


Quick Start: Using Go language functions to implement a simple audio streaming service
Introduction:
Audio streaming services are becoming more and more popular in today's digital world, which allows us to directly Play audio files without full download. This article will introduce how to use Go language functions to quickly implement a simple audio streaming service so that you can better understand and use this function.
Step One: Preparation
First, you need to install the Go language development environment. You can download and install Go language from the official website (https://golang.org/).
Next, you need to create a new Go language project. You can create a new directory and initialize the Go module in the terminal using the following command:
$ mkdir audio-streaming-service $ cd audio-streaming-service $ go mod init github.com/your-github-username/audio-streaming-service
Next, let’s install some dependencies. Create a file named go.mod in the project directory and add the following code in it:
module github.com/your-github-username/audio-streaming-service go 1.14 require ( github.com/gorilla/mux v1.8.0 )
Save and close the file. Then, run the following command to install the required dependencies:
$ go mod tidy
Step Two: Write the Code
Now, we can start writing the code for our audio streaming service.
Open the main.go file and add the following code in it:
package main import ( "log" "net/http" "github.com/gorilla/mux" ) func main() { router := mux.NewRouter() router.HandleFunc("/stream", streamHandler).Methods("GET") log.Println("Audio streaming service started on http://localhost:8000") log.Fatal(http.ListenAndServe(":8000", router)) } func streamHandler(w http.ResponseWriter, r *http.Request) { // TODO: 实现音频流媒体逻辑 }
The above code creates a new HTTP router and defines a route processor function. We use the Gorilla Mux library to easily manage routing. In the main function, we start an HTTP server, listen on the local port 8000, and then hand over all GET requests to "/stream" to the streamHandler function for processing.
Step 3: Implement audio streaming logic
Now, we need to implement the audio streaming logic in the streamHandler function.
func streamHandler(w http.ResponseWriter, r *http.Request) { // 读取音频文件 audioFile, err := os.Open("audio.mp3") if err != nil { http.Error(w, "Failed to open audio file", http.StatusInternalServerError) return } defer audioFile.Close() // 设置响应头部 w.Header().Set("Content-Type", "audio/mpeg") w.Header().Set("Content-Disposition", "attachment; filename=audio.mp3") // 将音频文件拷贝到响应体中 _, err = io.Copy(w, audioFile) if err != nil { http.Error(w, "Failed to stream audio", http.StatusInternalServerError) return } }
The above code first attempts to open the audio file named "audio.mp3", and if it fails, returns an internal server error HTTP response. It then sets the response headers, setting Content-Type to "audio/mpeg" and Content-Disposition to "attachment; filename=audio.mp3". Finally, it copies the contents of the audio file into the response body.
Step 4: Test Run
We can run our audio streaming service locally by executing the following command:
$ go run main.go
Open the browser and visit http:/ /localhost:8000/stream, you should be able to start playing an audio file named "audio.mp3".
Conclusion:
In this article, we learned how to use Go language functions to quickly implement a simple audio streaming service. We used Gorilla Mux to manage routing and used functions in the standard library to handle reading and sending audio files. I hope the sample code and instructions in this article will be helpful to you, allowing you to better understand the implementation principles of audio streaming services, and be able to expand and delve deeper according to your own needs.
The above is the detailed content of Quick Start: Use Go language functions to implement a simple audio streaming service. For more information, please follow other related articles on the PHP Chinese website!
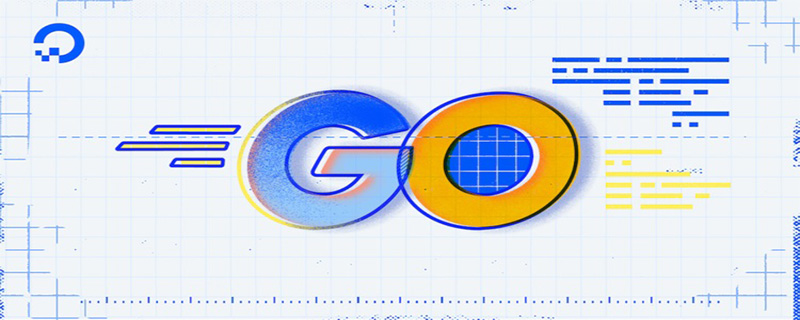
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
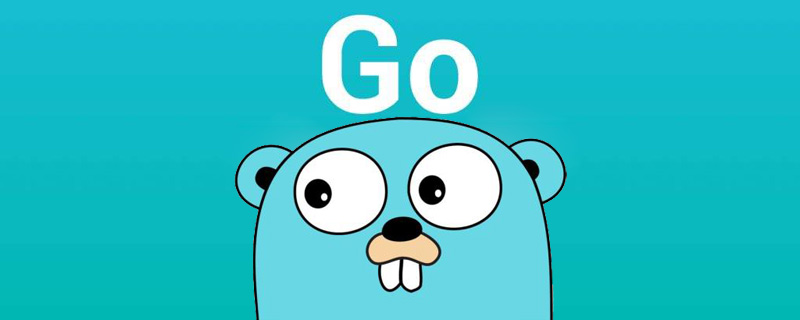
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
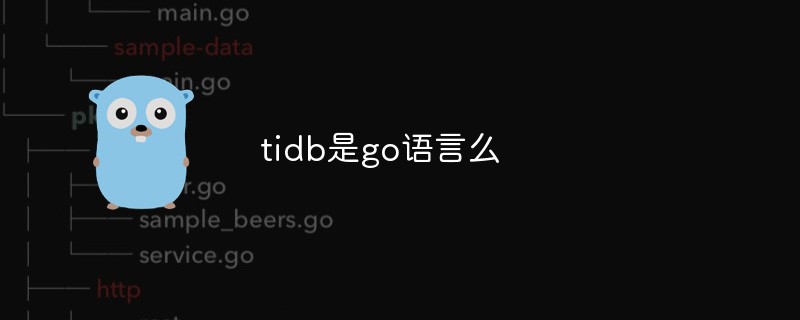
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
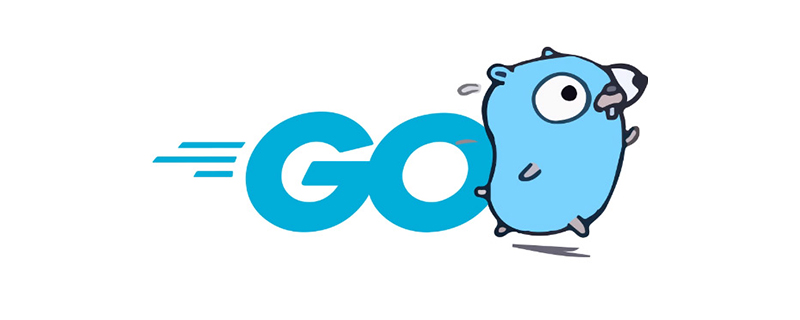
go语言能编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言。对Go语言程序进行编译的命令有两种:1、“go build”命令,可以将Go语言程序代码编译成二进制的可执行文件,但该二进制文件需要手动运行;2、“go run”命令,会在编译后直接运行Go语言程序,编译过程中会产生一个临时文件,但不会生成可执行文件。
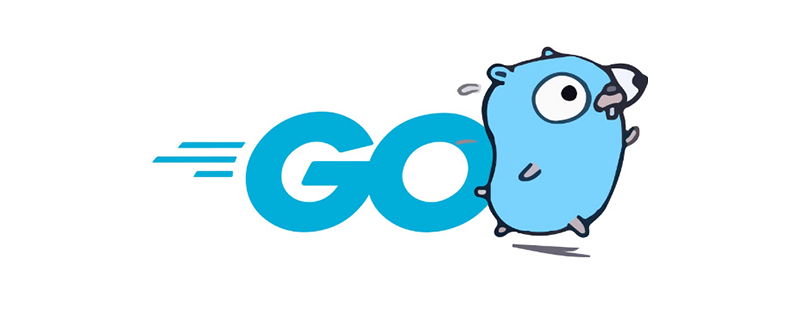
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
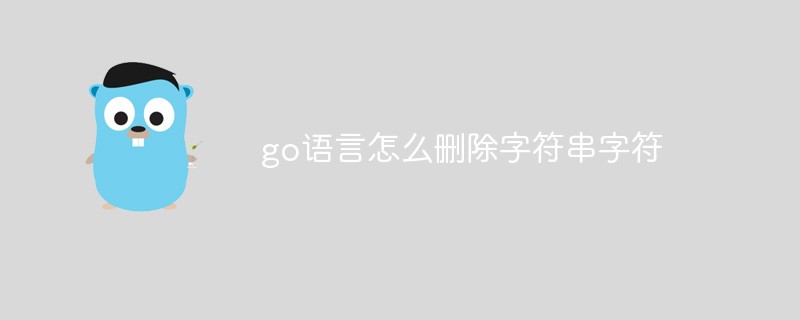
删除字符串的方法:1、用TrimSpace()来去除字符串空格;2、用Trim()、TrimLeft()、TrimRight()、TrimPrefix()或TrimSuffix()来去除字符串中全部、左边或右边指定字符串;3、用TrimFunc()、TrimLeftFunc()或TrimRightFunc()来去除全部、左边或右边指定规则字符串。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
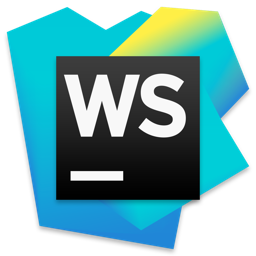
WebStorm Mac version
Useful JavaScript development tools
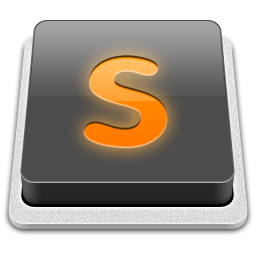
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
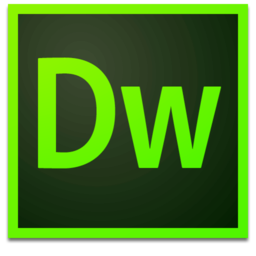
Dreamweaver Mac version
Visual web development tools
