


PHP file upload guide: How to use the move_uploaded_file function to handle uploaded files
PHP File Upload Guide: How to use the move_uploaded_file function to process uploaded files
In developing web applications, file uploading is a common requirement. PHP provides a convenient function move_uploaded_file() for processing uploaded files. This article will introduce you how to use this function to implement the file upload function.
1. Preparation
Before you start, make sure that your PHP environment has been configured with file upload parameters. You can confirm this by opening the php.ini file and looking for the "file_uploads" parameter. If the value of this parameter is "Off", please change it to "on", save the file and restart the server.
2. Create a file upload form
First, we need to create a file upload input field in the HTML form. The following is a simple example:
<form action="upload.php" method="post" enctype="multipart/form-data"> <input type="file" name="fileToUpload" id="fileToUpload"> <input type="submit" value="上传文件" name="submit"> </form>
In the above code, we use enctype="multipart/form-data"
to specify that the form contains an input field for file upload .
3. Process file upload requests
Next, we need to create a PHP script to process file upload requests. In this script, we will use the move_uploaded_file()
function to save the uploaded file. The following is a basic example:
<?php $targetDir = "uploads/"; if(isset($_POST["submit"])){ $targetFile = $targetDir . basename($_FILES["fileToUpload"]["name"]); $uploadOk = 1; $imageFileType = strtolower(pathinfo($targetFile,PATHINFO_EXTENSION)); // 检查文件是否已经存在 if (file_exists($targetFile)) { echo "文件已经存在。"; $uploadOk = 0; } // 检查文件大小 if ($_FILES["fileToUpload"]["size"] > 500000) { echo "文件大小超过限制。"; $uploadOk = 0; } // 允许的文件格式 if($imageFileType != "jpg" && $imageFileType != "png" && $imageFileType != "jpeg" && $imageFileType != "gif" ) { echo "只允许上传JPG, JPEG, PNG, GIF格式的文件。"; $uploadOk = 0; } // 检查上传是否成功 if ($uploadOk == 0) { echo "文件上传失败。"; } else { if (move_uploaded_file($_FILES["fileToUpload"]["tmp_name"], $targetFile)) { echo "文件上传成功。"; } else { echo "文件上传失败。"; } } } ?>
In the above code, we first define a target folder $targetDir
to store uploaded files. Then, we obtain the information of the uploaded file through $_FILES["fileToUpload"]
, and use the basename()
function to obtain the file name of the uploaded file.
Next, we perform some checks on the uploaded files, such as determining whether the file already exists, whether the file size exceeds the limit, and whether the file format meets the requirements. If the check passes, we can use the move_uploaded_file()
function to move the file from the temporary directory to the target folder.
4. Display the upload results
Finally, we can display the upload results by outputting the results on the file upload form page. The following is a simple example:
<?php if(isset($_FILES["fileToUpload"]["name"])) { echo "上传的文件名:" . $_FILES["fileToUpload"]["name"] . "<br>"; echo "文件类型:" . $_FILES["fileToUpload"]["type"] . "<br>"; echo "文件大小:" . ($_FILES["fileToUpload"]["size"] / 1024) . " KB<br>"; } ?>
The above code will display the uploaded file name, file type and file size after the file upload is completed.
Summary
By using the move_uploaded_file() function, we can easily handle the file upload function in PHP. In a real application, you might also add more validation logic and file processing operations to meet your specific needs. At the same time, you must also pay attention to security protection measures, such as only allowing specified types to be uploaded, limiting the size of uploaded files, etc. I hope this article helps you understand how to use the move_uploaded_file() function to handle file uploads.
Reference link:
- PHP official documentation: https://www.php.net/manual/en/function.move-uploaded-file.php
The above is the detailed content of PHP file upload guide: How to use the move_uploaded_file function to handle uploaded files. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
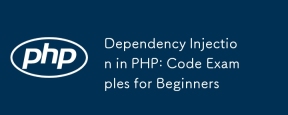
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
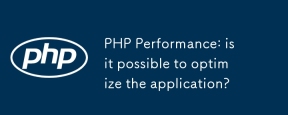
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
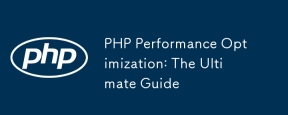
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
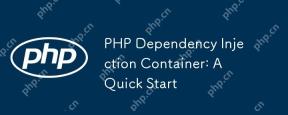
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
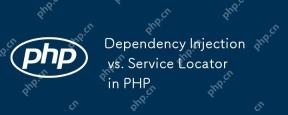
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
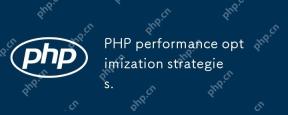
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
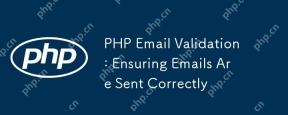
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
