Implementing PHP security verification using Keycloak
Using Keycloak to implement PHP security authentication
Introduction:
As web applications grow and diversify, protecting user data and identities has become critical. In order to achieve secure authentication, we often need to implement authentication and authorization schemes based on standards such as OAuth and OpenID Connect. Keycloak is an open source identity and access management solution that provides unified authentication and authorization services. This article will introduce how to use Keycloak to implement PHP-based security authentication and provide corresponding code examples.
1. Set up Keycloak server
First, we need to set up Keycloak on the server. You can download the corresponding installation package through Keycloak's official website (https://www.keycloak.org/), and follow the steps in the official documentation to install and configure it.
2. Create Keycloak client
On the Keycloak management interface, create a new Realm (realm), and then create a new client (Client) under the Realm. In the client configuration, configure the correct redirect URI and valid client credentials, and optionally other related options.
3. Install the OAuth client library
In order to use Keycloak's authentication and authorization mechanism in PHP projects, we can use some third-party OAuth client libraries. In this article, we will use the "OAuth 2.0 Client" open source library (https://github.com/thephpleague/oauth2-client).
First, use Composer to install the library:
composer require league/oauth2-client
After the installation is complete, we can start writing PHP code.
4. PHP code example
In the PHP code, we need to configure Keycloak's client credentials and redirect URI. Then, we can implement identity authentication and authorization verification by obtaining Keycloak's authorization code, token, and user information.
// 引入 OAuth 2.0 Client 库 require_once('vendor/autoload.php'); use LeagueOAuth2ClientProviderKeycloak; // Keycloak 客户端凭证和重定向 URI $clientId = 'YOUR_CLIENT_ID'; $clientSecret = 'YOUR_CLIENT_SECRET'; $redirectUri = 'http://localhost/callback.php'; // 创建 Keycloak 提供程序 $provider = new Keycloak([ 'authServerUrl' => 'http://localhost:8080/auth', 'realm' => 'YOUR_REALM', 'clientId' => $clientId, 'clientSecret' => $clientSecret, 'redirectUri' => $redirectUri ]); // 获取授权码 if (!isset($_GET['code'])) { $authUrl = $provider->getAuthorizationUrl(); $_SESSION['oauth2state'] = $provider->getState(); header('Location: ' . $authUrl); exit; } // 验证授权码 if (empty($_GET['state']) || ($_GET['state'] !== $_SESSION['oauth2state'])) { unset($_SESSION['oauth2state']); exit('Invalid state'); } // 授权码授权 $token = $provider->getAccessToken('authorization_code', [ 'code' => $_GET['code'] ]); // 使用令牌获取用户信息 $user = $provider->getResourceOwner($token); // 打印用户信息 echo 'Logged in as ' . $user->getName(); // 使用令牌调用 Keycloak API $response = $provider->getHttpClient()->get( 'http://localhost:8080/auth/realms/YOUR_REALM/protocol/openid-connect/userinfo', [ 'headers' => [ 'Authorization' => 'Bearer ' . $token->getToken() ] ] ); $data = json_decode($response->getBody(), true); // 打印用户信息 print_r($data);
Code Description:
- First, we introduce the OAuth 2.0 Client library and create the Keycloak provider.
- Then, we determine whether the authorization code has been obtained, and if not, redirect to the Keycloak authentication page.
- After obtaining the authorization code, we verify the validity of the authorization code and obtain the token through the authorization code.
- Use the token to obtain user information and perform corresponding operations.
5. Summary
Using Keycloak to implement PHP security verification is a safe and reliable way. By configuring the Keycloak client and combining it with the OAuth 2.0 Client library, we can easily implement Keycloak-based authentication and authorization verification.
The above is a basic example, you can extend and customize it according to your own needs. I hope this article has been helpful in understanding Keycloak and PHP security validation.
Reference link:
- Keycloak official website: https://www.keycloak.org/
- OAuth 2.0 Client open source library: https://github. com/thephpleague/oauth2-client
The above is the detailed content of Implementing PHP security verification using Keycloak. For more information, please follow other related articles on the PHP Chinese website!
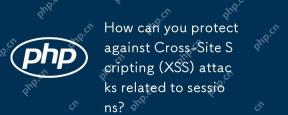
To protect the application from session-related XSS attacks, the following measures are required: 1. Set the HttpOnly and Secure flags to protect the session cookies. 2. Export codes for all user inputs. 3. Implement content security policy (CSP) to limit script sources. Through these policies, session-related XSS attacks can be effectively protected and user data can be ensured.
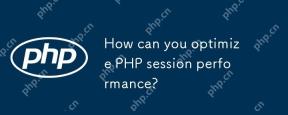
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
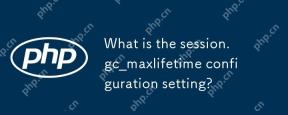
Thesession.gc_maxlifetimesettinginPHPdeterminesthelifespanofsessiondata,setinseconds.1)It'sconfiguredinphp.iniorviaini_set().2)Abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3)PHP'sgarbagecollectionisprobabilistic,influencedbygc_probabi

In PHP, you can use the session_name() function to configure the session name. The specific steps are as follows: 1. Use the session_name() function to set the session name, such as session_name("my_session"). 2. After setting the session name, call session_start() to start the session. Configuring session names can avoid session data conflicts between multiple applications and enhance security, but pay attention to the uniqueness, security, length and setting timing of session names.
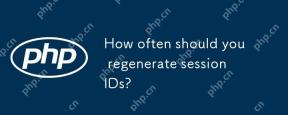
The session ID should be regenerated regularly at login, before sensitive operations, and every 30 minutes. 1. Regenerate the session ID when logging in to prevent session fixed attacks. 2. Regenerate before sensitive operations to improve safety. 3. Regular regeneration reduces long-term utilization risks, but the user experience needs to be weighed.
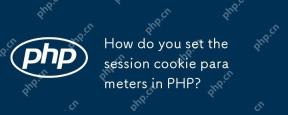
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
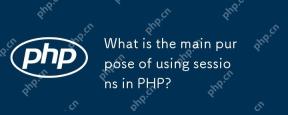
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
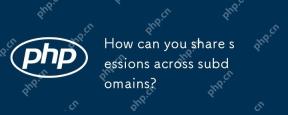
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
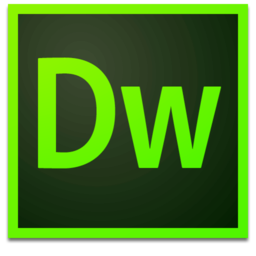
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
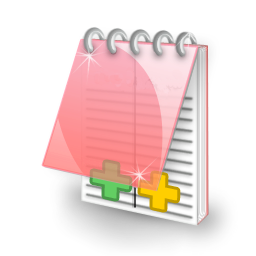
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function