


Use Java's String.matches() function to determine whether a string matches a specified regular expression
Use Java's String.matches() function to determine whether a string matches a specified regular expression
In Java, we often need to perform some pattern matching operations on strings. Regular expressions are a very powerful tool for describing and matching string patterns. The String class in Java provides a very convenient method matches() to determine whether a string matches a specified regular expression.
Let's take a look at how to use the String.matches() function to determine whether a string matches a regular expression, and understand its usage through some sample code.
First of all, we need to clarify the definition of the String.matches() function:
boolean matches(String regex)
This method matches the given regular expression with a string. A return value of true indicates a successful match, otherwise it indicates a failed match.
Next, let’s look at some sample codes to better understand how to use the String.matches() function:
Example 1: Determine whether to match a mobile phone number
public class StringMatchExample { public static void main(String[] args) { String phoneNumber1 = "12345678900"; String phoneNumber2 = "0987654321"; String phoneNumber3 = "1234567"; String regex = "1[345789]\d{9}"; System.out.println(phoneNumber1.matches(regex)); // true System.out.println(phoneNumber2.matches(regex)); // false System.out.println(phoneNumber3.matches(regex)); // false } }
In the above example, we defined a regular expression 1[345789]\d{9}
to match mobile phone numbers in mainland China. We use three string variables phoneNumber1, phoneNumber2 and phoneNumber3 to test whether they match. According to the running results, it can be seen that phoneNumber1 matches successfully, but phoneNumber2 and phoneNumber3 fail to match.
Example 2: Determine whether it matches the email address
public class StringMatchExample { public static void main(String[] args) { String email1 = "abc123@gmail.com"; String email2 = "abc@123@gmail.com"; String email3 = "abc123@.com"; String regex = "\w+@(\w+.)+[a-z]{2,3}"; System.out.println(email1.matches(regex)); // true System.out.println(email2.matches(regex)); // false System.out.println(email3.matches(regex)); // false } }
In the above example, we use the regular expression\w @(\w .) [a-z]{2,3 }
to match email addresses. We use three string variables email1, email2 and email3 to test whether they match. According to the running results, you can see that email1 matches successfully, but email2 and email3 fail.
Through the above two sample codes, we can see that it is very simple to use the String.matches() function to determine whether a string matches a specified regular expression. You only need to pass the target string and regular expression as parameters to the matches() function, and the function will return a boolean type result to indicate whether the match is successful.
It should be noted that when using the String.matches() function to determine whether a string matches a regular expression, you need to ensure that the entire string exactly matches the regular expression. If the string is only partially matched, false will also be returned.
In addition to the String.matches() function, Java also provides some other regular expression matching methods, such as String.match(), Pattern class, Matcher class, etc. These methods may be more flexible and convenient to use in complex regular expression applications.
In actual development, regular expressions are widely used. By using the String.matches() function to determine whether a string matches a specified regular expression, we can effectively perform data verification, formatting, matching and other operations. I hope the explanations and examples in this article can help you better understand and use the String.matches() function.
The above is the detailed content of Use Java's String.matches() function to determine whether a string matches a specified regular expression. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
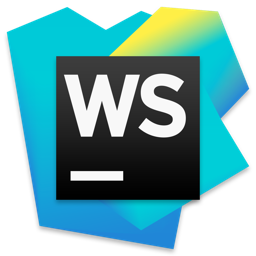
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor