


How to use the ftplib module for FTP client programming in Python 2.x
In Python, we can use the ftplib module to create an FTP client to facilitate interaction with the remote FTP server. Through this module, we can implement functions such as uploading files, downloading files, and deleting files. This article will introduce in detail how to use the ftplib module for FTP client programming, with code examples.
First, we need to introduce the ftplib module:
from ftplib import FTP
Then, we can use the FTP class to create an FTP object and connect to the remote server:
ftp = FTP() ftp.connect('ftp.example.com', 21)
Where, ' ftp.example.com' is the hostname of the target server, and 21 is the default port number of the FTP server.
Next, we need to log in to the FTP server. If you need login permission, you can use the login() method for authentication:
ftp.login('username', 'password')
where 'username' is the username and 'password' is the password. If authentication is not required, you can use anonymous login:
ftp.login()
After successful login, we can start FTP operations. The following are some commonly used FTP operations:
-
Uploading files
with open('example.txt', 'rb') as file: ftp.storbinary('STOR example.txt', file)
Among them, 'example.txt' is the path to the local file, and 'STOR example.txt' is the path to the local file. The path to upload to the server.
-
Download file
with open('example.txt', 'wb') as file: ftp.retrbinary('RETR example.txt', file.write)
Among them, 'example.txt' is the path of the file to be downloaded on the server, and 'file.write' means writing the file content to a local file.
-
Delete files
ftp.delete('example.txt')
Where, 'example.txt' is the path of the file to be deleted on the server.
-
Create directory
ftp.mkd('new_directory')
Where, 'new_directory' is the name of the directory to be created.
-
Switch directory
ftp.cwd('directory')
Where, 'directory' is the name of the directory to be switched to.
-
List directory contents
print ftp.nlst()
This method will return a list of files and folders in the directory.
After completing the FTP operation, we can use the quit() method to close the FTP connection:
ftp.quit()
The following is a complete FTP client programming example:
from ftplib import FTP def ftp_client(): ftp = FTP() ftp.connect('ftp.example.com', 21) ftp.login('username', 'password') # 上传文件 with open('example.txt', 'rb') as file: ftp.storbinary('STOR example.txt', file) # 下载文件 with open('example.txt', 'wb') as file: ftp.retrbinary('RETR example.txt', file.write) # 删除文件 ftp.delete('example.txt') # 创建目录 ftp.mkd('new_directory') # 切换目录 ftp.cwd('directory') # 列出目录内容 print ftp.nlst() ftp.quit() if __name__ == '__main__': ftp_client()
With the above code example, we can use the ftplib module for FTP client programming in Python 2.x. According to actual needs, we can flexibly use the above FTP operation methods to achieve the required FTP functions.
The above is the detailed content of How to use the ftplib module for FTP client programming in Python 2.x. For more information, please follow other related articles on the PHP Chinese website!
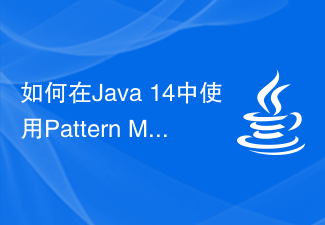
如何在Java14中使用PatternMatching进行类型模式匹配引言:Java14引入了一种新的特性,即PatternMatching,这是一种强大的工具,可用于在编译时进行类型模式匹配。本文将介绍如何在Java14中使用PatternMatching进行类型模式匹配,并提供代码示例。理解PatternMatching的概念Pattern
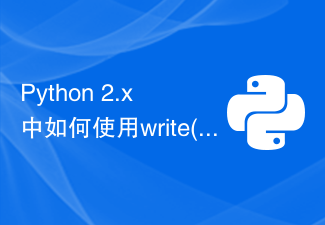
Python2.x中如何使用write()函数向文件写入内容在Python2.x中,我们可以使用write()函数将内容写入文件中。write()函数是file对象的方法之一,可用于向文件中写入字符串或二进制数据。在本文中,我将详细介绍如何使用write()函数以及一些常见的使用案例。打开文件在使用write()函数写入文件之前,我
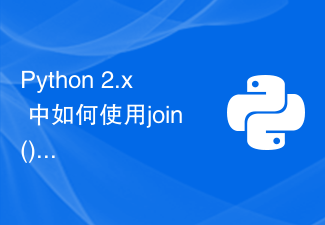
Python2.x中如何使用join()函数将字符串列表合并为一个字符串在Python中,我们经常需要将多个字符串合并成一个字符串。Python提供了多种方式来实现这个目标,其中一种常用的方式是使用join()函数。join()函数可以将一个字符串列表拼接成一个字符串,并且可以指定拼接时的分隔符。使用join()函数的基本语法如下:&
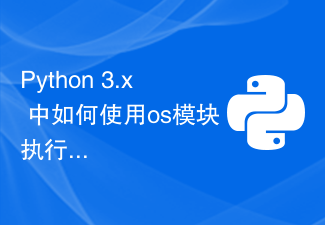
Python3.x中如何使用os模块执行系统命令在Python3.x的标准库中,os模块提供了一系列方法,用于执行系统命令。在本文中,我们将学习如何使用os模块来执行系统命令,并给出相应的代码示例。Python中的os模块是与操作系统进行交互的一个接口。它提供了一些方法,例如执行系统命令、访问文件和目录等。下面是一些常用的os模块方法,可以在执行系统命
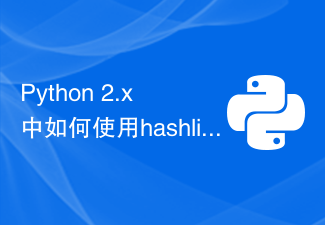
Python2.x中如何使用hashlib模块进行哈希算法计算在Python编程中,哈希算法是一种常用的算法,用于生成数据的唯一标识。Python提供了hashlib模块来进行哈希算法的计算。本文将介绍如何使用hashlib模块进行哈希算法计算,并给出一些示例代码。hashlib模块是Python标准库中的一部分,提供了多种常见的哈希算法,如MD5、SH
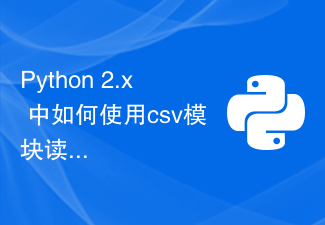
Python2.x中如何使用csv模块读取和写入CSV文件导言:CSV(CommaSeparatedValues)是一种常见的文件格式,用于存储和交换数据。Python的csv模块提供了一种简单的方式来读取和写入CSV文件。本文将介绍如何使用csv模块在Python2.x中读取和写入CSV文件,并提供相应的代码示例。一、读取CSV文件要读取CSV文
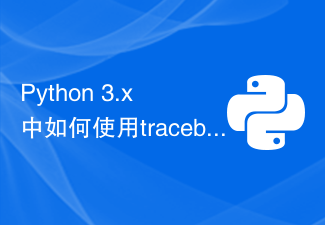
Python3.x中如何使用traceback模块进行异常跟踪引言:在编写和调试Python程序时,我们经常会遇到各种异常。异常是程序在运行过程中发生的错误,为了更好地定位和解决问题,我们需要了解异常发生的上下文信息。Python提供了traceback模块,它可以帮助我们获取异常的相关信息,并进行异常跟踪。本文将介绍如何在Python
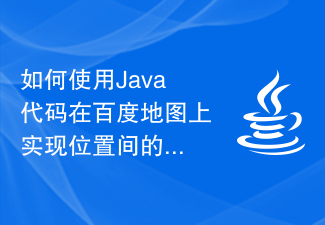
如何使用Java代码在百度地图上实现位置间的地理编码和逆地理编码?在开发地理位置相关的应用程序时,常常需要进行地理编码和逆地理编码的操作。百度地图提供了丰富的API来满足这个需求。本文将介绍如何使用Java代码来实现百度地图上的地理编码和逆地理编码。首先,我们需要通过百度地图开放平台获取一个API密钥。在申请完成后,我们就可以使用该密钥来访问地理编码和逆地理


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
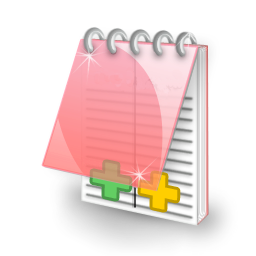
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
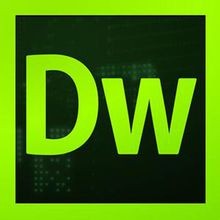
Dreamweaver CS6
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
