


PHP calls the camera for real-time image processing: simple tutorial sharing
Real-time image processing of the camera is widely used in many scenarios, such as video surveillance, face recognition, image analysis, etc. For PHP developers, it is also feasible to achieve real-time image processing by calling the camera. This article will share a simple tutorial to teach you how to use PHP to call the camera for real-time image processing.
- Install the corresponding software and driver
To use PHP to call the camera, we need to install the corresponding software and driver first. In Windows systems, you can use the open source camera driver library OpenCV and the PHP plug-in php-opencv to achieve this. The specific installation steps are as follows:
1.1 Download and install OpenCV
You can go to the official website of OpenCV (https://opencv.org/) to download the latest version of OpenCV. Select the corresponding installation package according to your system, download and complete the installation.
1.2 Install the php-opencv plug-in
php-opencv is an extension plug-in for PHP developers to operate the OpenCV library. You can find the source code of the plug-in on GitHub (https://github.com/opencv/opencv_contrib), download it, compile and install it. The specific installation steps can be carried out according to the official documentation.
- Calling the camera and displaying real-time images
After installing the relevant software and drivers, we can start writing PHP code to call the camera and display real-time images.
<?php $video = new VideoCapture(0); // 打开默认摄像头 while (true) { $frame = $video->read(); // 读取摄像头的图像帧 if ($frame !== null) { $image = cvimencode(".bmp", $frame); // 对图像帧进行编码 echo "<img src="/static/imghwm/default1.png" data-src="data:image/bmp;base64," class="lazy" . base64_encode($image) . ""/ alt="PHP calls the camera for real-time image processing: simple tutorial sharing" >"; // 显示图像 } if (waitKey(1) >= 0) { // 按下任意键退出循环 break; } } $video->release(); // 释放摄像头资源 ?>
The above code uses the php-opencv plug-in to open the default camera and read the image frame of the camera by calling the VideoCapture class. The image frame is then encoded and the image is displayed in the browser via an echo statement. When any key is pressed, exit the loop and release the camera resources.
- Real-time image processing
In addition to displaying real-time images, we can also perform real-time processing on images. Taking face recognition as an example, we can use OpenCV's face recognition algorithm for real-time face detection.
<?php $video = new VideoCapture(0); // 打开默认摄像头 $cascade = new CascadeClassifier('haarcascade_frontalface_default.xml'); // 加载人脸识别模型 while (true) { $frame = $video->read(); // 读取摄像头的图像帧 if ($frame !== null) { $gray = cvcvtColor($frame, cvCOLOR_BGR2GRAY); // 将彩色图像转换为灰度图像 cvequalizeHist($gray, $gray); // 直方图均衡化增强对比度 $faces = $cascade->detectMultiScale($gray); // 人脸检测 foreach ($faces as $face) { cvectangle($frame, $face, new Scalar(0, 255, 0)); // 绘制人脸矩形 } $image = cvimencode(".bmp", $frame); // 对图像帧进行编码 echo "<img src="/static/imghwm/default1.png" data-src="data:image/bmp;base64," class="lazy" . base64_encode($image) . ""/ alt="PHP calls the camera for real-time image processing: simple tutorial sharing" >"; // 显示图像 } if (waitKey(1) >= 0) { // 按下任意键退出循环 break; } } $video->release(); // 释放摄像头资源 ?>
After reading the image frame from the camera, the above code first converts the color image into a grayscale image and uses histogram equalization to enhance the contrast. Then use the loaded face recognition model to perform face detection and draw the detected face rectangle. Finally, the image frames are encoded and displayed.
Through the above simple tutorial, we can use PHP to call the camera for real-time image processing. Of course, more complex algorithms and processing procedures may be required in actual applications, but this article provides an entry-level example, which I hope will be helpful to your learning of real-time image processing. If you are interested, you can further learn and explore more functions and interfaces provided by OpenCV and php-opencv.
The above is the detailed content of PHP calls the camera for real-time image processing: simple tutorial sharing. For more information, please follow other related articles on the PHP Chinese website!
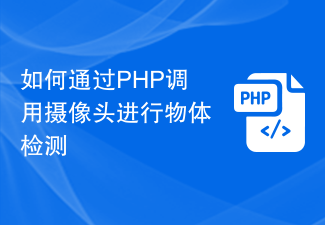
如何通过PHP调用摄像头进行物体检测摄像头在现代生活中已经变得非常普遍。我们可以利用摄像头进行各种操作,其中之一就是物体检测。本文将介绍如何使用PHP语言调用摄像头并进行物体检测。在开始之前,我们需要确保已经安装了PHP,并且可以使用摄像头。以下是使用PHP进行物体检测的步骤:安装相关库要使用PHP进行物体检测,我们首先需要安装一些必要的库。在这里,我们将使
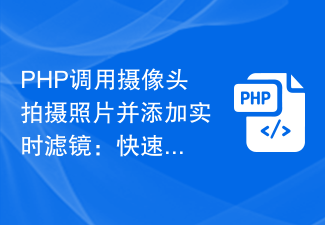
PHP调用摄像头拍摄照片并添加实时滤镜:快速入门指南摄影技术一直在不断创新和发展,而现在,我们可以利用PHP语言来调用摄像头并添加实时滤镜效果,为我们的照片增添更多乐趣。本篇文章将为您提供一份快速入门指南,教您如何使用PHP调用摄像头拍摄照片,并添加想要的实时滤镜效果。一、安装必要的组件和库首先,我们需要安装一些必要的组件和库来实现这个功能。我们需要安装以下
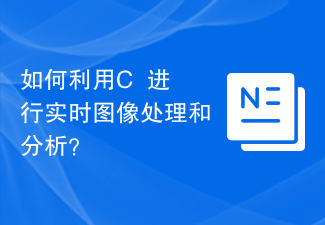
如何利用C++进行实时图像处理和分析?随着计算机视觉和图像处理的发展,越来越多的应用需要对实时图像进行处理和分析。而C++作为一种高效且强大的编程语言,被广泛应用于图像处理领域。本文将介绍如何利用C++进行实时图像处理和分析,同时提供一些代码示例。一、图像读取和显示在进行图像处理前,首先需要从文件或摄像头中读取图像数据,同时还需要将处理后的图像显示出来。首先
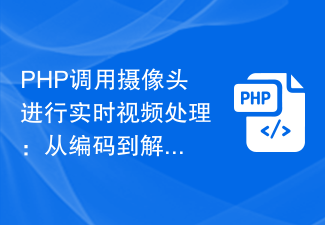
PHP调用摄像头进行实时视频处理:从编码到解码的实践摄像头即时视频处理在互联网应用中很常见,特别是在视频会议、在线教育、直播等场景下。本文将介绍如何使用PHP调用摄像头进行实时视频处理,具体包括从编码到解码的实践步骤,并附上代码示例。一、环境搭建在进行摄像头视频处理之前,我们需要确保PHP环境已经搭建好,并且已安装好相关的依赖库和扩展。可以考虑使用OpenC
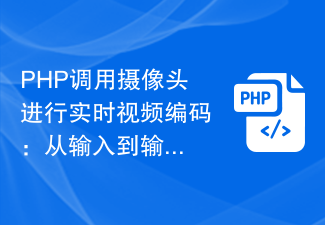
PHP调用摄像头进行实时视频编码:从输入到输出的实践摘要:本文将介绍如何使用PHP调用摄像头进行实时视频编码。我们将通过使用PHP的FFI扩展,以及调用ffmpeg库实现。关键词:PHP,摄像头,视频编码,FFI,ffmpeg引言随着现代技术的进步,越来越多的应用需要对实时视频进行处理。而PHP作为一门在Web开发中广泛应用的语言,我们经常希望能够使用PHP
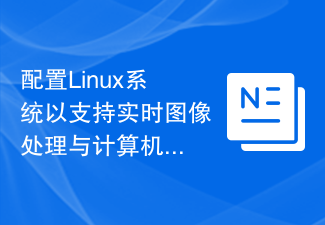
配置Linux系统以支持实时图像处理与计算机视觉开发引言:计算机视觉作为人工智能的重要分支之一,近年来在各个领域都取得了巨大的发展。实现实时图像处理和计算机视觉开发需要一个强大的平台来支持,而Linux系统作为一种自由开放且功能强大的操作系统,成为了开发者们的首选。本文将介绍如何配置Linux系统以支持实时图像处理与计算机视觉开发,并提供代码示例供读者参考。
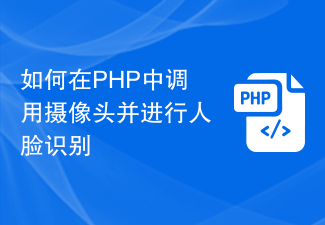
如何在PHP中调用摄像头并进行人脸识别在如今的数字化时代,人脸识别已经成为一种十分普及的技术。它广泛应用于安全门禁系统、人脸支付、人脸解锁等领域。本文将介绍如何通过PHP语言调用摄像头并进行人脸识别的方法。首先,我们需要确保计算机中已经安装好了摄像头,以及相应的摄像头驱动程序。接下来,我们需要使用PHP的拓展库来实现摄像头的调用和人脸识别的功能。在PHP中,
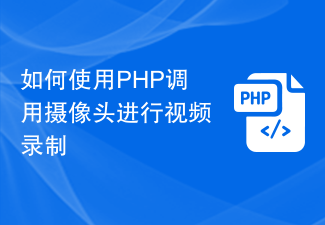
如何使用PHP调用摄像头进行视频录制随着科技的进步,摄像头已经成为人们日常生活中必备的设备之一。而在互联网应用领域,摄像头的应用也越来越多。本文将介绍如何使用PHP调用摄像头进行视频录制,并且提供相应的代码示例,希望对开发者们有所帮助。在PHP中,我们可以通过调用系统命令来实现对摄像头的操作。首先,我们需要确认系统中是否已经安装了相应的摄像头驱动程序。接下来


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
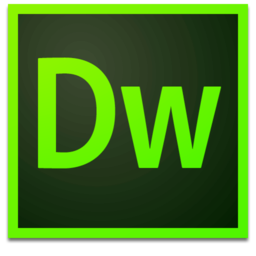
Dreamweaver Mac version
Visual web development tools
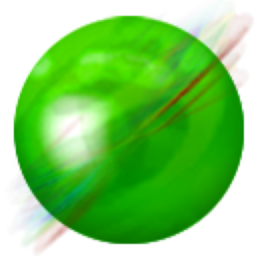
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
