


Redis data structure operations in Golang development: how to store and retrieve data efficiently
Redis data structure operation in Golang development: how to store and retrieve data efficiently
Introduction:
Redis is a high-performance key-value database, which is widely used in cache, message queue, In scenarios such as rankings. As a high-performance programming language, Golang can achieve better performance when used with Redis. This article will introduce how to use Redis data structure operations in Golang development to achieve efficient storage and retrieval of data.
1. Connect to the Redis database
Before using Redis for data operations, we first need to connect to the Redis database. In Golang, you can use the "github.com/go-redis/redis" package to connect to Redis. The following is a sample code to connect to the Redis database:
package main import ( "fmt" "github.com/go-redis/redis" ) func main() { // 创建Redis客户端 client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", // Redis服务器地址和端口 Password: "", // 密码 DB: 0, // 数据库 }) // 测试连接 pong, err := client.Ping().Result() if err != nil { fmt.Println("连接Redis数据库失败:", err) return } fmt.Println("成功连接到Redis数据库:", pong) }
2. String data operation
- Storing data:
Use the Set command of Redis to save string data to Redis. In Golang, we can use the Set method provided by the Redis client. The following sample code shows how to store data into Redis:
package main import ( "fmt" "github.com/go-redis/redis" ) func main() { // 创建Redis客户端 client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", // Redis服务器地址和端口 Password: "", // 密码 DB: 0, // 数据库 }) // 存储数据 err := client.Set("name", "redis").Err() if err != nil { fmt.Println("存储数据失败:", err) return } fmt.Println("成功存储数据") }
- Retrieve data:
String data can be retrieved from Redis using the Redis Get command. In Golang, we can use the Get method provided by the Redis client. The following sample code shows how to retrieve data from Redis:
package main import ( "fmt" "github.com/go-redis/redis" ) func main() { // 创建Redis客户端 client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", // Redis服务器地址和端口 Password: "", // 密码 DB: 0, // 数据库 }) // 检索数据 val, err := client.Get("name").Result() if err != nil { fmt.Println("检索数据失败:", err) return } fmt.Println("检索到的数据为:", val) }
3. Hash data operation
- Store data:
Use Redis’ HSet command to Save key-value pair data into a hash table. In Golang, we can use the HSet method provided by the Redis client. The following sample code shows how to store data into a hash table:
package main import ( "fmt" "github.com/go-redis/redis" ) func main() { // 创建Redis客户端 client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", // Redis服务器地址和端口 Password: "", // 密码 DB: 0, // 数据库 }) // 存储数据 err := client.HSet("user", "name", "redis").Err() if err != nil { fmt.Println("存储数据失败:", err) return } fmt.Println("成功存储数据") }
- Retrieve data:
Use Redis's HGet command to retrieve the key corresponding to the hash table value. In Golang, we can use the HGet method provided by the Redis client. The following sample code shows how to retrieve data from a hash table:
package main import ( "fmt" "github.com/go-redis/redis" ) func main() { // 创建Redis客户端 client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", // Redis服务器地址和端口 Password: "", // 密码 DB: 0, // 数据库 }) // 检索数据 val, err := client.HGet("user", "name").Result() if err != nil { fmt.Println("检索数据失败:", err) return } fmt.Println("检索到的数据为:", val) }
Fourth, list data operation
- Store data:
Use the LPush command of Redis Data can be stored into lists. In Golang, we can use the LPush method provided by the Redis client. The following sample code shows how to store data into a list:
package main import ( "fmt" "github.com/go-redis/redis" ) func main() { // 创建Redis客户端 client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", // Redis服务器地址和端口 Password: "", // 密码 DB: 0, // 数据库 }) // 存储数据 err := client.LPush("list", "data1", "data2", "data3").Err() if err != nil { fmt.Println("存储数据失败:", err) return } fmt.Println("成功存储数据") }
- Retrieve data:
Use Redis's LRange command to retrieve a specified range of data from a list. In Golang, we can use the LRange method provided by the Redis client. The following sample code shows how to retrieve data from a list:
package main import ( "fmt" "github.com/go-redis/redis" ) func main() { // 创建Redis客户端 client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", // Redis服务器地址和端口 Password: "", // 密码 DB: 0, // 数据库 }) // 检索数据 vals, err := client.LRange("list", 0, -1).Result() if err != nil { fmt.Println("检索数据失败:", err) return } fmt.Println("检索到的数据为:", vals) }
Summary:
This article introduces how to use the Redis data structure operation in Golang development to achieve efficient storage and retrieval of data. By using the Go-Redis package, we can easily connect to the Redis database and implement storage and retrieval of data types such as strings, hashes, and lists. I hope this article will be helpful to your learning and development.
The above is the detailed content of Redis data structure operations in Golang development: how to store and retrieve data efficiently. For more information, please follow other related articles on the PHP Chinese website!
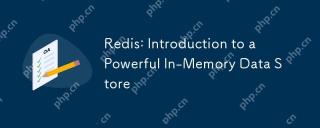
Redisisahigh-performancein-memorydatastructurestorethatexcelsinspeedandversatility.1)Itsupportsvariousdatastructureslikestrings,lists,andsets.2)Redisisanin-memorydatabasewithpersistenceoptions,ensuringfastperformanceanddatasafety.3)Itoffersatomicoper
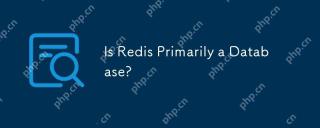
Redis is primarily a database, but it is more than just a database. 1. As a database, Redis supports persistence and is suitable for high-performance needs. 2. As a cache, Redis improves application response speed. 3. As a message broker, Redis supports publish-subscribe mode, suitable for real-time communication.
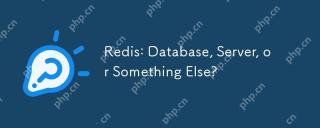
Redisisamultifacetedtoolthatservesasadatabase,server,andmore.Itfunctionsasanin-memorydatastructurestore,supportsvariousdatastructures,andcanbeusedasacache,messagebroker,sessionstorage,andfordistributedlocking.
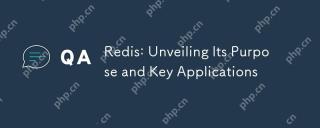
Redisisanopen-source,in-memorydatastructurestoreusedasadatabase,cache,andmessagebroker,excellinginspeedandversatility.Itiswidelyusedforcaching,real-timeanalytics,sessionmanagement,andleaderboardsduetoitssupportforvariousdatastructuresandfastdataacces
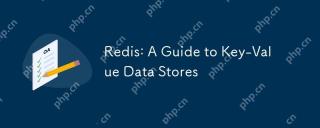
Redis is an open source memory data structure storage used as a database, cache and message broker, suitable for scenarios where fast response and high concurrency are required. 1.Redis uses memory to store data and provides microsecond read and write speed. 2. It supports a variety of data structures, such as strings, lists, collections, etc. 3. Redis realizes data persistence through RDB and AOF mechanisms. 4. Use single-threaded model and multiplexing technology to handle requests efficiently. 5. Performance optimization strategies include LRU algorithm and cluster mode.
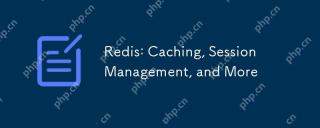
Redis's functions mainly include cache, session management and other functions: 1) The cache function stores data through memory to improve reading speed, and is suitable for high-frequency access scenarios such as e-commerce websites; 2) The session management function shares session data in a distributed system and automatically cleans it through an expiration time mechanism; 3) Other functions such as publish-subscribe mode, distributed locks and counters, suitable for real-time message push and multi-threaded systems and other scenarios.
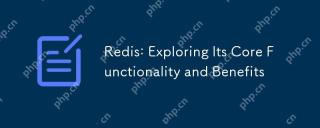
Redis's core functions include memory storage and persistence mechanisms. 1) Memory storage provides extremely fast read and write speeds, suitable for high-performance applications. 2) Persistence ensures that data is not lost through RDB and AOF, and the choice is based on application needs.
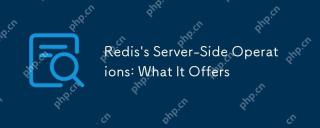
Redis'sServer-SideOperationsofferFunctionsandTriggersforexecutingcomplexoperationsontheserver.1)FunctionsallowcustomoperationsinLua,JavaScript,orRedis'sscriptinglanguage,enhancingscalabilityandmaintenance.2)Triggersenableautomaticfunctionexecutionone


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Notepad++7.3.1
Easy-to-use and free code editor
