


Optional class in Java 8: How to handle possibly null values using orElseThrow() method
Optional class in Java 8: How to use the orElseThrow() method to handle possibly null values
Introduction:
In Java development, we often encounter the problem of processing possibly null values Condition. In earlier versions of Java, we usually used null to indicate the absence of a value. However, there are some problems with using null. For example, we need to frequently determine whether it is null, and null pointer exceptions are prone to occur. To solve these problems, Java 8 introduced the Optional class. The Optional class provides an elegant way to handle potentially null values.
This article will introduce orElseThrow(), an important method of the Optional class, and demonstrate its use through sample code.
Basic usage of the Optional class:
The Optional class is a container class introduced in Java 8, used to wrap a value that may be null. It provides some methods to help us deal with null value situations. First, let's look at the basic usage of the Optional class.
- Creating Optional objects
You can create Optional objects through the static method Optional.ofNullable(T value). If value is null, an empty Optional object is created; if value is not null, an Optional object containing value is created.
Sample code:
String name = "Alice"; Optional<String> optionalName = Optional.ofNullable(name); // 另一种方式创建空的 Optional Optional<String> emptyOptional = Optional.empty();
- Determine whether the value of Optional exists
You can determine whether the value exists by calling the isPresent() method of the Optional object. This method returns a boolean value indicating whether the Optional object contains a non-null value.
Sample code:
if (optionalName.isPresent()) { System.out.println("存在值"); } else { System.out.println("值为空"); }
- Get the value of Optional
You can get its value by calling the get() method of the Optional object. But before calling the get() method, it is best to use the isPresent() method to determine whether the Optional exists. Because if Optional is empty, calling the get() method will throw a NoSuchElementException exception.
Sample code:
if (optionalName.isPresent()) { System.out.println("姓名为:" + optionalName.get()); } else { System.out.println("姓名为空"); }
Usage of orElseThrow():
In addition to the above basic usage, the Optional class also provides a powerful method orElseThrow(). This method will throw the specified exception when the Optional object does not contain a value.
- Use the orElseThrow() method to throw exceptions
You can specify the exception to be thrown by calling the orElseThrow(Supplier extends Throwable> exceptionSupplier) method of the Optional object. Note that what is passed in here is an abnormal constructor method reference.
Sample code:
Double price = null; Double finalPrice = Optional.ofNullable(price) .orElseThrow(() -> new IllegalArgumentException("价格为空")); System.out.println("最终价格为:" + finalPrice);
In the above code, if price is empty, an IllegalArgumentException exception will be thrown.
- Customized exception class
We can also customize an exception class to handle specific exceptions.
Sample code:
class PriceNullException extends RuntimeException { public PriceNullException(String message) { super(message); } } Double price = null; Double finalPrice = Optional.ofNullable(price) .orElseThrow(() -> new PriceNullException("价格为空")); System.out.println("最终价格为:" + finalPrice);
In the above code, if price is empty, a custom PriceNullException exception will be thrown.
- Comparison between the orElseThrow() method and other methods
Compared with the previous get() method, the orElseThrow() method will not throw a NoSuchElementException exception when the Optional is empty, but can Throws a custom exception. This makes the code cleaner and more readable.
Conclusion:
This article introduces how to use the orElseThrow() method of the Optional class in Java 8, and demonstrates how to handle possibly null values through code examples. Using the Optional class can make our code more stable and safe, and reduce the frequency of null pointer exceptions. However, you also need to be careful not to overdo it when using Optional classes, lest the code becomes verbose and difficult to understand. I hope this article can help readers better understand and use the Optional class.
The above is the detailed content of Optional class in Java 8: How to handle possibly null values using orElseThrow() method. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
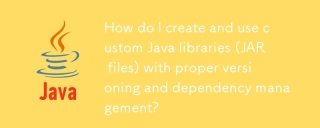
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
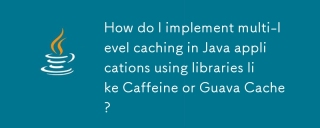
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
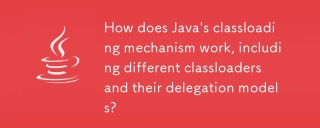
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
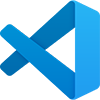
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor

SublimeText3 Chinese version
Chinese version, very easy to use