


CodeIgniter middleware: implementing flexible URL forwarding and redirection
CodeIgniter Middleware: Implementing Flexible URL Forwarding and Redirection
As a popular PHP framework, CodeIgniter provides many features and tools to simplify the web application development process. One of the important functions is middleware, which can implement flexible URL forwarding and redirection, providing developers with better control and customization capabilities. This article will introduce how to use CodeIgniter middleware, with code examples to help readers understand it in depth.
- Initialize middleware
First, before using middleware, we need to initialize and configure related settings. Please add the following code in CodeIgniter’s configuration file (config.php):
$config['middleware'] = [ 'enabled' => true, 'route_group' => 'api', // 设置中间件所属的路由组标识符 'middlewares' => [ 'auth' => 'AuthMiddleware', // 配置中间件名称和对应的类名 'logger' => 'LoggerMiddleware' ] ];
In the above example, we enabled the middleware and specified the routing group to which they belong. At the same time, we also defined two middleware: "auth" and "logger", and specified their corresponding class names.
- Create the middleware class
Next, we need to create the middleware class. Please create two files in the "App/Middlewares" directory of CodeIgniter: AuthMiddleware.php and LoggerMiddleware.php. Here is the sample code:
AuthMiddleware.php:
<?php namespace AppMiddlewares; class AuthMiddleware { public function handle($request, $response, $next) { // 实现验证逻辑 if (!$this->isAuthenticated()) { redirect('login'); // 重定向到登录页面 } $response = $next($request, $response); return $response; } private function isAuthenticated() { // 实现验证逻辑 return check_login_status(); } }
LoggerMiddleware.php:
<?php namespace AppMiddlewares; class LoggerMiddleware { public function handle($request, $response, $next) { // 记录日志 $this->logRequest($request); $response = $next($request, $response); return $response; } private function logRequest($request) { // 实现日志记录逻辑 // ... } }
In the above example, we have created two middleware classes. They all contain a handle method that receives the request and response objects, and a closure function $next. In the handle method, we can perform some specific logic, such as verifying that the user is logged in (AuthMiddleware) or logging each request (LoggerMiddleware). Finally, we call the $next closure function passing the request and response objects to continue processing subsequent middleware or controllers.
- Register middleware
Next, we need to register the middleware in CodeIgniter’s routing file (Routes.php). Please add the following code to your Routes.php file:
// 使用中间件的路由组 $routes->group('api', ['middleware' => ['auth', 'logger']], function ($routes) { // 定义相关路由 $routes->get('dashboard', 'AdminController::dashboard'); $routes->post('users', 'UserController::create'); });
In the above example, we used the route group "api" of the middleware and specified the middleware to be applied ('auth' and 'logger'). Next, we define two specific routes and specify their corresponding controller methods. Routing groups using middleware ensure that the middleware's logic is executed before a specific route is executed.
- Using Middleware
Now, we can use middleware to forward and redirect URLs. The following example code shows how to use middleware in a controller:
<?php namespace AppControllers; use CodeIgniterController; class AdminController extends Controller { public function __construct() { helper('url'); } public function dashboard() { // 执行其他逻辑... // 转发到其他路由 return redirect()->to('api/users'); // 或者重定向到其他URL // return redirect()->to('https://example.com'); } }
In the above example, we use the redirect function in the dashboard method of AdminController to forward to other routes ('api/users' ), or redirect to another URL ('https://example.com').
Through the above steps, we successfully implemented the flexible URL forwarding and redirection functions of CodeIgniter middleware. Middleware allows us to have more control over request and response objects and perform some custom logic before processing a specific route. This provides developers with greater flexibility and customization capabilities to meet different application needs.
Summary
Middleware is a powerful feature in CodeIgniter, which makes URL forwarding and redirection more flexible and customizable. Through middleware, we can perform some custom logic before processing a specific route, such as verifying that the user is logged in or logging each request. With the above steps and code examples, we can easily start using middleware in CodeIgniter and add more functionality and flexibility to our web applications.
The above is the detailed content of CodeIgniter middleware: implementing flexible URL forwarding and redirection. For more information, please follow other related articles on the PHP Chinese website!
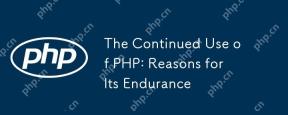
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
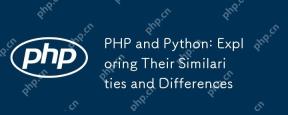
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
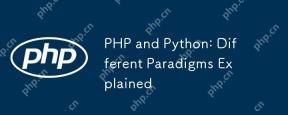
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
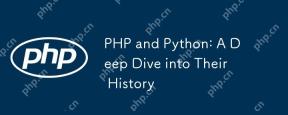
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
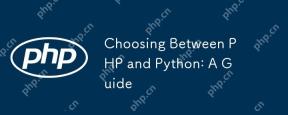
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
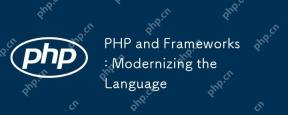
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
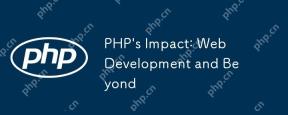
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
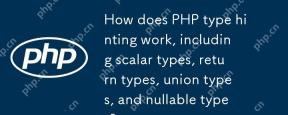
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
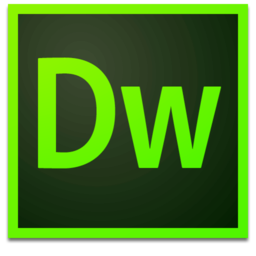
Dreamweaver Mac version
Visual web development tools
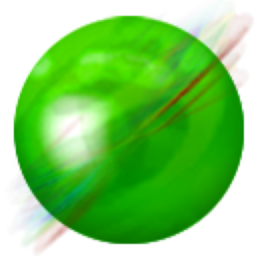
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
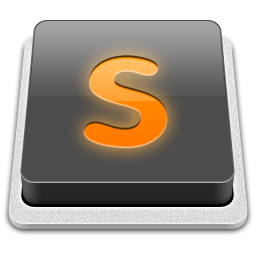
SublimeText3 Mac version
God-level code editing software (SublimeText3)