How to use MySQL to implement data migration function in Django
How to use MySQL to implement data migration function in Django
Introduction:
Data migration is a very important link when developing and maintaining applications. It allows the current database to be updated when the database schema changes. Have data or migrate data to a new table structure. In Django, we can use MySQL as the database engine and use Django's own migration tool to implement data migration functions. This article will introduce in detail how to use MySQL for data migration in Django, and provide code examples for readers' reference.
Step 1: Configure the database
First, we need to configure the database in the Django configuration file (settings.py). You can add MySQL related configuration items in the DATABASES dictionary, for example:
DATABASES = { 'default': { 'ENGINE': 'django.db.backends.mysql', 'NAME': 'your_database_name', 'USER': 'your_username', 'PASSWORD': 'your_password', 'HOST': 'your_host', 'PORT': 'your_port', } }
Modify the value of each configuration item according to the actual situation. These configuration items will tell Django how to connect to the MySQL database.
Step 2: Create migration files
In Django, we use the makemigrations
command to create migration files. Migration files store instructions for making changes to the database, including creating data tables, adding fields, modifying fields, and so on. In the root directory of the project, execute the following command:
python manage.py makemigrations
Django will automatically detect model changes and generate corresponding migration files. The migration files will be saved in the app/migrations
directory, where app
is the name of your application.
Step 3: Apply migration
Use the migrate
command to apply the migration file to the database:
python manage.py migrate
Django will execute the operations defined in the migration file in sequence. Update the database structure to the latest state.
Step 4: Data Migration
Sometimes, we need to migrate existing data to a new table structure. Django provides a mechanism to implement data migration, using the RunPython
operation. We can define a function in the migration file and write the logic of data migration in the function.
For example, we have a model named User
, and now we need to add a new field age
to the model. We can add the following code to the migration file:
from django.db import migrations def migrate_user_data(apps, schema_editor): User = apps.get_model('app', 'User') for user in User.objects.all(): # 迁移数据的逻辑,这里是将原有的`age`字段设置为用户的年龄 user.age = user.age_field user.save() class Migration(migrations.Migration): dependencies = [ ('app', '0001_initial'), ] operations = [ migrations.RunPython(migrate_user_data, reverse_code=migrations.RunPython.noop), ]
In the above code, the specific data migration logic is implemented in the migrate_user_data
function. RunPython
The operation accepts two parameters, the first is the migration function, and the second is the reverse migration function (optional, used to roll back the migration). In this example, we omit the reverse migration function.
Step 5: Perform data migration
Use the migrate
command to perform data migration:
python manage.py migrate
Django will perform the operations defined in the migration file in sequence, including data migration . The logic of data migration will be executed in the migrate_user_data
function in the migration file.
Summary:
It is very simple to implement data migration function in Django using MySQL. First, you need to configure the MySQL database in the Django configuration file; secondly, use the makemigrations
command to create the migration file; then, use the migrate
command to apply the migration file to the database; finally, if necessary For data migration, the corresponding data migration logic can be defined in the migration file. The above are the detailed steps and sample code on how to use MySQL to implement data migration in Django. I hope this article can help readers in actual development.
The above is the detailed content of How to use MySQL to implement data migration function in Django. For more information, please follow other related articles on the PHP Chinese website!
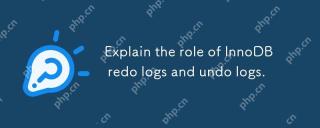
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
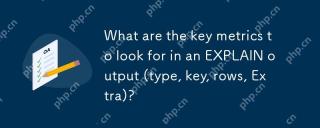
Key metrics for EXPLAIN commands include type, key, rows, and Extra. 1) The type reflects the access type of the query. The higher the value, the higher the efficiency, such as const is better than ALL. 2) The key displays the index used, and NULL indicates no index. 3) rows estimates the number of scanned rows, affecting query performance. 4) Extra provides additional information, such as Usingfilesort prompts that it needs to be optimized.
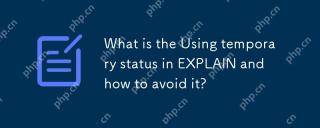
Usingtemporary indicates that the need to create temporary tables in MySQL queries, which are commonly found in ORDERBY using DISTINCT, GROUPBY, or non-indexed columns. You can avoid the occurrence of indexes and rewrite queries and improve query performance. Specifically, when Usingtemporary appears in EXPLAIN output, it means that MySQL needs to create temporary tables to handle queries. This usually occurs when: 1) deduplication or grouping when using DISTINCT or GROUPBY; 2) sort when ORDERBY contains non-index columns; 3) use complex subquery or join operations. Optimization methods include: 1) ORDERBY and GROUPB
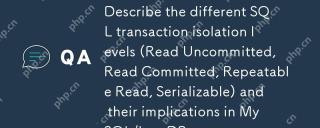
MySQL/InnoDB supports four transaction isolation levels: ReadUncommitted, ReadCommitted, RepeatableRead and Serializable. 1.ReadUncommitted allows reading of uncommitted data, which may cause dirty reading. 2. ReadCommitted avoids dirty reading, but non-repeatable reading may occur. 3.RepeatableRead is the default level, avoiding dirty reading and non-repeatable reading, but phantom reading may occur. 4. Serializable avoids all concurrency problems but reduces concurrency. Choosing the appropriate isolation level requires balancing data consistency and performance requirements.
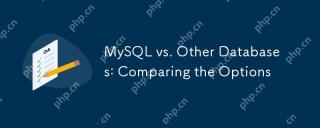
MySQL is suitable for web applications and content management systems and is popular for its open source, high performance and ease of use. 1) Compared with PostgreSQL, MySQL performs better in simple queries and high concurrent read operations. 2) Compared with Oracle, MySQL is more popular among small and medium-sized enterprises because of its open source and low cost. 3) Compared with Microsoft SQL Server, MySQL is more suitable for cross-platform applications. 4) Unlike MongoDB, MySQL is more suitable for structured data and transaction processing.
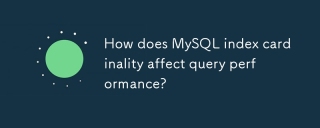
MySQL index cardinality has a significant impact on query performance: 1. High cardinality index can more effectively narrow the data range and improve query efficiency; 2. Low cardinality index may lead to full table scanning and reduce query performance; 3. In joint index, high cardinality sequences should be placed in front to optimize query.
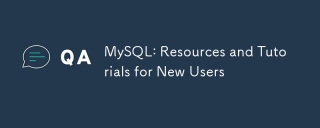
The MySQL learning path includes basic knowledge, core concepts, usage examples, and optimization techniques. 1) Understand basic concepts such as tables, rows, columns, and SQL queries. 2) Learn the definition, working principles and advantages of MySQL. 3) Master basic CRUD operations and advanced usage, such as indexes and stored procedures. 4) Familiar with common error debugging and performance optimization suggestions, such as rational use of indexes and optimization queries. Through these steps, you will have a full grasp of the use and optimization of MySQL.
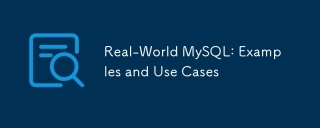
MySQL's real-world applications include basic database design and complex query optimization. 1) Basic usage: used to store and manage user data, such as inserting, querying, updating and deleting user information. 2) Advanced usage: Handle complex business logic, such as order and inventory management of e-commerce platforms. 3) Performance optimization: Improve performance by rationally using indexes, partition tables and query caches.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
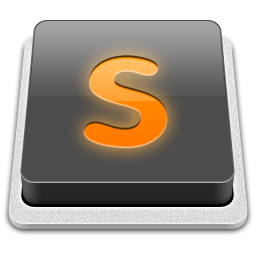
SublimeText3 Mac version
God-level code editing software (SublimeText3)