


Java uses the remove() function of the ArrayList class to delete elements in the collection
ArrayList is one of the commonly used collection classes in Java. It provides an implementation of a dynamic array. ArrayList can store different types of elements and can be added and deleted at any time as needed.
In ArrayList, we often need to delete specific elements in the collection. Java provides the remove() function to implement this function. Next, we will introduce in detail how to use the remove() function of ArrayList to delete elements in the collection.
The remove() function of ArrayList has two signatures:
- boolean remove(Object o): Remove the specified element from the ArrayList.
- E remove(int index): Delete elements in ArrayList based on index position.
The following is a detailed explanation and sample code for these two functions.
- Use the remove(Object o) function to delete the specified element:
The remove(Object o) function is used to delete the specified element from the ArrayList. It iterates through the ArrayList, finds the first element equal to the specified element, and removes it from the ArrayList. If the deletion is successful, return true; otherwise, return false.
The sample code is as follows:
import java.util.ArrayList; public class ArrayListDemo { public static void main(String[] args) { ArrayList<String> list = new ArrayList<>(); list.add("apple"); list.add("banana"); list.add("orange"); System.out.println("删除之前的ArrayList:" + list); boolean result = list.remove("banana"); if(result){ System.out.println("删除成功"); }else{ System.out.println("删除失败"); } System.out.println("删除之后的ArrayList:" + list); } }
The running results are as follows:
删除之前的ArrayList:[apple, banana, orange] 删除成功 删除之后的ArrayList:[apple, orange]
As can be seen from the running results, we used the remove() function to successfully delete the element "banana" in the ArrayList ".
- Use the remove(int index) function to delete elements based on the index position:
The remove(int index) function is used to delete elements in the ArrayList based on the index position. It will remove the element at the specified index position from the ArrayList and move subsequent elements forward.
The sample code is as follows:
import java.util.ArrayList; public class ArrayListDemo { public static void main(String[] args) { ArrayList<String> list = new ArrayList<>(); list.add("apple"); list.add("banana"); list.add("orange"); System.out.println("删除之前的ArrayList:" + list); String removedElement = list.remove(1); System.out.println("被删除的元素:" + removedElement); System.out.println("删除之后的ArrayList:" + list); } }
The running results are as follows:
删除之前的ArrayList:[apple, banana, orange] 被删除的元素:banana 删除之后的ArrayList:[apple, orange]
As can be seen from the running results, we use the remove() function to successfully delete the items in the ArrayList based on the index position. Element "banana".
Summary:
Java's ArrayList class provides the remove() function for removing elements from the collection. We can delete elements in ArrayList based on element value or index position. Use the remove() function to easily delete elements in ArrayList, making collection element management more flexible and efficient.
The above is an example of using the remove() function of the ArrayList class to delete elements in the collection. I hope this article can help you understand and use the remove() function provided by the ArrayList class.
The above is the detailed content of Java uses the remove() function of the ArrayList class to delete elements from the collection. For more information, please follow other related articles on the PHP Chinese website!
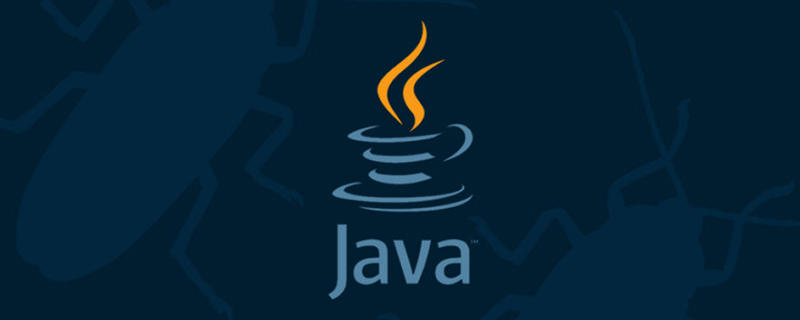
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
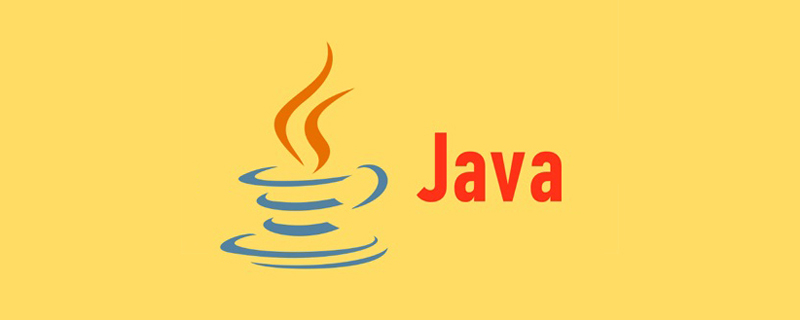
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
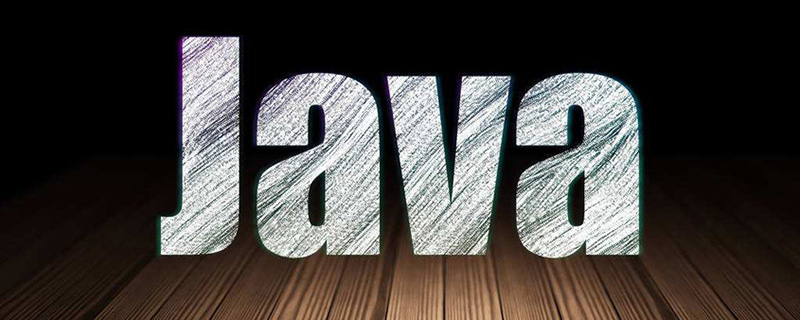
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
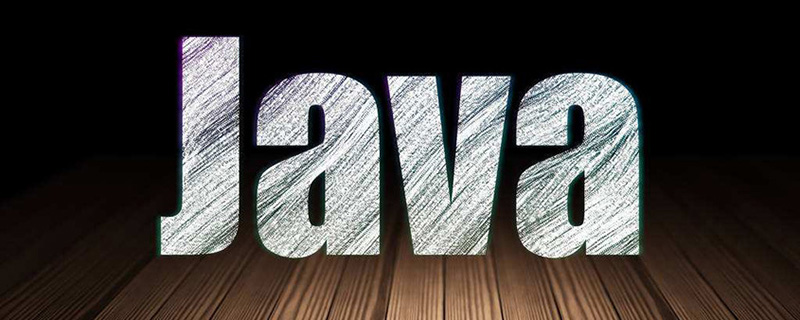
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
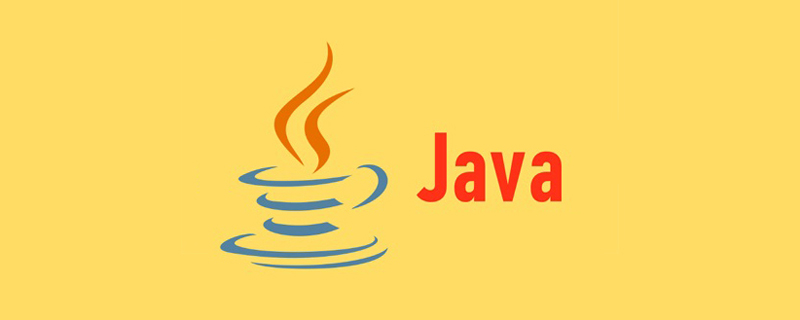
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
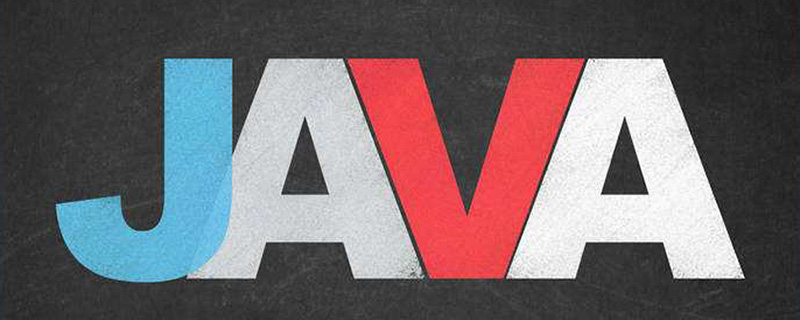
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
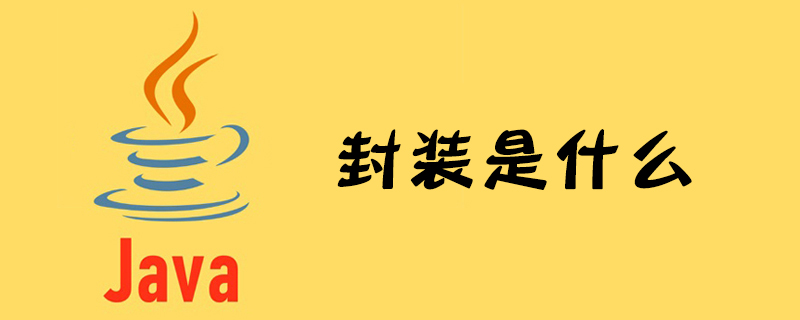
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
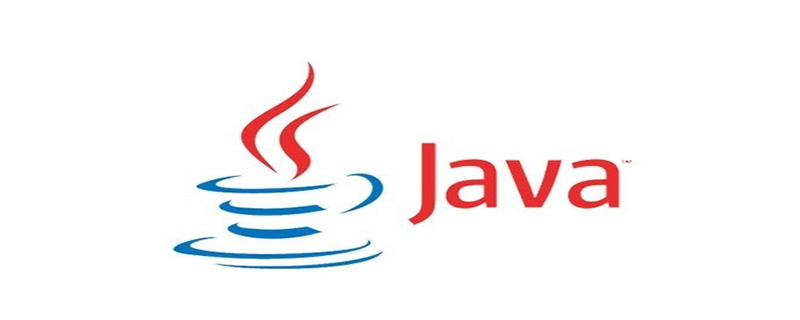
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
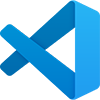
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
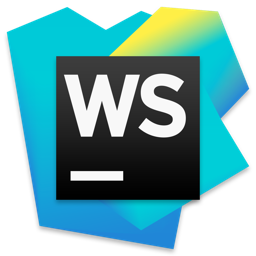
WebStorm Mac version
Useful JavaScript development tools
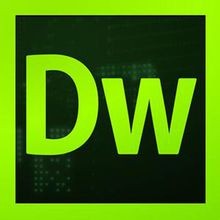
Dreamweaver CS6
Visual web development tools
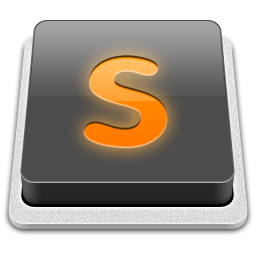
SublimeText3 Mac version
God-level code editing software (SublimeText3)
