


What is the method to write code in Java to draw a radar chart on a map through Baidu Map API?
What is the method to write code in Java to draw a radar chart on a map through Baidu Map API?
Overview:
Baidu Maps is a leading map and location technology service platform that provides rich API interface support, including the function of drawing radar maps. This article will introduce how to use Java to write code to draw radar charts on the map through Baidu Map API.
Steps:
The following are the steps to implement this function:
- Get the developer key of Baidu Map Open Platform: Before using Baidu Map API, you need to first Register a developer account on the map open platform and obtain the API key.
- Create a Java project and import related dependencies: Use Java development tools (such as Eclipse or IntelliJ IDEA) to create a new Java project and import the related dependency libraries of Baidu Map API.
- Initialize the map object: Initialize the map object by calling the relevant functions provided by Baidu Map API in the code. You need to provide the ID and API key of the map container.
- Draw a radar chart: Draw a radar chart on the map by calling the corresponding Baidu Map API function. Data for each indicator in the radar chart needs to be provided.
Sample code:
import com.baidu.mapapi.map.BaiduMap; import com.baidu.mapapi.map.MapView; import com.baidu.mapapi.model.LatLng; public class RadarMapDemo { public static void main(String[] args) { // 1. 获取百度地图API密钥 String ak = "your_api_key"; // 2. 创建地图容器 MapView mMapView = new MapView(); // 设置地图容器的ID mMapView.setId("your_mapview_id"); // 设置百度地图API密钥 mMapView.setApiKey(ak); // 3. 初始化地图对象 BaiduMap mBaiduMap = mMapView.getMap(); // 4. 绘制雷达图 // 创建一个指标数值数组 double[] values = { 8, 6, 9, 5, 7 }; // 创建一个雷达图中坐标点的数组 LatLng[] points = { new LatLng(39.916527, 116.397128), new LatLng(39.926668, 116.416248), new LatLng(39.93968, 116.42472), new LatLng(39.910643, 116.377359), new LatLng(39.910643, 116.377359) }; // 绘制雷达图 mBaiduMap.addOverlay(new RadarOverlayOptions().values(values).points(points)); // 5. 在activity的onDestroy()方法中销毁地图对象 mMapView.onDestroy(); } }
Note: In the above sample code, you need to replace your_api_key
with your own Baidu Map API key and set up the map container ID.
Summary:
This article introduces the method of using Java to write code to draw radar charts on the map through Baidu Map API. The function of drawing radar charts is realized by obtaining the Baidu Map API key, creating a map container, initializing the map object, and calling related functions. With sample code, you can easily implement this functionality in your own Java projects. Hope this article helps you!
The above is the detailed content of What is the method to write code in Java to draw a radar chart on a map through Baidu Map API?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
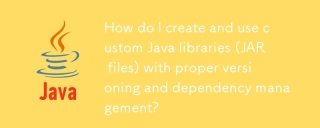
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
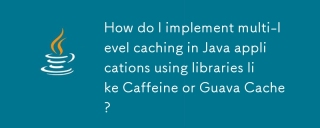
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
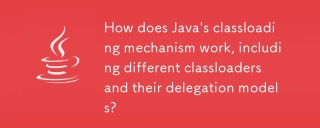
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
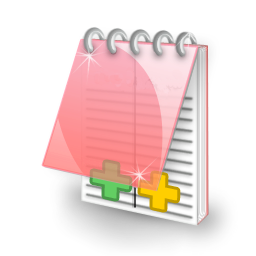
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment