Multiple database operations between Redis and PHP: How to implement data partitioning
Redis is a fast, high-performance key-value storage database that is often used to cache data and handle high-concurrency operations. In practical applications, we often need to process large amounts of data, and a single Redis database may not be able to meet our needs. Therefore, using multiple databases for data partitioning is a very common solution. This article will introduce how to perform multi-database operations through PHP and Redis to achieve data partitioning.
1. Introduction to Redis multi-database
Redis supports data distribution in multiple databases. By default, Redis creates 16 databases, numbered from 0 to 15. We can switch databases through the select command, as shown below:
$redis = new Redis(); $redis->connect('127.0.0.1', 6379); $redis->select(1); // 切换到数据库1
After switching the database through the select command, subsequent operations will be performed in the currently selected database.
2. Use hash functions to implement data partitioning
In practical applications, we may need to store data dispersedly in multiple databases, and we can use hash functions to partition the keys of the data. , so that it is evenly distributed in different databases.
The following is a simple example that demonstrates how to implement data partitioning through hash functions:
function getDatabaseIndex($key, $totalDatabases) { $hash = crc32($key); $databaseIndex = $hash % $totalDatabases; return $databaseIndex; } $redis = new Redis(); $redis->connect('127.0.0.1', 6379); $key = 'user:123'; $totalDatabases = 8; $databaseIndex = getDatabaseIndex($key, $totalDatabases); $redis->select($databaseIndex); // 切换到计算得到的数据库 // 对数据进行操作 $redis->set($key, 'value'); $value = $redis->get($key);
With the above code, we can calculate the database where the data should be stored based on the hash value of the key number, and use the select command to switch to the corresponding database for operation.
3. Use Redis Cluster to implement data partitioning
In addition to using hash functions for data partitioning, Redis also provides the Redis Cluster function, which can implement data partitioning and partitioning between different nodes. Load balancing.
The following is a sample code that demonstrates how to use Redis Cluster to implement data partitioning:
$redis = new RedisCluster(NULL, [ '127.0.0.1:7000', '127.0.0.1:7001', '127.0.0.1:7002', '127.0.0.1:7003', '127.0.0.1:7004', '127.0.0.1:7005', ]); // 对数据进行操作 $redis->set('key', 'value'); $value = $redis->get('key');
In the above code, we connect to the Redis cluster through the RedisCluster class and perform data read and write operations. Redis Cluster will automatically partition data to different nodes to achieve data load balancing.
4. Summary
Through the multi-database operation of Redis and PHP, we can realize partition storage of data to better meet the needs of practical applications. We can disperse and store data in multiple databases through hash functions, or we can use Redis Cluster to achieve data partitioning and load balancing. In practical applications, suitable solutions can be selected according to specific needs.
The above is an introduction to the multi-database operation of Redis and PHP: how to implement data partitioning. I hope it will be helpful to you.
The above is the detailed content of Multi-database operations with Redis and PHP: how to implement data partitioning. For more information, please follow other related articles on the PHP Chinese website!
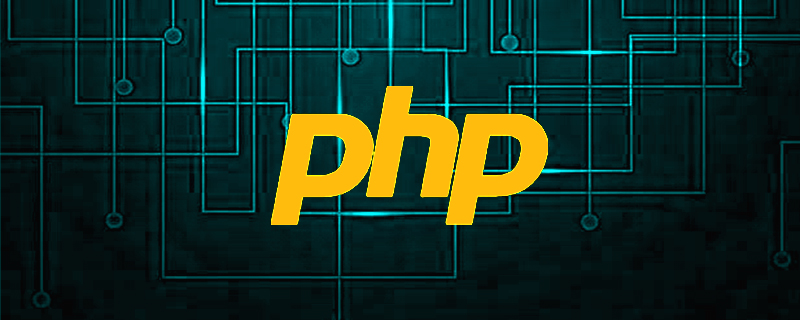
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
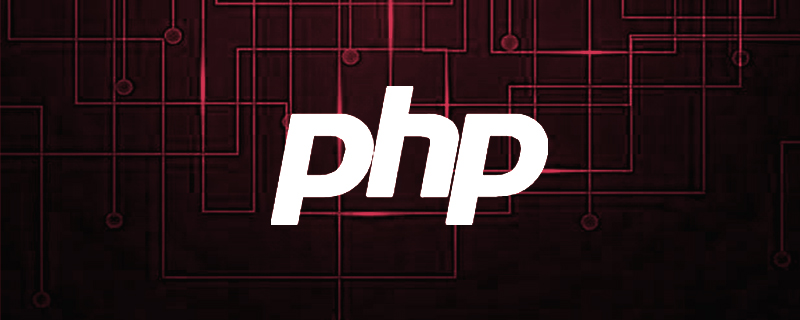
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
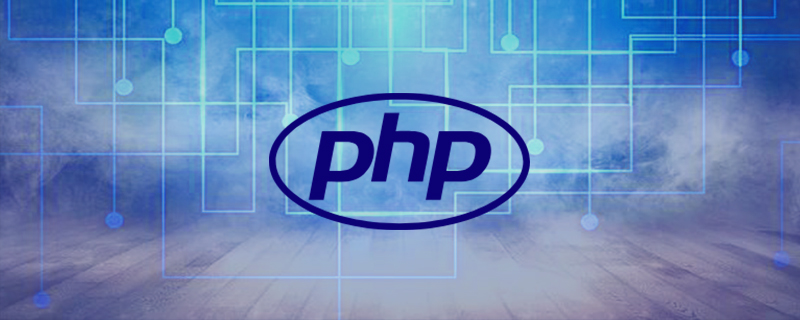
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
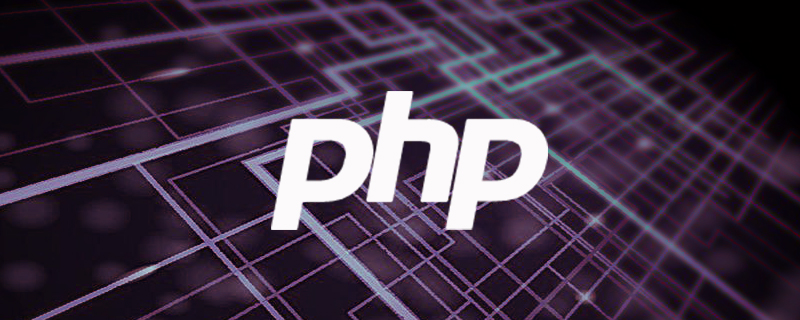
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
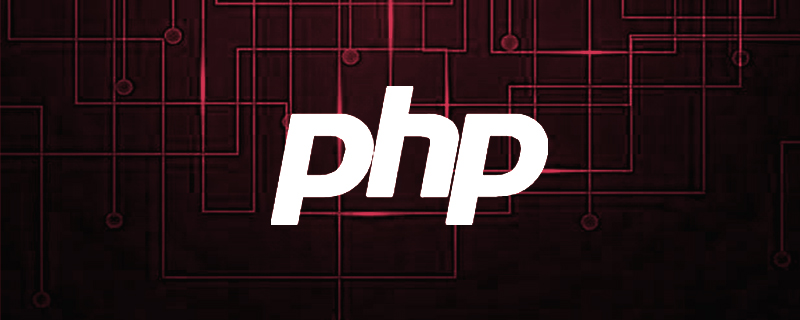
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
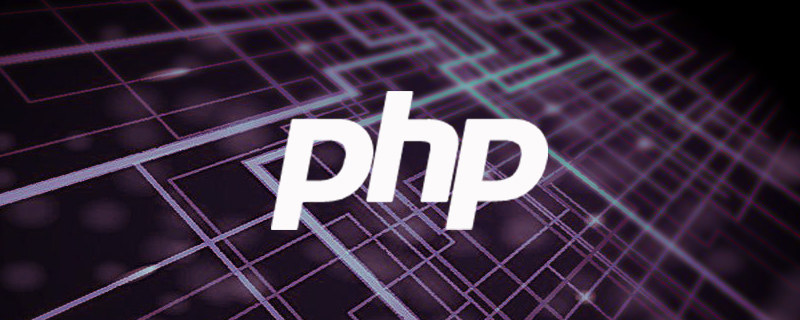
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
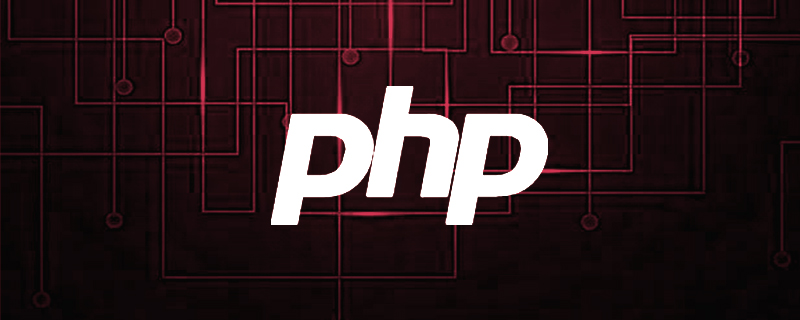
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
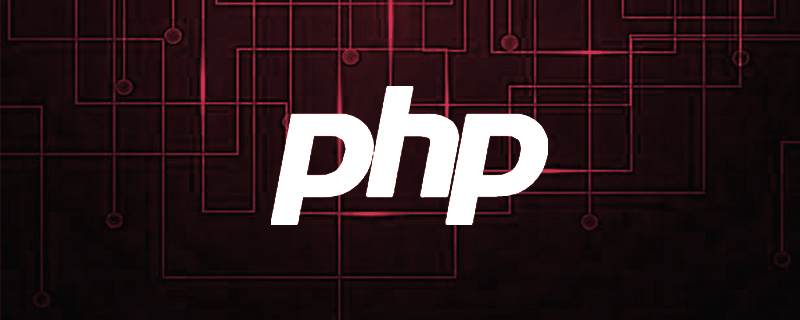
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
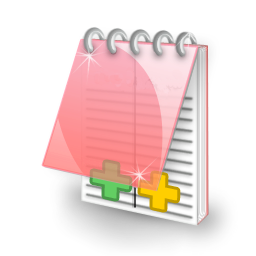
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
