


Data structure operations of Redis and Golang: How to store and index data efficiently
Introduction:
In modern Internet applications, data storage and indexing are very important parts. Redis, as a high-performance in-memory database, combined with Golang, a powerful programming language, can help us store and index data efficiently. This article will introduce the data structure operations between Redis and Golang, and how to use them to store and index data efficiently.
1. Redis data structure
Redis supports a variety of data structures, including String, List, Hash, Set and Sorted Set). Each data structure has its specific application scenarios and operation methods.
- String(String)
String is the most basic data structure in Redis. You can set the value of a string through the SET command and obtain the value of the string through the GET command.
import "github.com/go-redis/redis" client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", DB: 0, }) err := client.Set("key", "value", 0).Err() if err != nil { panic(err) } value, err := client.Get("key").Result() if err != nil { panic(err) } fmt.Println("key:", value)
- List (List)
A list is a collection of ordered strings. You can use the LPUSH command to insert one or more elements to the left side of the list, and the RPUSH command to insert one or more elements to the right side of the list. You can use the LLEN command to get the length of the list, and the LPOP and RPOP commands to get the first and last elements of the list respectively.
// LPUSH err := client.LPush("list", "element1", "element2").Err() if err != nil { panic(err) } // RPUSH err := client.RPush("list", "element3").Err() if err != nil { panic(err) } // LLEN length, err := client.LLen("list").Result() if err != nil { panic(err) } fmt.Println("list length:", length) // LPOP value, err := client.LPop("list").Result() if err != nil { panic(err) } fmt.Println("popped value:", value) // RPOP value, err := client.RPop("list").Result() if err != nil { panic(err) } fmt.Println("popped value:", value)
- Hash(Hash)
Hash is a collection of key-value pairs. You can set the hash key-value pair through the HSET command, obtain the hash value through the HGET command, and delete the hash key-value pair through the HDEL command.
// HSET err := client.HSet("hash", "field", "value").Err() if err != nil { panic(err) } // HGET value, err := client.HGet("hash", "field").Result() if err != nil { panic(err) } fmt.Println("value:", value) // HDEL err := client.HDel("hash", "field").Err() if err != nil { panic(err) }
- Set(Set)
A set is an unordered collection of elements. You can add one or more elements to the set through the SADD command, obtain all elements of the set through the SMEMBERS command, and determine whether an element exists in the set through the SISMEMBER command.
// SADD err := client.SAdd("set", "element1", "element2").Err() if err != nil { panic(err) } // SMEMBERS elements, err := client.SMembers("set").Result() if err != nil { panic(err) } fmt.Println("elements:", elements) // SISMEMBER exists, err := client.SIsMember("set", "element1").Result() if err != nil { panic(err) } fmt.Println("element1 exists in set:", exists)
- Ordered Set (Sorted Set)
An ordered set is an ordered set of elements. You can use the ZADD command to add one or more elements with scores to the ordered set, and use the ZREVRANGE command to get the elements arranged from large to small by score.
// ZADD err := client.ZAdd("sortedset", &redis.Z{Score: 1, Member: "element1"}, &redis.Z{Score: 2, Member: "element2"}).Err() if err != nil { panic(err) } // ZREVRANGE elements, err := client.ZRevRange("sortedset", 0, -1).Result() if err != nil { panic(err) } fmt.Println("elements:", elements)
2. Combination application of Redis and Golang
Through the above example code, we understand how to use different data structures and commands of Redis to store and index data, then how to combine Redis with What about Golang combined applications? The following takes a simple sample code as an example to demonstrate how to use Redis and Golang to implement an efficient user login and record login times.
import ( "fmt" "github.com/go-redis/redis" ) func main() { // 连接Redis client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", DB: 0, }) // 用户登录 username := "user1" password := "123456" // 校验用户名和密码 if checkLogin(client, username, password) { fmt.Println("Login success!") } else { fmt.Println("Login failed!") } // 记录登录次数 loginTimes := getLoginTimes(client, username) fmt.Println("Login times:", loginTimes) } // 检查用户名和密码 func checkLogin(client *redis.Client, username string, password string) bool { // 从Redis获取密码 savedPwd, err := client.Get(username).Result() if err != nil { panic(err) } // 校验密码 if savedPwd == password { // 登录成功,增加登录次数 client.Incr(username + "_times").Result() return true } return false } // 获取登录次数 func getLoginTimes(client *redis.Client, username string) int64 { times, err := client.Get(username + "_times").Int64() if err != nil { panic(err) } return times }
In the above example code, we check whether the user name and password match through the checkLogin function. If the match is successful, the user's login times are increased through the client.Incr command, and the user's login times are obtained through the getLoginTimes function.
Conclusion:
Through Redis's data structure and Golang's programming capabilities, we can store and index data efficiently. Using the various data structures and commands provided by Redis, combined with Golang's powerful programming language features, we can implement more complex and efficient data storage and indexing functions, providing better performance and reliability for our applications.
References:
- Redis official documentation: https://redis.io/documentation
- Go Redis client library: https://github.com /go-redis/redis
The above is the entire content of this article, I hope it will be helpful to everyone. thanks for reading!
The above is the detailed content of Redis and Golang data structure operations: how to store and index data efficiently. For more information, please follow other related articles on the PHP Chinese website!
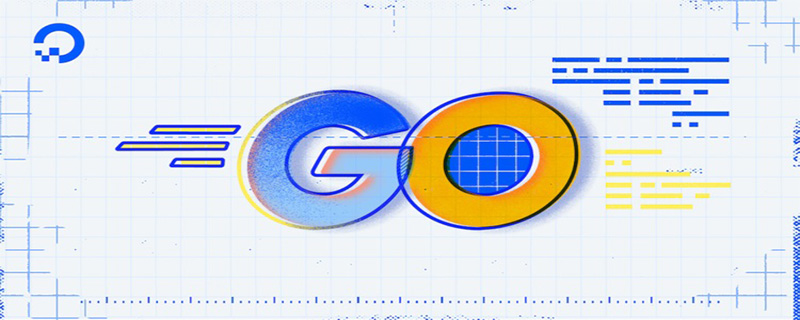
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
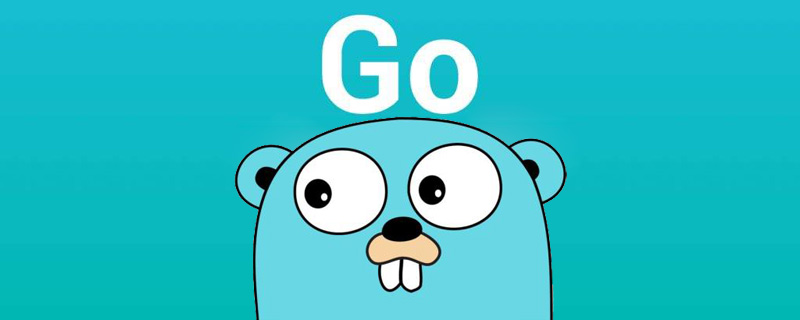
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
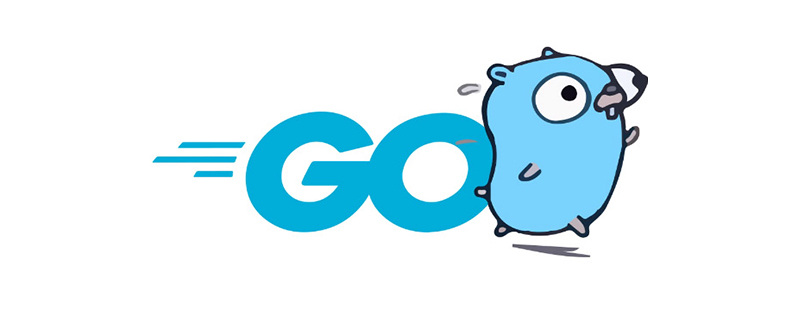
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
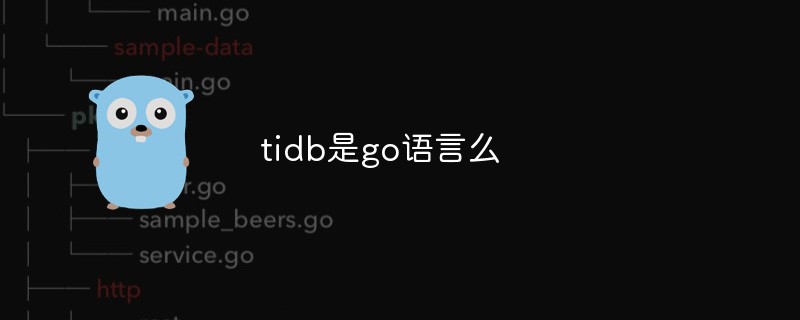
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
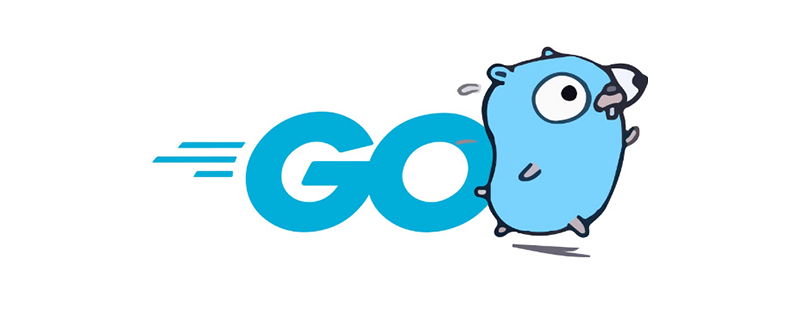
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
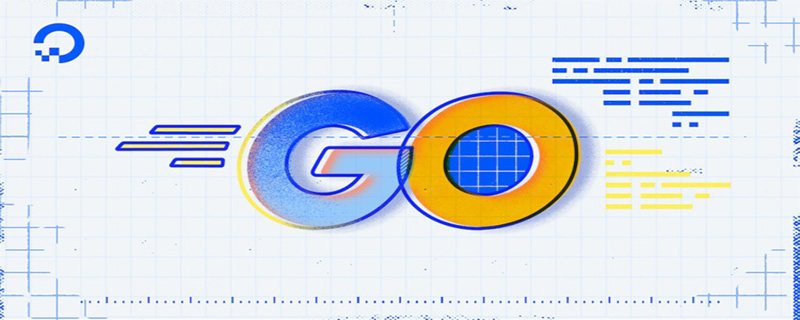
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
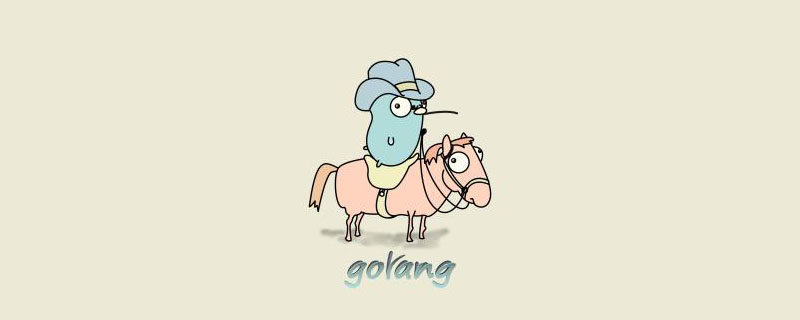
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
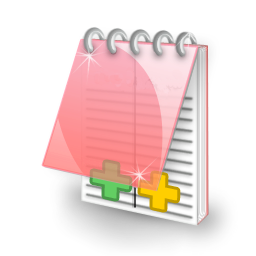
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
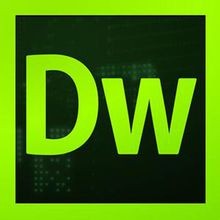
Dreamweaver CS6
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
