


Developing with MySQL and CoffeeScript: How to implement data search function
Development using MySQL and CoffeeScript: How to implement data search function
Introduction:
In Web applications, data search function is very common and important. Whether it’s an e-commerce website or a social media platform, users want to be able to find the information they need quickly and accurately. This article will introduce how to use MySQL and CoffeeScript to implement a simple data search function, and attach corresponding code examples.
1. Preparation:
Before starting, we need to first ensure that the MySQL database and CoffeeScript compiler have been installed. You can choose the appropriate installation method according to your operating system and preferences.
2. Database design:
Suppose we have a table named "products", which contains the following fields:
- id: product ID (primary key)
- name: Product name
- price: Product price
- description: Product description
3. Server-side code:
- First, create a file named "search.coffee" for writing server-side code.
- In the file, first introduce the MySQL module and create a connection to the database. The specific code is as follows:
mysql = require 'mysql' # 创建与数据库的连接 connection = mysql.createConnection( host: 'localhost', user: 'root', password: '', database: 'my_database' ) # 连接数据库 connection.connect((err) -> if err throw err console.log 'Connected to MySQL database' ) # 在此处编写其他相关服务器端代码
- Next, we can write an API interface to receive search keywords and return matching data. The code is as follows:
# 创建搜索API接口 app.get '/api/search', (req, res) -> # 获取搜索关键字 keyword = req.query.keyword # 在数据库中执行搜索 sql = 'SELECT * FROM products WHERE name LIKE ? OR description LIKE ?' params = ['%' + keyword + '%', '%' + keyword + '%'] connection.query sql, params, (err, results) -> if err throw err res.json results # 关闭数据库连接 connection.end()
4. Client code:
- Create an HTML file named "search.html" and add a text box and A button for entering search keywords and triggering the search function. The code is as follows:
<!DOCTYPE html> <html> <head> <title>Data Search</title> </head> <body> <input type="text" id="keyword"> <button onclick="search()">Search</button> <div id="results"></div> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script> <script src="search.js"></script> </body> </html>
- Create a CoffeeScript file named "search.coffee" and add the JavaScript code used to send the search request in the file. The code is as follows:
search = -> # 获取搜索关键字 keyword = document.getElementById('keyword').value # 发送搜索请求 $.get( '/api/search', {keyword: keyword}, (data) -> # 将搜索结果显示在页面上 resultsDiv = document.getElementById('results') resultsDiv.innerHTML = '' for product in data resultItem = document.createElement('div') resultItem.innerHTML = product.name resultsDiv.appendChild(resultItem) )
- Create a JavaScript file named "search.js" and use the CoffeeScript compiler to compile the "search.coffee" file into a JavaScript file.
5. Run the application:
- In the terminal, enter the directory where the server-side code is stored, and execute the following command to start the server:
coffee search.coffee
- Open the "search.html" file in the browser and you will see a simple search interface.
- Enter keywords and click the "Search" button, you will see the product names matching the keywords displayed on the page.
Conclusion:
Through the above steps, we successfully implemented a simple data search function using MySQL and CoffeeScript. Of course, this is just a basic example, and actual applications may require more complex search logic and interface design. I hope this article can help readers better understand and apply related technologies, and further expand and optimize their projects.
Reference materials:
- MySQL official documentation: https://dev.mysql.com/doc/
- CoffeeScript official documentation: https://coffeescript. org/
The above is the detailed content of Developing with MySQL and CoffeeScript: How to implement data search function. For more information, please follow other related articles on the PHP Chinese website!
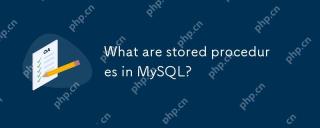
Stored procedures are precompiled SQL statements in MySQL for improving performance and simplifying complex operations. 1. Improve performance: After the first compilation, subsequent calls do not need to be recompiled. 2. Improve security: Restrict data table access through permission control. 3. Simplify complex operations: combine multiple SQL statements to simplify application layer logic.
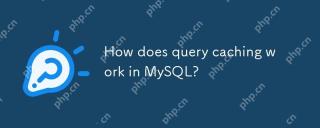
The working principle of MySQL query cache is to store the results of SELECT query, and when the same query is executed again, the cached results are directly returned. 1) Query cache improves database reading performance and finds cached results through hash values. 2) Simple configuration, set query_cache_type and query_cache_size in MySQL configuration file. 3) Use the SQL_NO_CACHE keyword to disable the cache of specific queries. 4) In high-frequency update environments, query cache may cause performance bottlenecks and needs to be optimized for use through monitoring and adjustment of parameters.
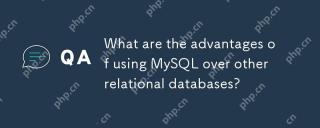
The reasons why MySQL is widely used in various projects include: 1. High performance and scalability, supporting multiple storage engines; 2. Easy to use and maintain, simple configuration and rich tools; 3. Rich ecosystem, attracting a large number of community and third-party tool support; 4. Cross-platform support, suitable for multiple operating systems.
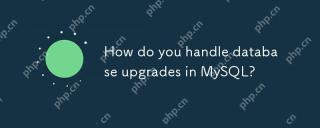
The steps for upgrading MySQL database include: 1. Backup the database, 2. Stop the current MySQL service, 3. Install the new version of MySQL, 4. Start the new version of MySQL service, 5. Recover the database. Compatibility issues are required during the upgrade process, and advanced tools such as PerconaToolkit can be used for testing and optimization.
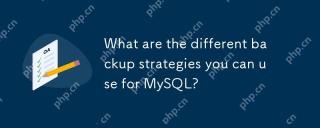
MySQL backup policies include logical backup, physical backup, incremental backup, replication-based backup, and cloud backup. 1. Logical backup uses mysqldump to export database structure and data, which is suitable for small databases and version migrations. 2. Physical backups are fast and comprehensive by copying data files, but require database consistency. 3. Incremental backup uses binary logging to record changes, which is suitable for large databases. 4. Replication-based backup reduces the impact on the production system by backing up from the server. 5. Cloud backups such as AmazonRDS provide automation solutions, but costs and control need to be considered. When selecting a policy, database size, downtime tolerance, recovery time, and recovery point goals should be considered.
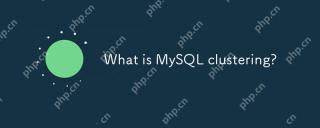
MySQLclusteringenhancesdatabaserobustnessandscalabilitybydistributingdataacrossmultiplenodes.ItusestheNDBenginefordatareplicationandfaulttolerance,ensuringhighavailability.Setupinvolvesconfiguringmanagement,data,andSQLnodes,withcarefulmonitoringandpe
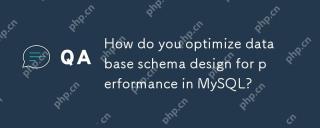
Optimizing database schema design in MySQL can improve performance through the following steps: 1. Index optimization: Create indexes on common query columns, balancing the overhead of query and inserting updates. 2. Table structure optimization: Reduce data redundancy through normalization or anti-normalization and improve access efficiency. 3. Data type selection: Use appropriate data types, such as INT instead of VARCHAR, to reduce storage space. 4. Partitioning and sub-table: For large data volumes, use partitioning and sub-table to disperse data to improve query and maintenance efficiency.
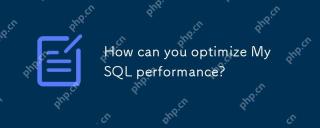
TooptimizeMySQLperformance,followthesesteps:1)Implementproperindexingtospeedupqueries,2)UseEXPLAINtoanalyzeandoptimizequeryperformance,3)Adjustserverconfigurationsettingslikeinnodb_buffer_pool_sizeandmax_connections,4)Usepartitioningforlargetablestoi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use
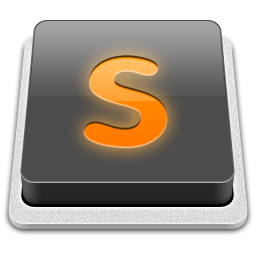
SublimeText3 Mac version
God-level code editing software (SublimeText3)
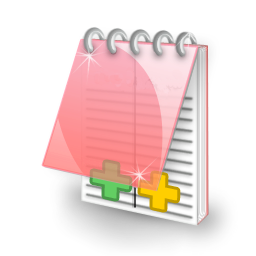
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
