


The Stream API was introduced in Java 8, providing a new way to operate data collections. The stream API allows us to operate on collections in a simpler and more flexible way, in which the map() method is a commonly used conversion operation.
Before Java 8, when we convert a collection, we usually need to use a for loop or iterator to traverse the elements in the collection and then process them one by one. Using the map() method of the stream allows us to convert the collection more conveniently, simplifying the operation process.
First, let’s understand the definition of the map() method. The map() method is an intermediate operation in the stream API. It accepts a function as a parameter. This function will be applied to each element in the stream and map it into a new element. Finally, the map() method returns the stream containing the new elements.
The following is a sample code that uses the map() method to convert a collection:
import java.util.ArrayList; import java.util.List; import java.util.stream.Collectors; public class MapExample { public static void main(String[] args) { List<Integer> numbers = new ArrayList<>(); numbers.add(1); numbers.add(2); numbers.add(3); numbers.add(4); numbers.add(5); List<Integer> squaredNumbers = numbers.stream() .map(n -> n * n) .collect(Collectors.toList()); System.out.println("原始集合: " + numbers); System.out.println("转换后的集合: " + squaredNumbers); } }
In the above sample code, we first create a collection numbers containing integers. Then, we call the stream() method to convert it to a stream. Next, we call the map() method and pass a function (n -> n * n) as a parameter. What this function does is map each element n in the stream to n squared. Finally, we use the collect() method to collect the converted elements into a new list.
Run the above code, the output is as follows:
原始集合: [1, 2, 3, 4, 5] 转换后的集合: [1, 4, 9, 16, 25]
You can see that each element in the original collection has been squared, and the new elements are collected into a new list .
In addition to the above examples, we can also use the map() method to implement more complex conversion operations. For example, you can convert all strings in a string collection to uppercase, or extract a certain attribute of an object in a collection to form a new collection, etc. The map() method can convert elements according to the function logic we pass, and is very flexible.
In summary, the stream API in Java 8 provides a convenient way to perform conversion operations on collections. The map() method is one of its core methods. It can map the elements in the stream to new elements according to the function we pass, and return the stream containing the new elements. By using the map() method, we can convert collections more concisely and flexibly, improving the readability and maintainability of the code.
The above is the detailed content of Stream API in Java 8: How to use map() method to perform transformation operations on collections. For more information, please follow other related articles on the PHP Chinese website!
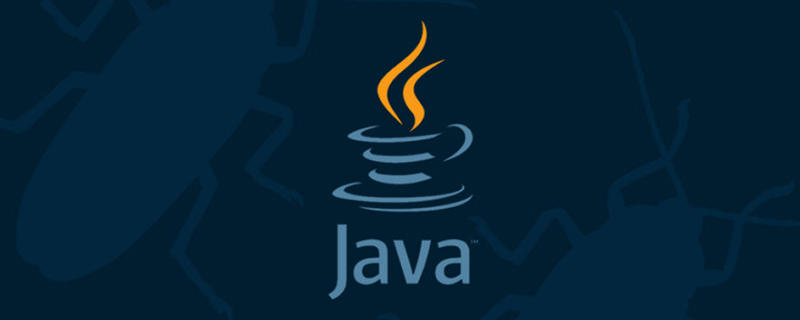
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
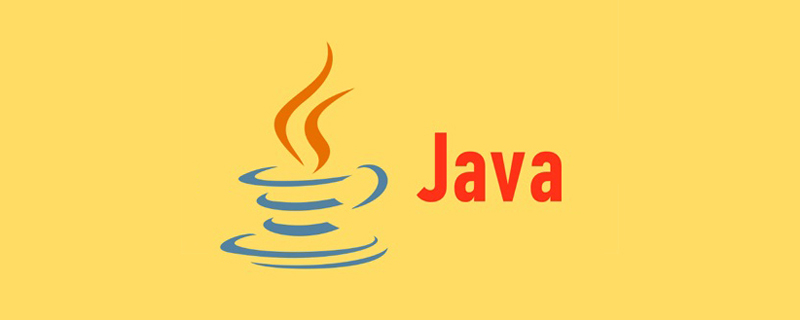
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
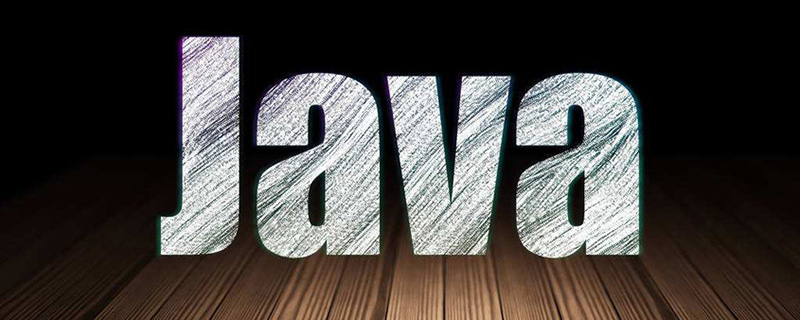
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
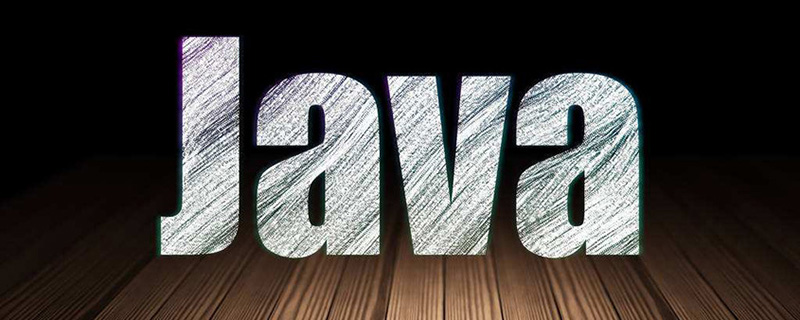
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
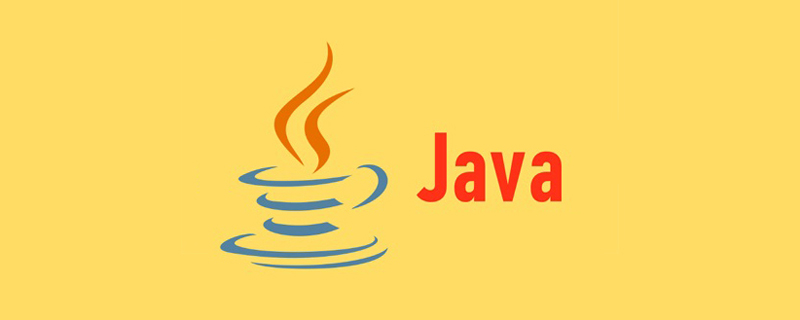
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
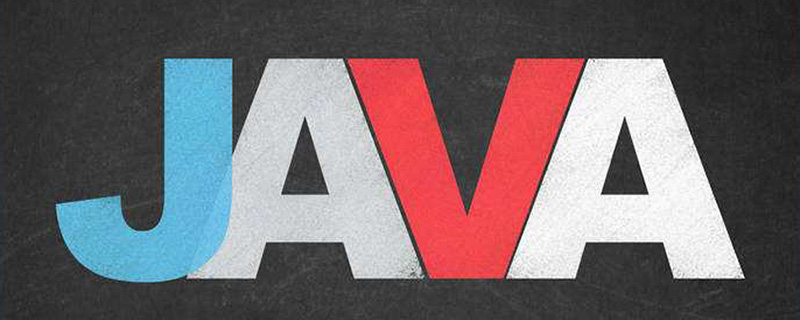
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
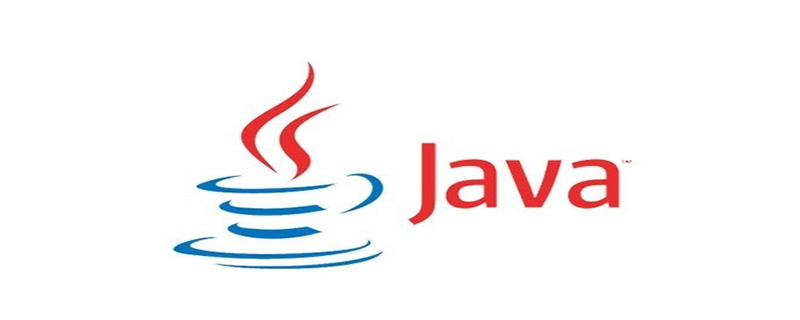
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。
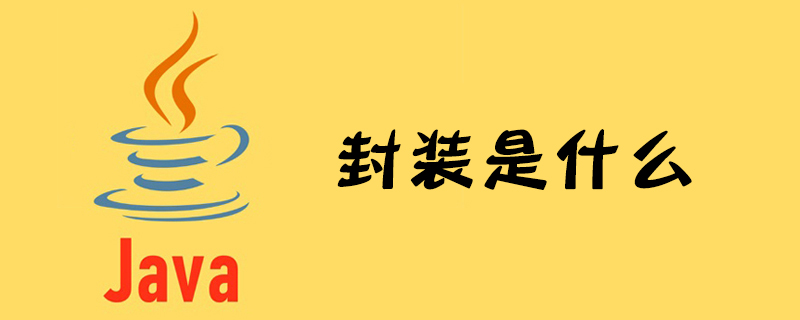
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
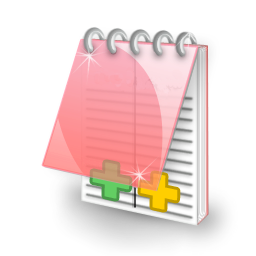
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
