Implementing PHP security verification using Laravel Passport
Using Laravel Passport to implement PHP security authentication
Introduction:
In modern web development, user authentication and authorization are very important security functions. Laravel Passport is an OAuth2-based authentication tool that can help us easily implement user authentication and authorization functions. This article explains how to implement secure authentication in PHP using Laravel Passport.
Steps:
-
Install Laravel Passport
First, make sure the Laravel framework is installed. Next, enter the project directory in the terminal and execute the following command to install the Laravel Passport package:composer require laravel/passport
After successful installation, run the following command to publish the files and database migrations required by the package:
php artisan passport:install
-
Configure Passport
Open theconfig/app.php
file and add the following service provider in theproviders
array:LaravelPassportPassportServiceProvider::class,
Then, in the
$routeMiddleware
array in theapp/Http/Kernel.php
file, add the following middleware:'client' => LaravelPassportHttpMiddlewareCheckClientCredentials::class,
Finally, in the
file:app /User.php
Add theHasApiTokens
trait ofPassport
to the /User.phpuse LaravelPassportHasApiTokens;
and introduce it in the
trait array of the class:use HasApiTokens;
Now, we have successfully configured Laravel Passport. -
First, we need to create a controller to handle user authentication requests. You can execute the following command to generate a new controller:
Create user authentication interfacephp artisan make:controller AuthController
Then, add the following method in
AuthController to handle registration and login requests: -
Create RoutesOpen the
routes/api.php -
:
Use Passport GuardOpen
config/auth.phpfile, and change the
apiguard driver to
passport -
, you can add the following route:
Use authenticated userNow, we can use the
auth:apimiddleware to authenticate users and secure related API routes. For example, in
AuthControllerpublic function profile() { $user = Auth::user(); return response()->json(['user' => $user], 200); }
Then, in
routes/api.php, add the following route:Route::get('profile', 'AuthController@profile')->middleware('auth:api');
In this way, when accessing the
/api/profile route, the user will be asked to provide a valid authentication token first.
use IlluminateHttpRequest; use IlluminateSupportFacadesAuth; class AuthController extends Controller { public function register(Request $request) { // 验证请求数据 $request->validate([ 'name' => 'required|unique:users', 'email' => 'required|email|unique:users', 'password' => 'required|min:6' ]); // 创建用户 $user = User::create([ 'name' => request('name'), 'email' => request('email'), 'password' => bcrypt(request('password')), ]); // 生成访问令牌 $token = $user->createToken('accessToken')->accessToken; // 返回响应 return response()->json(['token' => $token], 200); } public function login(Request $request) { // 验证请求数据 $credentials = request(['email', 'password']); // 检查用户凭据 if (!Auth::attempt($credentials)) { return response()->json(['message' => 'Unauthorized'], 401); } // 获取当前用户 $user = $request->user(); // 生成访问令牌 $token = $user->createToken('accessToken')->accessToken; // 返回响应 return response()->json(['user' => $user, 'token' => $token], 200); } }
Route::post('register', 'AuthController@register'); Route::post('login', 'AuthController@login')->middleware('client');
'guards' => [ 'api' => [ 'driver' => 'passport', 'provider' => 'users', ], ],
Summary:
The above is the detailed content of Implementing PHP security verification using Laravel Passport. For more information, please follow other related articles on the PHP Chinese website!
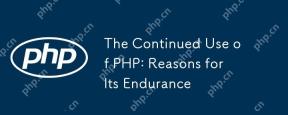
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
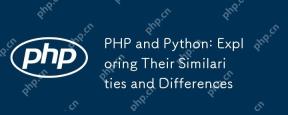
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
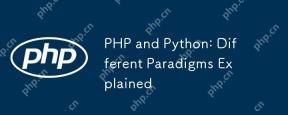
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
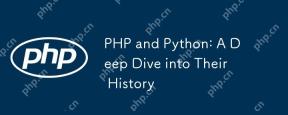
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
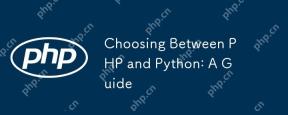
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
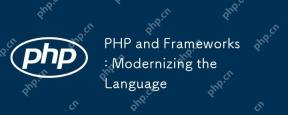
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
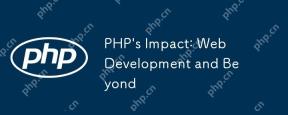
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
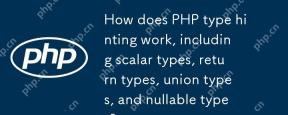
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
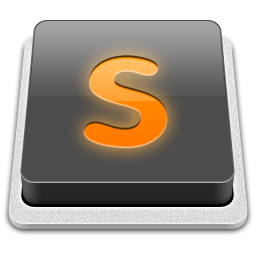
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.