


How to use the HTTP server function in Go language to implement the cache refresh function of dynamic routing?
How to use the HTTP server function in the Go language to implement the cache refresh function of dynamic routing?
In web development, the caching function is one of the important means to improve performance and reduce server load. When the server returns the same response, the client can obtain the data directly from the cache, reducing requests to the server. However, in some cases, we may need to dynamically refresh the cache to ensure that the data obtained by the client is always up to date. This article will introduce how to use the HTTP server function in the Go language to implement the cache refresh function of dynamic routing.
First, we need to implement an HTTP server and set routing rules. The "net/http" package in the Go language provides the ServerMux type to implement routing functions. We can register a handler function by calling the http.HandleFunc
or http.Handle
method. Below is a simple example showing how to implement a basic HTTP server.
package main import ( "fmt" "io" "net/http" ) func main() { http.HandleFunc("/", helloHandler) http.ListenAndServe(":8080", nil) } func helloHandler(w http.ResponseWriter, r *http.Request) { io.WriteString(w, "Hello, world!") }
In the above example, we register the helloHandler
method as the handler function of the root route by calling the http.HandleFunc
method. Then, we call the http.ListenAndServe
method to start the server and listen on port 8080.
Next, we will add a dynamic routing cache refresh function to the HTTP server. When a client requests a specific resource, the server first checks to see if a copy of the resource exists in the cache. If there is, the server will return the resource in the cache to the client; otherwise, the server will regenerate the resource and store it in the cache. In order to implement this function, we need to use the http.Handler
interface and a custom Cache type.
First, we define a Cache type to store cache data of resources.
type Cache struct { data map[string]string } func NewCache() *Cache { return &Cache{ data: make(map[string]string), } } func (c *Cache) Get(key string) (string, bool) { value, ok := c.data[key] return value, ok } func (c *Cache) Set(key, value string) { c.data[key] = value } func (c *Cache) Delete(key string) { delete(c.data, key) }
In the above code, we use a map to store the cache data of the resource. The Cache type includes methods such as Get, Set, and Delete, which are used to operate cached data.
Next, we modify the previous HTTP server code and use the Cache type to implement the cache refresh function.
package main import ( "fmt" "io" "net/http" ) type Cache struct { data map[string]string } func NewCache() *Cache { return &Cache{ data: make(map[string]string), } } func (c *Cache) Get(key string) (string, bool) { value, ok := c.data[key] return value, ok } func (c *Cache) Set(key, value string) { c.data[key] = value } func (c *Cache) Delete(key string) { delete(c.data, key) } func main() { cache := NewCache() http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { if cacheValue, ok := cache.Get(r.URL.Path); ok { io.WriteString(w, cacheValue) return } value := generateResource(r.URL.Path) // 生成资源 cache.Set(r.URL.Path, value) // 将资源存入缓存 io.WriteString(w, value) }) http.ListenAndServe(":8080", nil) } func generateResource(path string) string { // 根据path生成相应的资源,这里假设资源内容为"Resource: {path}" return "Resource: " + path }
In the above code, we first create a Cache instance cache and pass it as a parameter to the http.HandleFunc
function. In the request handling function, we first check if a copy of the requested resource exists in the cache. If it exists, we fetch and return the resource data directly from the cache. Otherwise, we call the generateResource
method to generate the resource and store it in the cache. Finally, we write the resource data to the response body.
Through the above steps, we successfully implemented the cache refresh function of dynamic routing using the HTTP server function in the Go language. In actual projects, we can further improve the caching mechanism according to needs, and add functions such as cache expiration time and cache storage methods to meet specific business needs.
The above is the detailed content of How to use the HTTP server function in Go language to implement the cache refresh function of dynamic routing?. For more information, please follow other related articles on the PHP Chinese website!
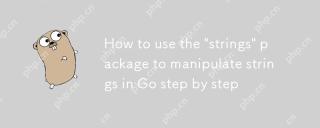
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
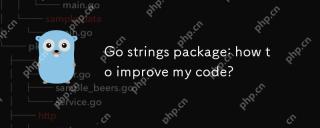
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
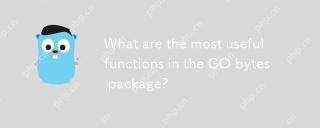
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
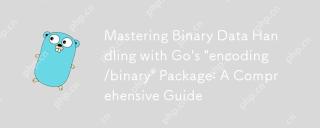
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
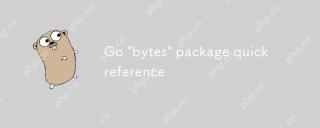
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
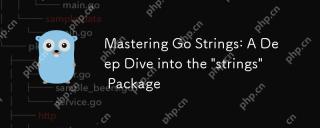
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
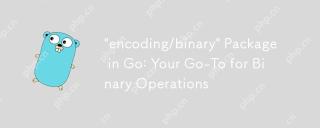
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
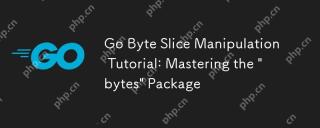
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
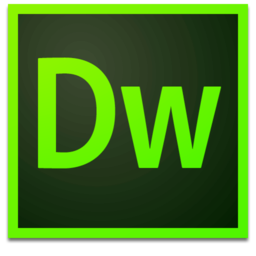
Dreamweaver Mac version
Visual web development tools
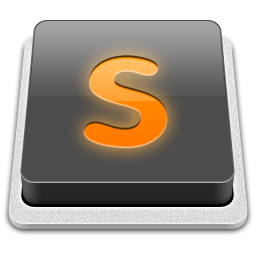
SublimeText3 Mac version
God-level code editing software (SublimeText3)
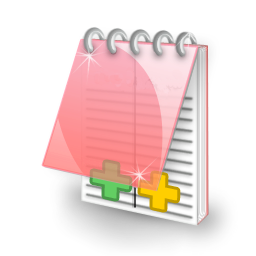
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
