How to use PHP and SQLite for data caching and optimization
How to use PHP and SQLite for data caching and optimization
Introduction:
In the process of developing web applications, data caching and optimization are very important to improve performance and reduce the number of database queries. PHP provides rich database operation functions, while SQLite is a lightweight embedded database that is very suitable for caching data. This article will introduce how to use PHP and SQLite for data caching and optimization.
1. What is SQLite
SQLite is an embedded relational database management system that is lightweight, fast, and reliable. Its database is a separate file. Compared with other database systems, SQLite does not require additional configuration or maintenance work, making it very suitable for small projects or mobile applications.
2. Install and configure SQLite
Before using PHP and SQLite for data caching and optimization, we need to install and configure SQLite first.
1. Install SQLite extension
First, we need to ensure that PHP supports SQLite extension. Find the php.ini file in the PHP configuration, open it and find the following line:
;extension=sqlite3
Uncomment (remove the semicolon at the beginning of the line) and save the file.
2. Create a new database
In the root directory of the project, create a new SQLite database file, for example: example.db. SQLite database files have a .db extension.
3. Use PHP and SQLite for data caching
The following will introduce how to use PHP and SQLite for data caching. There are generally two ways: caching the overall data and caching query results.
1. Cache the overall data
By caching the overall data into the SQLite database, you can reduce the number of queries to the database and improve system performance.
(1) Connect to SQLite database
<?php $db = new SQLite3('example.db'); ?>
(2) Create data table
<?php $db->exec('CREATE TABLE IF NOT EXISTS cache_data (id INTEGER PRIMARY KEY, data TEXT)'); ?>
(3) Write data to cache
<?php $data = // 获取数据的逻辑 $query = $db->prepare('INSERT INTO cache_data (data) VALUES (:data)'); $query->bindValue(':data', $data, SQLITE3_TEXT); $query->execute(); ?>
(4 ) Reading cached data
<?php $result = $db->querySingle('SELECT data FROM cache_data ORDER BY id DESC LIMIT 1', true); $data = $result['data']; ?>
2. Caching query results
By caching query results, repeated query operations can be avoided and the data reading speed can be improved.
(1)Connect to SQLite database
<?php $db = new SQLite3('example.db'); ?>
(2)Create data table
<?php $db->exec('CREATE TABLE IF NOT EXISTS cache_query (id INTEGER PRIMARY KEY, query TEXT, result TEXT)'); ?>
(3)Check whether the query results have been cached
<?php $query = // 查询语句 $result = $db->querySingle('SELECT result FROM cache_query WHERE query=:query', true, [':query' => $query]); if ($result) { $data = $result['result']; } else { // 进行数据库查询的逻辑 // ... // 将查询结果写入缓存 $insertQuery = $db->prepare('INSERT INTO cache_query (query, result) VALUES (:query, :result)'); $insertQuery->bindValue(':query', $query, SQLITE3_TEXT); $insertQuery->bindValue(':result', $data, SQLITE3_TEXT); $insertQuery->execute(); } ?>
Conclusion :
Using PHP and SQLite for data caching and optimization is an effective way to improve the performance of your web applications. By caching the entire data or caching query results, the number of queries to the database can be reduced and the data reading speed can be improved. As a lightweight embedded database, SQLite is easy to use and has excellent performance. I hope this article will be helpful to you in the process of data caching and optimization using PHP and SQLite.
The above is the detailed content of How to use PHP and SQLite for data caching and optimization. For more information, please follow other related articles on the PHP Chinese website!
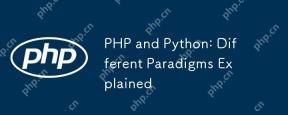
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
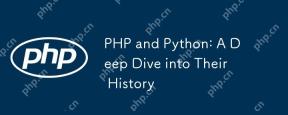
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
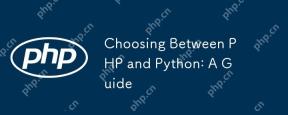
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
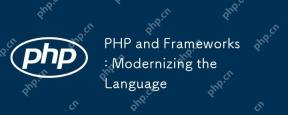
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
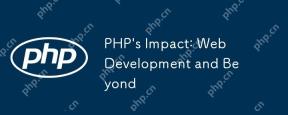
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
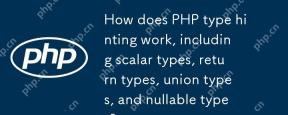
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
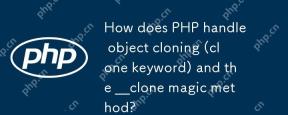
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
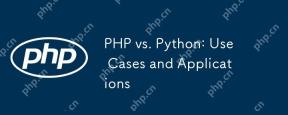
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
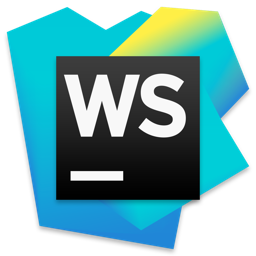
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor