


How to use Java code to display multiple markers on the map and implement functions based on click events?
How to use Java code to display multiple markers on the map and implement functions based on click events?
Map applications have become an indispensable part of our lives. They not only help us find destinations, but also display information about attractions, restaurants and other information around us. When developing map applications, we often need to display multiple marker points on the map, and implement corresponding functions based on the user's click events, such as displaying specific information about the marker point. Below, we will use Java code to implement this functionality.
First, we need to import map-related libraries, such as Google Maps API. Add the corresponding library to the project's dependency configuration.
Next, we need to create a map container to display the map. This can be achieved using Swing or JavaFX. Here we take Swing as an example to demonstrate.
import javax.swing.*; import java.awt.*; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; public class MapApplication extends JFrame { private JPanel mapPanel; public MapApplication() { setTitle("地图应用"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setLayout(new BorderLayout()); // 创建地图容器 mapPanel = new JPanel(); mapPanel.setPreferredSize(new Dimension(800, 600)); add(mapPanel, BorderLayout.CENTER); // 绑定鼠标点击事件 mapPanel.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { // 获取鼠标点击的坐标 double latitude = convertYToLatitude(e.getY()); double longitude = convertXToLongitude(e.getX()); // 根据坐标显示标记点 addMarker(latitude, longitude); } }); pack(); setVisible(true); } private double convertYToLatitude(int y) { // 省略坐标转换逻辑 return 0.0; } private double convertXToLongitude(int x) { // 省略坐标转换逻辑 return 0.0; } private void addMarker(double latitude, double longitude) { // 在地图上显示标记点 // 省略具体的标记点显示逻辑 } public static void main(String[] args) { SwingUtilities.invokeLater(() -> { new MapApplication(); }); } }
In the above code, we created a MapApplication
class, inherited from JFrame
, and completed the initialization of the interface in the constructor. We created a JPanel
objectmapPanel
as the map container and set it in the center of the window using the BorderLayout
layout. Then, we bound the mouse click event of mapPanel
. When the user clicks on the map, the event is triggered, and the coordinates of the mouse click are obtained in the event processing method and converted into geographical coordinates. Finally, we use the addMarker
method to display the marker points on the map.
In actual development, we need to make corresponding adjustments based on specific map API and functional requirements. The above is just a simple example to help you understand how to use Java code to display multiple markers on the map and implement functions based on click events. Hope this helps!
The above is the detailed content of How to use Java code to display multiple markers on the map and implement functions based on click events?. For more information, please follow other related articles on the PHP Chinese website!
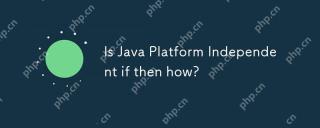
Java is platform-independent because of its "write once, run everywhere" design philosophy, which relies on Java virtual machines (JVMs) and bytecode. 1) Java code is compiled into bytecode, interpreted by the JVM or compiled on the fly locally. 2) Pay attention to library dependencies, performance differences and environment configuration. 3) Using standard libraries, cross-platform testing and version management is the best practice to ensure platform independence.
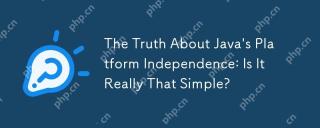
Java'splatformindependenceisnotsimple;itinvolvescomplexities.1)JVMcompatibilitymustbeensuredacrossplatforms.2)Nativelibrariesandsystemcallsneedcarefulhandling.3)Dependenciesandlibrariesrequirecross-platformcompatibility.4)Performanceoptimizationacros
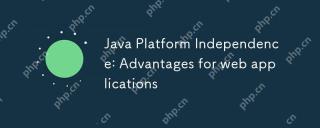
Java'splatformindependencebenefitswebapplicationsbyallowingcodetorunonanysystemwithaJVM,simplifyingdeploymentandscaling.Itenables:1)easydeploymentacrossdifferentservers,2)seamlessscalingacrosscloudplatforms,and3)consistentdevelopmenttodeploymentproce
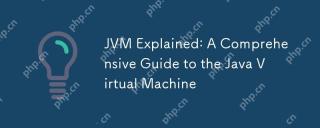
TheJVMistheruntimeenvironmentforexecutingJavabytecode,crucialforJava's"writeonce,runanywhere"capability.Itmanagesmemory,executesthreads,andensuressecurity,makingitessentialforJavadeveloperstounderstandforefficientandrobustapplicationdevelop
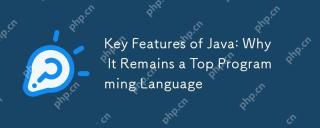
Javaremainsatopchoicefordevelopersduetoitsplatformindependence,object-orienteddesign,strongtyping,automaticmemorymanagement,andcomprehensivestandardlibrary.ThesefeaturesmakeJavaversatileandpowerful,suitableforawiderangeofapplications,despitesomechall
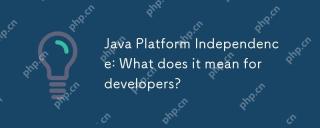
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
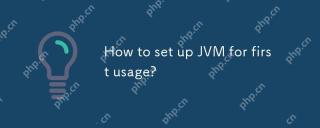
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
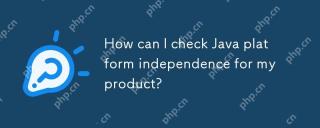
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
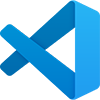
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor
