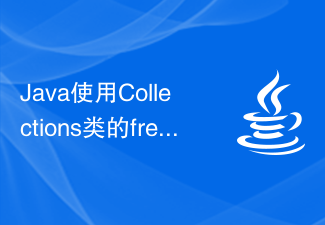
Java使用Collections类的frequency()函数计算集合中指定元素出现的次数在Java编程中,Collections类是一个包含了许多静态方法的实用类,用于对集合进行操作。其中之一是frequency()函数,用于计算集合中指定元素出现的次数。这个函数非常简单且易于使用,为Java开发人员提供了方便和灵活性。下面是一个示例代码,展示了如何使用
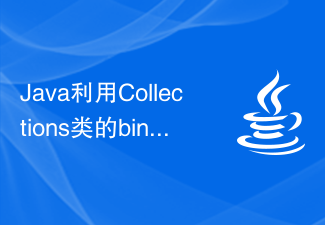
Java利用Collections类的binarySearch()函数在有序集合中进行二分查找二分查找是一种在有序集合中查找特定元素的高效算法。在Java中,我们可以利用Collections类的binarySearch()函数来实现二分查找。本文将介绍如何使用binarySearch()函数来在有序集合中进行查找,并提供具体的代码示例。二分查找算法的基本思
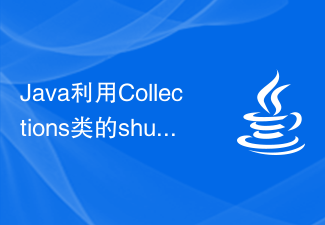
Java利用Collections类的shuffle()函数打乱集合中元素的顺序在Java编程语言中,Collections类是一个工具类,提供了各种静态方法,用于操作集合。其中之一是shuffle()函数,它可以用来打乱集合中元素的顺序。本篇文章将演示如何使用该函数,并提供相应的代码示例。首先,我们需要导入java.util包中的Collections类,
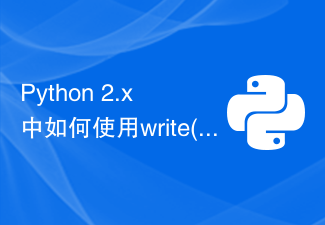
Python2.x中如何使用write()函数向文件写入内容在Python2.x中,我们可以使用write()函数将内容写入文件中。write()函数是file对象的方法之一,可用于向文件中写入字符串或二进制数据。在本文中,我将详细介绍如何使用write()函数以及一些常见的使用案例。打开文件在使用write()函数写入文件之前,我
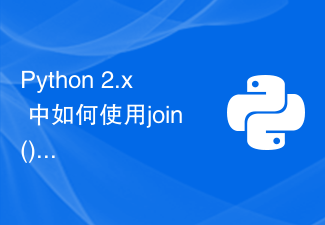
Python2.x中如何使用join()函数将字符串列表合并为一个字符串在Python中,我们经常需要将多个字符串合并成一个字符串。Python提供了多种方式来实现这个目标,其中一种常用的方式是使用join()函数。join()函数可以将一个字符串列表拼接成一个字符串,并且可以指定拼接时的分隔符。使用join()函数的基本语法如下:&
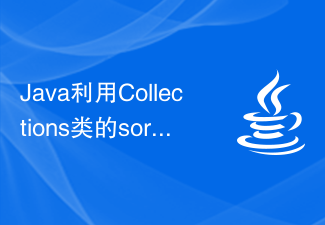
Java利用Collections类的sort()函数对集合进行排序在Java中,我们经常需要对集合进行排序。而Collections类提供了一个sort()函数,可以很方便地对集合进行排序。本文将介绍如何使用Collections类的sort()函数进行集合排序,并附带代码示例。首先,我们需要导入java.util包,以使用Collections类。imp
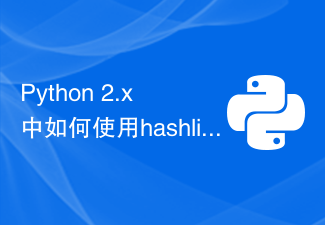
Python2.x中如何使用hashlib模块进行哈希算法计算在Python编程中,哈希算法是一种常用的算法,用于生成数据的唯一标识。Python提供了hashlib模块来进行哈希算法的计算。本文将介绍如何使用hashlib模块进行哈希算法计算,并给出一些示例代码。hashlib模块是Python标准库中的一部分,提供了多种常见的哈希算法,如MD5、SH
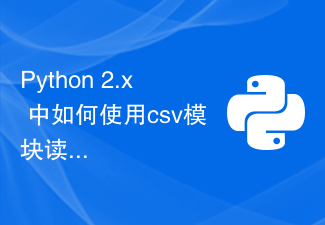
Python2.x中如何使用csv模块读取和写入CSV文件导言:CSV(CommaSeparatedValues)是一种常见的文件格式,用于存储和交换数据。Python的csv模块提供了一种简单的方式来读取和写入CSV文件。本文将介绍如何使用csv模块在Python2.x中读取和写入CSV文件,并提供相应的代码示例。一、读取CSV文件要读取CSV文


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
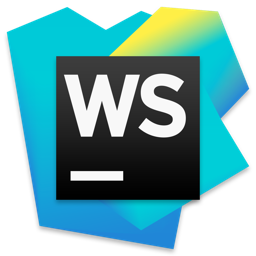
WebStorm Mac version
Useful JavaScript development tools
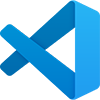
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
