Use Java's String.isEmpty() function to determine whether a string is empty
Use Java's String.isEmpty() function to determine whether a string is empty
In programming, you often encounter the situation of determining whether a string is empty. Java provides the isEmpty() function of the String class, which can easily determine whether a string is empty.
The String class is a commonly used string processing class in Java and provides a large number of methods for processing character sequences. Among them, isEmpty() is a method in the String class, used to determine whether the string is empty. Its definition is as follows:
public boolean isEmpty() {
return value.length == 0;
}
As can be seen from the code, the isEmpty() method is implemented by judging the string Whether the length is 0 determines whether the string is empty. When the string length is 0, it is empty; otherwise, the string is not empty.
Using the isEmpty() function can easily determine whether a string is empty, avoiding the trouble of manually writing judgment conditions. The following is a simple sample code:
public class Main { public static void main(String[] args) { String str1 = ""; String str2 = "Hello World"; if (str1.isEmpty()) { System.out.println("str1为空"); } else { System.out.println("str1不为空"); } if (str2.isEmpty()) { System.out.println("str2为空"); } else { System.out.println("str2不为空"); } } }
The above code defines two strings str1 and str2, and uses the isEmpty() function to determine whether they are empty. Running this code can get the following output:
str1 is empty
str2 is not empty
As can be seen from the output, the length of str1 is 0, so it is empty; and str2's The length is 11 and is not empty.
In addition to using the isEmpty() function, you can also use the conditional statement to determine whether the string length is 0 to determine whether the string is empty. For example, the following code can be used to achieve the same function:
if (str1.length() == 0) { System.out.println("str1为空"); } else { System.out.println("str1不为空"); }
By comparing the above two implementation methods, you can find that using the isEmpty() function is more concise and intuitive. Therefore, when determining whether a string is empty, it is recommended to use the isEmpty() function.
In short, using Java's String.isEmpty() function can easily determine whether a string is empty. By calling the isEmpty() function, you can avoid the tediousness of manually writing judgment conditions and improve the readability and simplicity of the code. In actual programming, we can choose the appropriate method to determine whether a string is empty according to specific needs.
The above is the detailed content of Use Java's String.isEmpty() function to determine whether a string is empty. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
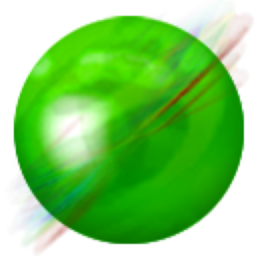
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
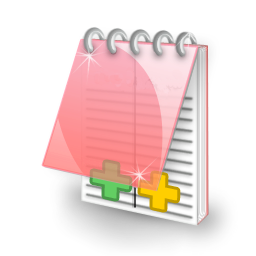
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
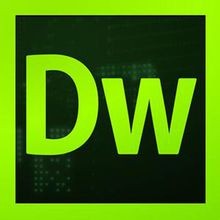
Dreamweaver CS6
Visual web development tools