PHP function introduction—fileperms(): Obtain file permissions
In PHP development, sometimes we need to obtain file permission information, such as determining whether the file is readable, writable, or executable. The PHP function fileperms() can help us achieve this function. This article will introduce the usage and examples of the fileperms() function in detail.
The fileperms() function is used to obtain file permissions. Its prototype is as follows:
string fileperms (string $filename)
Among them, $filename is the file path to obtain permission information. The function returns a string representing the file's permissions.
The following is a simple example showing how to use the fileperms() function to obtain the permissions of a file:
<?php $filename = 'test.txt'; $perms = fileperms($filename); echo "文件{$filename}的权限是:{$perms}"; ?>
In this example, we use test.txt as the file to obtain permissions . The permission information returned by the fileperms() function is stored in the variable $perms, and then the permission information is output to the user through the echo statement.
If we run the above code, the output may look like this:
文件test.txt的权限是:33204
In this example, we can see that the permission returned by the function is an integer. In fact, this integer represents the permissions of the file, with the lower 9 bits used to identify the read, write and execute permissions of the file. Among them: the first digit of
- indicates whether it is executable; the second digit of
- indicates whether it can be written; and the third digit of
- indicates whether it can be read.
The returned integer uses different bits in memory to store these three permissions, so some bit operations need to be used to obtain specific permissions.
Here is a helper function that converts the integer returned by fileperms() into a readable permission string:
<?php function format_perms($perms) { $result = ''; if (($perms & 0xC000) == 0xC000) { $result .= 's'; } elseif (($perms & 0xA000) == 0xA000) { $result .= 'l'; } elseif (($perms & 0x8000) == 0x8000) { $result .= '-'; } elseif (($perms & 0x6000) == 0x6000) { $result .= 'b'; } elseif (($perms & 0x4000) == 0x4000) { $result .= 'd'; } elseif (($perms & 0x2000) == 0x2000) { $result .= 'c'; } elseif (($perms & 0x1000) == 0x1000) { $result .= 'p'; } else { $result .= 'u'; } if (($perms & 0x0100) == 0x0100) { $result .= 'r'; } else { $result .= '-'; } if (($perms & 0x0080) == 0x0080) { $result .= 'w'; } else { $result .= '-'; } if (($perms & 0x0040) == 0x0040) { if ($perms & 0x0800) { $result .= 's'; } else { $result .= 'x'; } } else { if (($perms & 0x0800) == 0x0800) { $result .= 'S'; } else { $result .= '-'; } } if (($perms & 0x0020) == 0x0020) { $result .= 'r'; } else { $result .= '-'; } if (($perms & 0x0010) == 0x0010) { $result .= 'w'; } else { $result .= '-'; } if (($perms & 0x0008) == 0x0008) { if ($perms & 0x0400) { $result .= 't'; } else { $result .= 'x'; } } else { if (($perms & 0x0400) == 0x0400) { $result .= 'T'; } else { $result .= '-'; } } if (($perms & 0x0004) == 0x0004) { $result .= 'r'; } else { $result .= '-'; } if (($perms & 0x0002) == 0x0002) { $result .= 'w'; } else { $result .= '-'; } if (($perms & 0x0001) == 0x0001) { $result .= 'x'; } else { $result .= '-'; } return $result; } $filename = 'test.txt'; $perms = fileperms($filename); $formatted_perms = format_perms($perms); echo "文件{$filename}的权限是:{$formatted_perms}"; ?>
If we run this code, the output may look like this:
文件test.txt的权限是:-rw-r--r--
In this example, we define an auxiliary function format_perms() to convert the integer returned by fileperms() into a readable permission string. Finally, we use the echo statement to output the formatted permission string to the user.
Summary:
In PHP development, we often need to obtain file permission information. By using the fileperms() function, we can easily obtain the permissions of a file. This article details the usage of the fileperms() function and provides sample code. Using this function, we can ensure that operations on files comply with permission requirements.
I hope that through this article, you can better understand and use the fileperms() function, thereby improving your PHP development capabilities.
The above is the detailed content of PHP function introduction—fileperms(): Get file permissions. For more information, please follow other related articles on the PHP Chinese website!
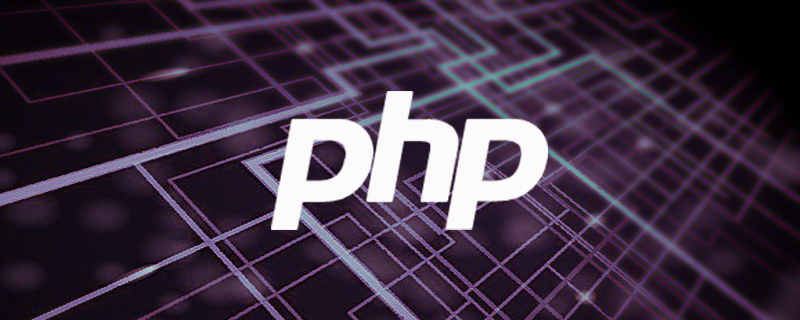
php函数返回值只能有一个。在PHP中,函数返回值使用return语句定义,语法“return 返回值;”。return语句只能返回一个参数,即函数只能有一个返回值;如果要返回多个值的话,就需在函数中定义一个数组,将返回值存储在数组中返回。
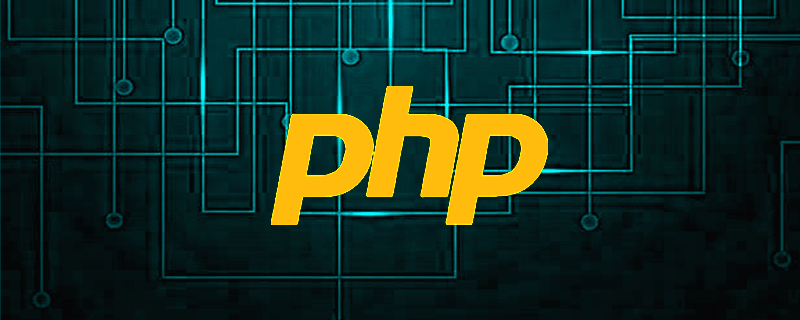
不是,php传参可以是字符串、数字、布尔值、数组等。从PHP5.6版本开始支持传递数组参数,函数的形式参数可使用“…”来表示函数可接受一个可变数量的参数,而可变参数将会被当作一个数组传递给函数,语法“function 函数名(...$arr){//执行代码}”。
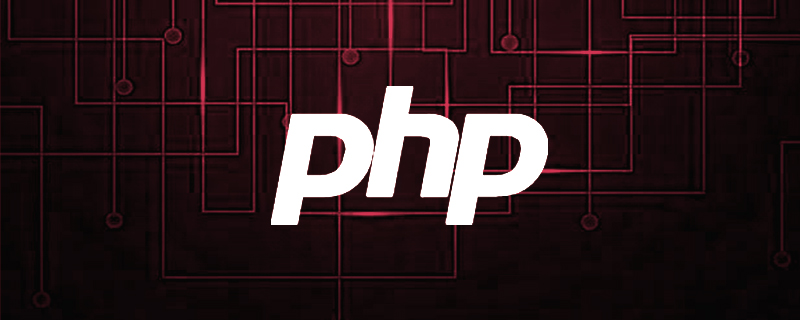
php函数的参数赋值有3种:1、值传递赋值,将实参的值复制一份再赋值给函数的形参;2、引用传递赋值,把实参的内存地址复制一份,然后传递给函数的形参,进而将实参值赋值给形参;3、直接给函数的参数指定默认值,语法“函数名(参数变量='值')”。
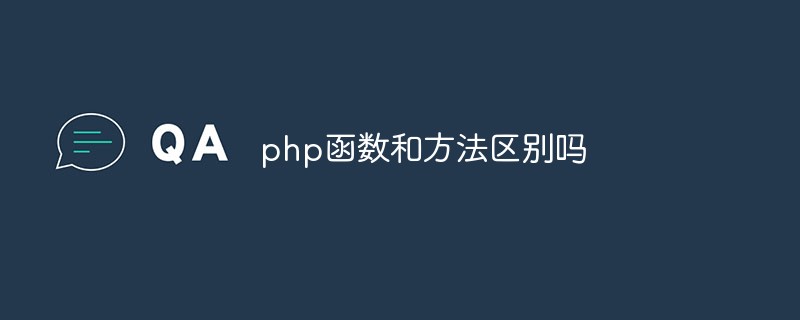
随着互联网技术的发展,PHP已经成为了非常流行的开发语言之一。身为一个PHP开发者,了解PHP函数和方法的区别是非常重要的,因为它们在编写代码的时候都是必不可少的。在本文中,我们将详细介绍PHP函数和方法的区别。
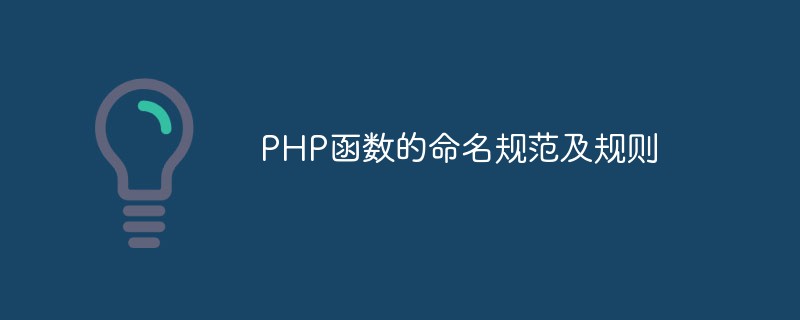
PHP作为一种非常流行的脚本语言,有着强大的函数库支持,其函数的命名规范和规则对于开发效率和代码可读性都有着重要的影响。本文将介绍PHP函数的命名规范及规则。一、命名风格在PHP中,函数名需要严格符合命名规范和规则,规范主要包括两个方面:命名风格和命名规则。1.下划线命名法下划线命名法是PHP函数命名最常用的方式,也是官方推荐的一种方式。遵循这种方式的函数名
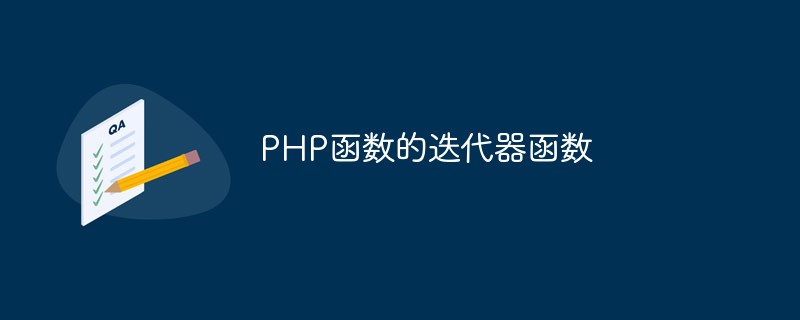
随着现代编程语言的不断发展,编程的效率和功能性也不断提高,其中PHP作为一种广泛使用的服务器端脚本语言,也在不断地更新和完善其自身的功能列表。PHP函数的迭代器函数就是其中的一种新功能,为PHP程序员提供了更加灵活和高效的编程方式。在本文中,我们将详细介绍PHP函数的迭代器函数的相关知识。什么是PHP函数的迭代器函数?在介绍PHP函数的迭代器函数之前,我们首
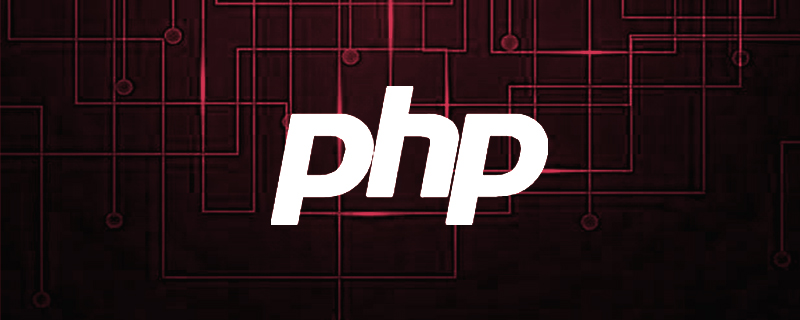
在php中,递归函数指的是自调用函数,也就是函数在函数体内部直接或间接地自己调用自己;使用递归函数时,需要在函数体中附加一个判断条件,以判断是否需要继续执行递归调用,当条件满足时会终止函数的递归调用。
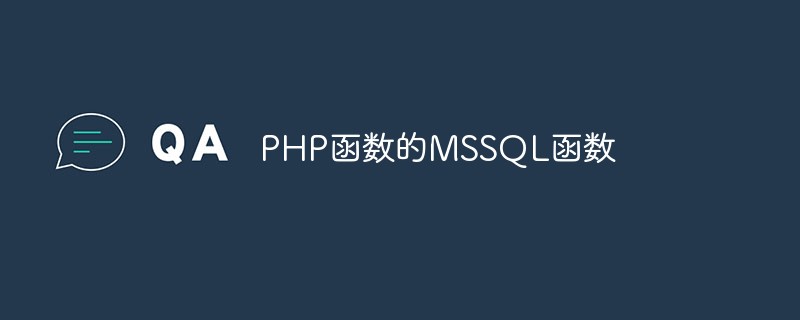
PHP是一种开源的服务器端脚本语言,通常用于开发Web应用程序。PHP具有易学易用、灵活、性能优异等优点,因此在Web开发领域得到了广泛应用。而MSSQL作为一种流行的关系型数据库管理系统,也被PHP所支持。在PHP中实现MSSQL数据库操作,需要使用MSSQL函数。MSSQL函数可用于连接数据库、执行查询语句、读写数据库中的数据等操作。接下来,将详细介绍一


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
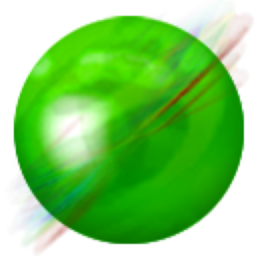
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
