Redis and Kotlin development: building efficient data persistence solutions
Redis and Kotlin development: Building efficient data persistence solutions
Introduction:
In modern software development, data persistence is a very important aspect. We need an efficient and reliable way to store and read data. Redis is a popular in-memory database, while Kotlin is a powerful and easy-to-use programming language. This article will introduce how to use Redis and Kotlin to build an efficient data persistence solution.
- Introduction to Redis
Redis is an open source, memory-based data structure storage system. It provides many data structures, such as strings, hashes, lists, sets, ordered sets, etc., and supports rich operations. Redis has the characteristics of high-speed reading and writing, persistence, and scalability, and is widely used in scenarios such as caching, message queues, and real-time statistics. - Introduction to Kotlin
Kotlin is a statically typed programming language developed by JetBrains that can be compiled to Java bytecode or JavaScript. It inherits the powerful functions of Java while providing a more concise, safe and efficient syntax. Kotlin is widely used in Android development and is gradually becoming popular in other fields. - Using Redis and Kotlin
To build an efficient data persistence solution using Redis and Kotlin, we first need to connect to the Redis server. The following is a sample code for connecting to Redis using the Jedis library:
import redis.clients.jedis.Jedis fun main() { val jedis = Jedis("localhost") jedis.connect() println("Connected to Redis") jedis.set("key", "value") val value = jedis.get("key") println("Value: $value") jedis.disconnect() println("Disconnected from Redis") }
In this example, we create a Jedis object and connect to the local Redis server through the connect
method. Then, we use the set
method to set a key-value pair, and use the get
method to get the value corresponding to the key. Finally, we disconnect from Redis through the disconnect
method.
- Encapsulating Redis operations
In order to use Redis more conveniently, we can create a RedisUtil class to encapsulate commonly used operations. The following is a simple example:
import redis.clients.jedis.Jedis class RedisUtil { private val jedis = Jedis("localhost") init { jedis.connect() } fun set(key: String, value: String) { jedis.set(key, value) } fun get(key: String): String? { return jedis.get(key) } fun disconnect() { jedis.disconnect() } }
Using the encapsulated RedisUtil class, we can perform Redis operations more conveniently. The following is an example of usage:
fun main() { val redisUtil = RedisUtil() redisUtil.set("key", "value") val value = redisUtil.get("key") println("Value: $value") redisUtil.disconnect() }
- Persistent data
In addition to storing in memory, Redis also supports persisting data to disk. This ensures that data is not lost after a power outage or restart. Redis provides two persistence methods, namely RDB and AOF. RDB is a snapshot method that saves a copy of the current data; while AOF records each write command in the form of a log.
To enable the persistence function, we can make the corresponding settings in the Redis configuration file. The following is a simple example:
# redis.conf save 60 1 dir /var/lib/redis appendonly yes
In this example, we save the RDB snapshot to the directory /var/lib/redis
, execute it every 60 seconds, and turn on the AOF log .
- Summary
This article introduces how to use Redis and Kotlin to build an efficient data persistence solution. We learned how to connect to a Redis server and perform basic data operations using the Jedis library. We also encapsulate Redis operations to improve code readability and ease of use. Finally, we learned about the persistence mechanism of Redis and simply configured it.
Redis and Kotlin provide powerful and flexible data persistence tools that can meet the needs of various scenarios. I hope this article can be helpful to your work in data persistence. I wish you success in your development!
Reference materials:
- Redis official website: https://redis.io/
- Kotlin official website: https://kotlinlang.org/
- Jedis GitHub repository: https://github.com/redis/jedis
The above is the detailed content of Redis and Kotlin development: building efficient data persistence solutions. For more information, please follow other related articles on the PHP Chinese website!
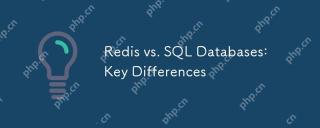
The main difference between Redis and SQL databases is that Redis is an in-memory database, suitable for high performance and flexibility requirements; SQL database is a relational database, suitable for complex queries and data consistency requirements. Specifically, 1) Redis provides high-speed data access and caching services, supports multiple data types, suitable for caching and real-time data processing; 2) SQL database manages data through a table structure, supports complex queries and transaction processing, and is suitable for scenarios such as e-commerce and financial systems that require data consistency.
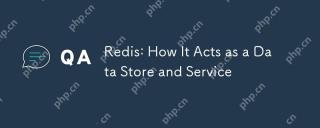
Redisactsasbothadatastoreandaservice.1)Asadatastore,itusesin-memorystorageforfastoperations,supportingvariousdatastructureslikekey-valuepairsandsortedsets.2)Asaservice,itprovidesfunctionalitieslikepub/submessagingandLuascriptingforcomplexoperationsan
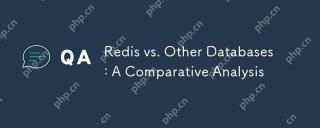
Compared with other databases, Redis has the following unique advantages: 1) extremely fast speed, and read and write operations are usually at the microsecond level; 2) supports rich data structures and operations; 3) flexible usage scenarios such as caches, counters and publish subscriptions. When choosing Redis or other databases, it depends on the specific needs and scenarios. Redis performs well in high-performance and low-latency applications.
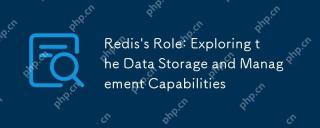
Redis plays a key role in data storage and management, and has become the core of modern applications through its multiple data structures and persistence mechanisms. 1) Redis supports data structures such as strings, lists, collections, ordered collections and hash tables, and is suitable for cache and complex business logic. 2) Through two persistence methods, RDB and AOF, Redis ensures reliable storage and rapid recovery of data.
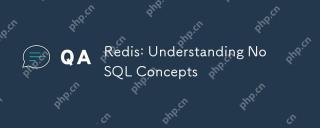
Redis is a NoSQL database suitable for efficient storage and access of large-scale data. 1.Redis is an open source memory data structure storage system that supports multiple data structures. 2. It provides extremely fast read and write speeds, suitable for caching, session management, etc. 3.Redis supports persistence and ensures data security through RDB and AOF. 4. Usage examples include basic key-value pair operations and advanced collection deduplication functions. 5. Common errors include connection problems, data type mismatch and memory overflow, so you need to pay attention to debugging. 6. Performance optimization suggestions include selecting the appropriate data structure and setting up memory elimination strategies.
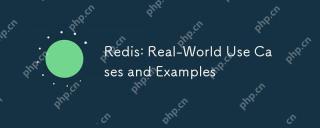
The applications of Redis in the real world include: 1. As a cache system, accelerate database query, 2. To store the session data of web applications, 3. To implement real-time rankings, 4. To simplify message delivery as a message queue. Redis's versatility and high performance make it shine in these scenarios.
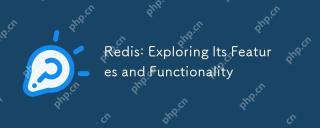
Redis stands out because of its high speed, versatility and rich data structure. 1) Redis supports data structures such as strings, lists, collections, hashs and ordered collections. 2) It stores data through memory and supports RDB and AOF persistence. 3) Starting from Redis 6.0, multi-threaded I/O operations have been introduced, which has improved performance in high concurrency scenarios.
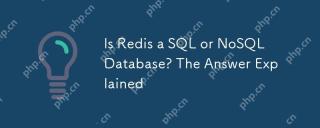
RedisisclassifiedasaNoSQLdatabasebecauseitusesakey-valuedatamodelinsteadofthetraditionalrelationaldatabasemodel.Itoffersspeedandflexibility,makingitidealforreal-timeapplicationsandcaching,butitmaynotbesuitableforscenariosrequiringstrictdataintegrityo


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
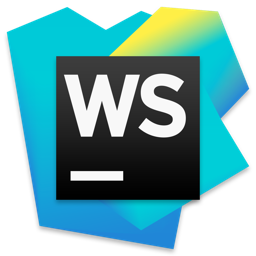
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
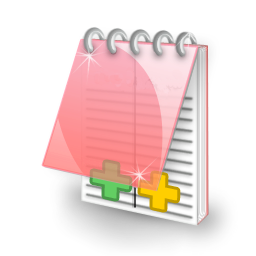
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Notepad++7.3.1
Easy-to-use and free code editor
