How to use Redis and Haskell to build high-performance computing applications
How to use Redis and Haskell to build high-performance computing applications
Redis is a high-performance in-memory data storage and caching database, while Haskell is a powerful, statically typed programming language. Combining these two tools, we can build high-performance computing applications. This article will introduce how to combine Redis and Haskell to build such an application, and provide relevant code examples.
- Install and configure Redis
First, we need to install and configure Redis. You can download and install Redis from the official Redis website, and then start the Redis server. By default, the Redis server runs on the local port number 6379.
- Installing and Configuring Haskell
Next, we need to install and configure Haskell. The Haskell platform can be downloaded and installed from the Haskell official website. After the installation is complete, you can use GHC (Glasgow Haskell Compiler) to compile and run Haskell programs.
- Connecting to Redis using Haskell
Next, we will use Haskell to connect to the Redis server. By using the hedis library in Haskell code, we can communicate with Redis easily. The following is a simple Haskell code example:
import Database.Redis main :: IO () main = do conn <- connect defaultConnectInfo runRedis conn $ do set "key" "value" get "key" >>= liftIO . print
In this example, we first create a connection object to connect to the Redis server using the connect
function. We can then use the runRedis
function to perform interaction with Redis. In this example, we set up a key-value pair named "key", get the value of "key" using the get
function, and print it out through the liftIO
function.
- Building high-performance computing applications
With the connection to Redis, we can start building high-performance computing applications. Below is an example where we will use Redis as cache to store calculation results.
import Database.Redis import Control.Monad (when) calculate :: Int -> Int calculate n = n * 2 getCachedResult :: Redis (Maybe Int) getCachedResult = get "result" >>= return . fmap read storeResult :: Int -> Redis () storeResult result = set "result" (show result) >> return () main :: IO () main = do conn <- connect defaultConnectInfo runRedis conn $ do cachedResult <- getCachedResult case cachedResult of Just result -> liftIO $ putStrLn $ "Cached result: " ++ show result Nothing -> do let value = 5 let result = calculate value liftIO $ putStrLn $ "Calculated result: " ++ show result storeResult result
In this example, we define a calculate
function that calculates the input integer. We also define the getCachedResult
function, which will get the cached calculation results from Redis. If the cached result exists, we print it; if there is no cached result, we calculate, print and store the result in Redis.
In this way, we can use Redis as a cache in high-performance computing applications, greatly improving computing efficiency.
Summary
This article introduces how to use Redis and Haskell to build high-performance computing applications. By using the Hedis library provided by Haskell to connect and interact with Redis, we can easily build applications with excellent performance. By combining the caching capabilities of Redis, we can further optimize application performance.
I hope this article will help you understand how to use Redis and Haskell to build high-performance computing applications. Happy programming!
The above is the detailed content of How to use Redis and Haskell to build high-performance computing applications. For more information, please follow other related articles on the PHP Chinese website!
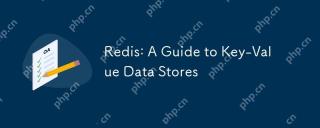
Redis is an open source memory data structure storage used as a database, cache and message broker, suitable for scenarios where fast response and high concurrency are required. 1.Redis uses memory to store data and provides microsecond read and write speed. 2. It supports a variety of data structures, such as strings, lists, collections, etc. 3. Redis realizes data persistence through RDB and AOF mechanisms. 4. Use single-threaded model and multiplexing technology to handle requests efficiently. 5. Performance optimization strategies include LRU algorithm and cluster mode.
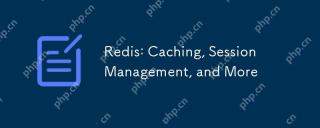
Redis's functions mainly include cache, session management and other functions: 1) The cache function stores data through memory to improve reading speed, and is suitable for high-frequency access scenarios such as e-commerce websites; 2) The session management function shares session data in a distributed system and automatically cleans it through an expiration time mechanism; 3) Other functions such as publish-subscribe mode, distributed locks and counters, suitable for real-time message push and multi-threaded systems and other scenarios.
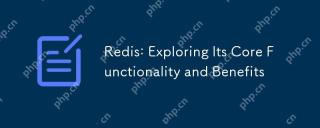
Redis's core functions include memory storage and persistence mechanisms. 1) Memory storage provides extremely fast read and write speeds, suitable for high-performance applications. 2) Persistence ensures that data is not lost through RDB and AOF, and the choice is based on application needs.
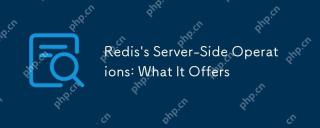
Redis'sServer-SideOperationsofferFunctionsandTriggersforexecutingcomplexoperationsontheserver.1)FunctionsallowcustomoperationsinLua,JavaScript,orRedis'sscriptinglanguage,enhancingscalabilityandmaintenance.2)Triggersenableautomaticfunctionexecutionone
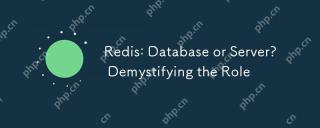
Redisisbothadatabaseandaserver.1)Asadatabase,itusesin-memorystorageforfastaccess,idealforreal-timeapplicationsandcaching.2)Asaserver,itsupportspub/submessagingandLuascriptingforreal-timecommunicationandserver-sideoperations.
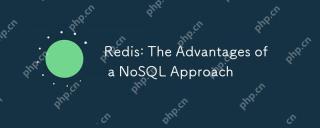
Redis is a NoSQL database that provides high performance and flexibility. 1) Store data through key-value pairs, suitable for processing large-scale data and high concurrency. 2) Memory storage and single-threaded models ensure fast read and write and atomicity. 3) Use RDB and AOF mechanisms to persist data, supporting high availability and scale-out.
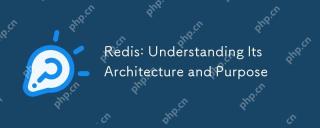
Redis is a memory data structure storage system, mainly used as a database, cache and message broker. Its core features include single-threaded model, I/O multiplexing, persistence mechanism, replication and clustering functions. Redis is commonly used in practical applications for caching, session storage, and message queues. It can significantly improve its performance by selecting the right data structure, using pipelines and transactions, and monitoring and tuning.
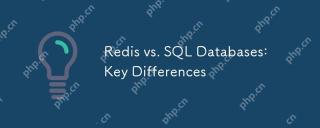
The main difference between Redis and SQL databases is that Redis is an in-memory database, suitable for high performance and flexibility requirements; SQL database is a relational database, suitable for complex queries and data consistency requirements. Specifically, 1) Redis provides high-speed data access and caching services, supports multiple data types, suitable for caching and real-time data processing; 2) SQL database manages data through a table structure, supports complex queries and transaction processing, and is suitable for scenarios such as e-commerce and financial systems that require data consistency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
