


Quick Start: Use Go language functions to implement simple message push functions
Quick Start: Use Go language functions to implement simple message push functions
In today's mobile Internet era, message push has become a standard feature of various APPs. Go language is a fast and efficient programming language, which is very suitable for developing message push functions. This article will introduce how to use Go language functions to implement a simple message push function, and provide corresponding code examples to help readers get started quickly.
Before we start, we need to understand the basic principles of message push. Typically, message push functionality requires two main components: a push server and a receiving client. The push server is responsible for receiving messages sent by clients and pushing them to the corresponding receiving clients. The receiving client is responsible for receiving the pushed message and processing it accordingly.
First, let's create a simple push server. We use the net/http package in the Go language to create an HTTP server and use the WebSocket protocol for message push. The following is a sample code for a simple push server:
package main import ( "fmt" "log" "net/http" "github.com/gorilla/websocket" ) var clients = make(map[*websocket.Conn]bool) // 存储所有连接的客户端 var broadcast = make(chan []byte) // 接收消息的通道 var upgrader = websocket.Upgrader{} // WebSocket升级器 func main() { http.HandleFunc("/", handleMessage) go handleMessages() log.Println("Server running on :8080") err := http.ListenAndServe(":8080", nil) if err != nil { log.Fatal("ListenAndServe: ", err) } } func handleMessage(w http.ResponseWriter, r *http.Request) { // 将HTTP连接升级为WebSocket连接 conn, err := upgrader.Upgrade(w, r, nil) if err != nil { log.Println(err) return } // 将连接添加到clients映射表中 clients[conn] = true // 关闭连接时从clients映射表中删除连接 defer func() { delete(clients, conn) conn.Close() }() for { // 读取客户端发送的消息 _, message, err := conn.ReadMessage() if err != nil { log.Println(err) break } // 将消息发送到broadcast通道中 broadcast <- message } } func handleMessages() { for { // 从broadcast通道中读取消息 message := <-broadcast // 向所有连接的客户端发送消息 for client := range clients { err := client.WriteMessage(websocket.TextMessage, message) if err != nil { log.Println(err) client.Close() delete(clients, client) } } } }
The above code creates a WebSocket server and implements the logic to handle connections, receive messages, and send messages. When a new client connects to the server, the server will add it to the clients mapping table and process the received message through the coroutine and send it to all clients.
Next, let’s write a simple client to receive and display messages pushed by the server. The following is a sample code for a simple client based on the command line:
package main import ( "fmt" "log" "net/url" "os" "os/signal" "time" "github.com/gorilla/websocket" ) func main() { interrupt := make(chan os.Signal, 1) signal.Notify(interrupt, os.Interrupt) u := url.URL{Scheme: "ws", Host: "localhost:8080", Path: "/"} log.Printf("connecting to %s", u.String()) c, _, err := websocket.DefaultDialer.Dial(u.String(), nil) if err != nil { log.Fatal("dial:", err) } defer c.Close() done := make(chan struct{}) // 接收和显示服务器推送的消息 go func() { defer close(done) for { _, message, err := c.ReadMessage() if err != nil { log.Println("read:", err) return } fmt.Printf("received: %s ", message) } }() for { select { case <-done: return case <-interrupt: log.Println("interrupt") // 断开与服务器的连接 err := c.WriteMessage(websocket.CloseMessage, websocket.FormatCloseMessage(websocket.CloseNormalClosure, "")) if err != nil { log.Println("write close:", err) return } select { case <-done: case <-time.After(time.Second): } return } } }
The above code creates a WebSocket client, connects to the server we created previously, and receives and displays the messages pushed by the server in real time through the coroutine. . When an interrupt signal is received, the client will disconnect from the server.
By running the above two pieces of code, we can simulate a simple message push system on the command line. When the user enters a message, the server pushes it to all clients and displays it on the client. This is just a simple example, you can extend and customize it according to your actual needs.
Summary
This article introduces how to use Go language functions to implement a simple message push function. We created a WebSocket push server and wrote a simple client to receive and display messages pushed by the server. Through the sample code in this article, readers can quickly get started and understand the basic principles of the Go language to implement the message push function.
The above is the detailed content of Quick Start: Use Go language functions to implement simple message push functions. For more information, please follow other related articles on the PHP Chinese website!
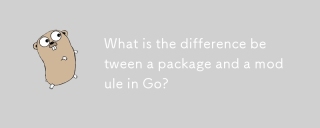
The article discusses packages and modules in Go, explaining their differences and uses. Packages organize source code, while modules manage multiple packages and their dependencies. Word count: 159.
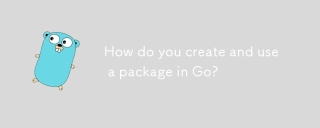
The article explains creating and using packages in Go, their benefits like code organization and reusability, managing dependencies with Go modules, and best practices for organizing packages effectively.
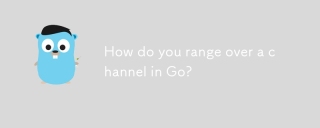
The article discusses ranging over channels in Go, highlighting its syntax, benefits like simplified syntax and automatic termination, and best practices for safely closing channels. It also covers common pitfalls to avoid.
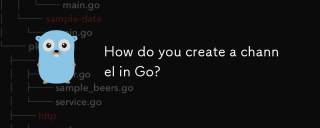
The article discusses creating and using channels in Go for concurrency management, detailing unbuffered, buffered, and directional channels. It highlights effective channel use for synchronization, data sharing, and avoiding common pitfalls like dea
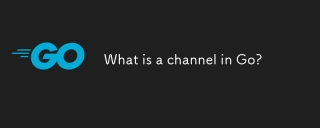
The article discusses channels in Go, a key feature for goroutine communication and synchronization. It explains how channels facilitate safe data exchange and coordination between concurrent goroutines, detailing unbuffered, buffered, directional, a
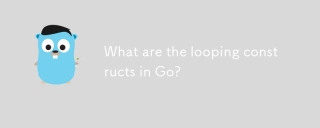
The article discusses Go's looping constructs: for loops, range loops, and while loop equivalents. It highlights the versatility and unique features of Go's for loop compared to other languages and provides best practices for using loops effectively
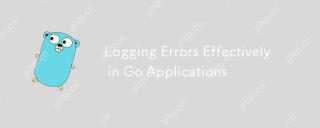
Effective Go application error logging requires balancing details and performance. 1) Using standard log packages is simple but lacks context. 2) logrus provides structured logs and custom fields. 3) Zap combines performance and structured logs, but requires more settings. A complete error logging system should include error enrichment, log level, centralized logging, performance considerations, and error handling modes.
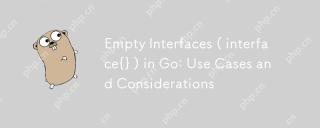
EmptyinterfacesinGoareinterfaceswithnomethods,representinganyvalue,andshouldbeusedwhenhandlingunknowndatatypes.1)Theyofferflexibilityforgenericdataprocessing,asseeninthefmtpackage.2)Usethemcautiouslyduetopotentiallossoftypesafetyandperformanceissues,


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment
