


How to use PHP functions to implement user login and logout functions?
How to use PHP functions to implement user login and logout functions?
User login and logout functionality is essential when developing a website or application. As a server-side scripting language, PHP has a rich function library and flexible syntax, which can help us easily implement user login and logout functions.
This article will introduce how to use PHP functions to implement user login and logout functions so that users can log in and log out of the website safely.
- Create database
First, we need to create a database to store user information. Databases and tables can be created using relational databases such as MySQL.
Sample code:
CREATE DATABASE user_info; USE user_info; CREATE TABLE users ( id INT AUTO_INCREMENT PRIMARY KEY, username VARCHAR(255) NOT NULL, password VARCHAR(255) NOT NULL );
- Create login page
The first step in the user login function is to create a login page that allows the user to enter their username and password .
Sample code:
<!DOCTYPE html> <html> <head> <title>Login</title> </head> <body> <h1 id="Login">Login</h1> <form action="login.php" method="POST"> <label for="username">Username:</label> <input type="text" name="username" id="username" required><br> <label for="password">Password:</label> <input type="password" name="password" id="password" required><br> <input type="submit" value="Login"> </form> </body> </html>
- Create login processing page
Next, we need to create a PHP page that handles user login requests. This page will verify the username and password entered by the user and determine whether they are correct.
Sample code:
<?php session_start(); // 获取用户输入的用户名和密码 $username = $_POST['username']; $password = $_POST['password']; // 连接数据库 $mysqli = new mysqli('localhost', 'username', 'password', 'user_info'); // 查询用户信息 $query = "SELECT * FROM users WHERE username='$username' AND password='$password'"; $result = $mysqli->query($query); // 验证用户登录信息 if ($result->num_rows == 1) { // 用户登录成功 $_SESSION['username'] = $username; header('Location: welcome.php'); } else { // 用户登录失败 echo 'Invalid username or password.'; } ?>
- Create a welcome page
After the user successfully logs in, we need to create a welcome page to indicate that the user has successfully logged in.
Sample code:
<?php session_start(); if (isset($_SESSION['username'])) { $username = $_SESSION['username']; echo "Welcome, $username! "; echo '<a href="logout.php">Logout</a>'; } else { header('Location: login.php'); } ?>
- Create a logout page
Finally, we need to create a logout page to handle the user's logout request.
Sample code:
<?php session_start(); session_unset(); session_destroy(); header('Location: login.php'); ?>
So far, we have completed the steps of using PHP functions to implement user login and logout functions.
Summary:
By making reasonable use of PHP functions and database query language, we can easily implement user login and logout functions. The code examples provided above can serve as a basic framework that can be extended and modified according to specific needs. At the same time, in order to ensure user login security, we also need to add security measures such as password encryption, verification codes, and preventing SQL injection in practical applications.
The above is the detailed content of How to use PHP functions to implement user login and logout functions?. For more information, please follow other related articles on the PHP Chinese website!
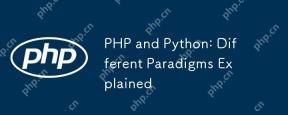
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
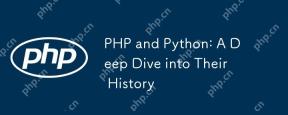
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
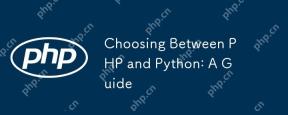
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
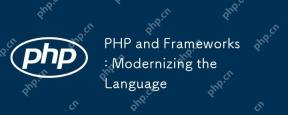
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
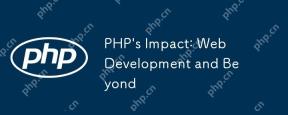
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
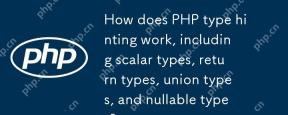
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
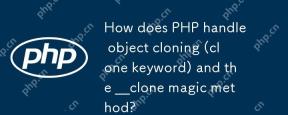
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
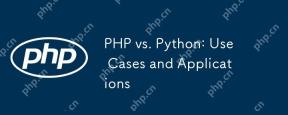
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
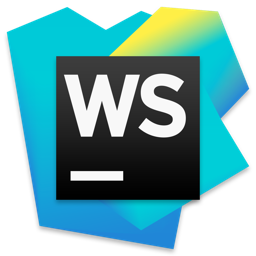
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
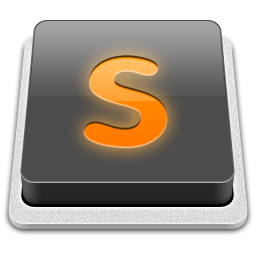
SublimeText3 Mac version
God-level code editing software (SublimeText3)