


Learn network programming functions in Go language and implement SMTP server to receive emails?
Learn the network programming functions in Go language and implement SMTP server to receive emails
As a powerful back-end development language, Go language has good network programming capabilities. In this article, we will learn how to use network programming functions in Go language to implement a basic SMTP server to receive emails.
SMTP (Simple Mail Transfer Protocol) is a standard protocol for email transmission. We will use the net/smtp package in the Go language to implement the SMTP server function. Before we start writing code, we need to make sure that the Go language environment has been installed correctly.
First, we need to import the net/smtp package and fmt package, as well as the net package to listen and receive connections. The following is a code example:
package main import ( "fmt" "log" "net" "net/smtp" ) func handleConnection(conn net.Conn) { // 打印连接信息 fmt.Println("接收到来自", conn.RemoteAddr(), "的连接") // 创建SMTP服务器 server := smtp.NewServer(conn) // 设置SMTP服务器的各项参数 server.Domain = "example.com" server.AllowInsecureAuth = true server.AuthMechanisms = []string{"PLAIN", "LOGIN"} // 设置用户验证函数 server.Authenticate = func(username, password string) error { // 这里可以进行用户验证逻辑 // 在此示例中,我们直接接受任何用户名和密码 return nil } // 开始SMTP服务器的处理循环 err := server.Process() if err != nil { log.Printf("处理连接时出错:%v ", err) } // 关闭连接 conn.Close() } func main() { // 监听本地的25端口 listener, err := net.Listen("tcp", ":25") if err != nil { log.Fatal(err) } defer listener.Close() fmt.Println("SMTP服务器已启动,正在监听端口25...") for { // 接收连接 conn, err := listener.Accept() if err != nil { log.Println("接受连接时出错:", err) continue } // 开启新的协程处理连接 go handleConnection(conn) } }
In the above code, we first listen to the local port 25 through the net.Listen
function, and then use the listener.Accept
function to receive connect. Whenever a new connection is established, we create a new SMTP server and handle the connection in a new coroutine.
In the handleConnection
function, we created a smtp.Server
object and made some settings for it. These include setting the domain name of the SMTP server, whether to allow insecure authentication, supported authentication mechanisms, etc.
Next, we customized a user verification function, which can be used for user authentication logic. In this example, we accept any username and password directly.
Finally, we start the processing loop of the SMTP server by calling the server.Process()
method. This method blocks the current coroutine until the connection is closed or an error occurs.
After running the above code, the SMTP server will listen on the local port 25. We can use the mail client to send emails to the server and receive and process these emails through processing logic in the code. In the terminal, we can see the corresponding information printed every time a new connection is received.
In summary, we have learned how to use the network programming functions in the Go language to implement a simple SMTP server. By using the net/smtp package, we can easily handle the details related to the SMTP protocol to implement a fully functional mail server. I hope this article can help you better understand the network programming functions in Go language and stimulate your interest in further exploration and application.
The above is the detailed content of Learn network programming functions in Go language and implement SMTP server to receive emails?. For more information, please follow other related articles on the PHP Chinese website!
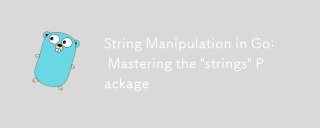
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
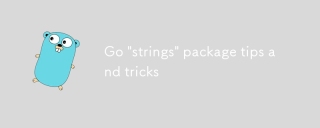
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
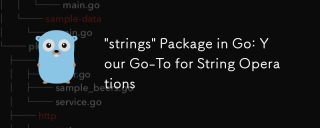
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
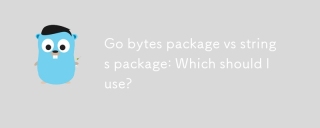
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
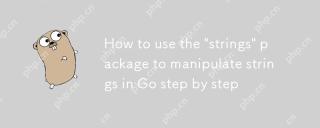
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
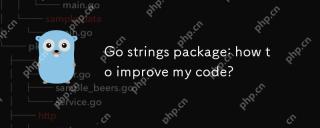
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
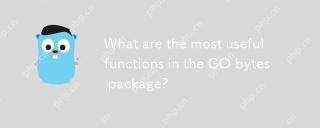
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
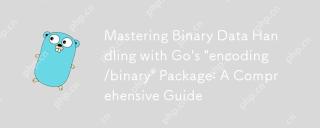
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
