


Convert multidimensional array to string using java's Arrays.deepToString() function
Use Java's Arrays.deepToString() function to convert multidimensional arrays into strings
In Java programming, we often encounter situations where we need to convert multidimensional arrays into strings. Java provides the Arrays.deepToString() function, which can easily convert multi-dimensional arrays into string form. This article will introduce how to use the Arrays.deepToString() function, with code examples.
The Arrays.deepToString() function is a static method in the Java.util.Arrays class, which can convert multi-dimensional arrays into string form. This method can handle arrays of any dimension and is suitable for two-dimensional arrays, three-dimensional arrays and even higher-dimensional arrays.
The following is the method signature of the Arrays.deepToString() function:
public static String deepToString(Object[] a)
This function accepts an array of type Object as a parameter , and returns a string. It will recursively iterate through each element in the array, convert each element to a string using the toString() method, and finally concatenate it into a comma-separated string form.
The following is a simple example that demonstrates how to use the Arrays.deepToString() function to convert a two-dimensional array into a string:
public class Main { public static void main(String[] args) { int[][] matrix = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}; String matrixString = Arrays.deepToString(matrix); System.out.println(matrixString); } }
Run the above code, the output is as follows:
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
As you can see, the Arrays.deepToString() function converts the two-dimensional array into a string form and encloses it in square brackets. Each subarray is also converted to a string and separated by commas.
In addition to two-dimensional arrays, the Arrays.deepToString() function can also handle higher-dimensional arrays. The following is an example that demonstrates how to convert a three-dimensional array to a string:
public class Main { public static void main(String[] args) { int[][][] cube = { {{1, 2, 3}, {4, 5, 6}}, {{7, 8, 9}, {10, 11, 12}} }; String cubeString = Arrays.deepToString(cube); System.out.println(cubeString); } }
Run the above code, the output is as follows:
[[[1, 2, 3], [4, 5, 6]], [[7, 8, 9], [10, 11, 12]]]
As you can see, the Arrays.deepToString() function recursively Convert a three-dimensional array to string form, enclosed in square brackets. Each subarray is also converted to a string and separated by commas.
It should be noted that the Arrays.deepToString() function can only handle elements of array type. If there are elements of non-array type in the array, this function will call the element's toString() method to convert it to a string. If the array contains instances of a custom class, it is recommended to override the class's toString() method so that the instances are correctly converted to strings.
To sum up, Java's Arrays.deepToString() function is a very convenient tool that can convert multi-dimensional arrays into string form. Whether it is a two-dimensional array, a three-dimensional array, or a higher-dimensional array, the Arrays.deepToString() function can recursively convert it to a string. In the actual development process, we can use this function to conveniently perform tasks such as debugging, output, and logging.
The above is the detailed content of Convert multidimensional array to string using java's Arrays.deepToString() function. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
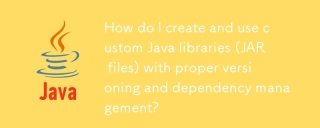
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
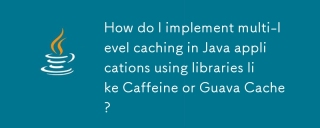
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
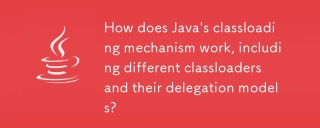
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
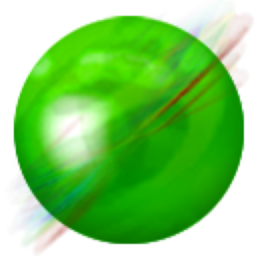
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
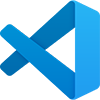
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
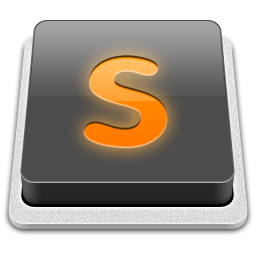
SublimeText3 Mac version
God-level code editing software (SublimeText3)
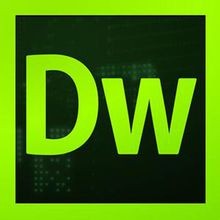
Dreamweaver CS6
Visual web development tools