What are the commonly used methods for traversing arrays in PHP?
The operating environment of this article: Windows 10 system, php8.1.3 version, dell g3 computer.
PHP is a widely used server-side scripting language that provides rich array processing methods that allow developers to easily traverse and operate arrays. In this article, we will introduce some commonly used array traversal methods in PHP.
1. Foreach loop:
The foreach loop is one of the most commonly used methods of traversing arrays in PHP. It can traverse numeric index arrays and associative arrays and obtain the values in the array one by one.
Syntax:
foreach ($array as $value) { // do something with $value }
Example:
$numbers = array(1, 2, 3, 4, 5); foreach ($numbers as $number) { echo $number; }
Output: 12345
$fruits = array("apple" => "red", "banana" => "yellow", "grape" => "purple"); foreach ($fruits as $fruit => $color) { echo $fruit . " is " . $color; }
Output: apple is red, banana is yellow, grape is purple
2. for loop:
The for loop is more useful when traversing a numerical index array because it can access the index of the array during the iteration process.
Syntax:
for ($i = 0; $i < count($array); $i++) { // do something with $array[$i] }
Example:
$numbers = array(1, 2, 3, 4, 5); for ($i = 0; $i < count($numbers); $i++) { echo $numbers[$i]; }
Output: 12345
3. while loop:
A while loop is a more flexible way of traversing an array that iterates continuously when a specific condition is met.
Syntax:
$i = 0; while ($i < count($array)) { // do something with $array[$i] $i++; }
Example:
$numbers = array(1, 2, 3, 4, 5); $i = 0; while ($i < count($numbers)) { echo $numbers[$i]; $i++; }
Output: 12345
4. array_map function:
The array_map function is a higher-order function that applies a callback function to each element of an array and returns a new array.
Syntax:
$newArray = array_map($callback, $array);
Example:
$numbers = array(1, 2, 3, 4, 5); $newArray = array_map(function($number) { return $number * $number; }, $numbers); print_r($newArray);
Output: Array([0] => 1 [1] => 4 [2] => 9 [ 3] => 16 [4] => 25)
5. array_walk function:
The array_walk function can apply a user to each element of the array Custom functions.
Syntax:
array_walk($array, $callback);
Example:
$numbers = array(1, 2, 3, 4, 5); function square($value, $key) { echo "{$key} => {$value} * {$value} = " . $value * $value"; } array_walk($numbers, "square");
Output: 0 => 1 * 1 = 1, 1 => 2 * 2 = 4, 2 => 3 * 3 = 9, 3 => 4 * 4 = 16, 4 => 5 * 5 = 25
6. array_filter function:
array_filter function Can be used to filter elements in an array and return a new array composed of elements that meet the conditions.
Syntax:
$newArray = array_filter($array, $callback);
Example:
$numbers = array(1, 2, 3, 4, 5); $newArray = array_filter($numbers, function($number) { return $number % 2 == 0; }); print_r($newArray);
Output: Array([1] => 2 [3] => 4)
Above It is a commonly used method of traversing arrays in PHP. Through these methods, developers can flexibly manipulate and process arrays. Whether it is a numerically indexed array or an associative array, PHP provides simple and powerful tools for us to use. Being proficient in these methods will help improve the efficiency and readability of your code.
The above is the detailed content of What are the commonly used methods for traversing arrays in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
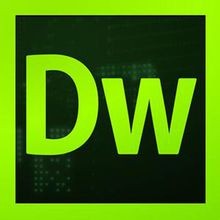
Dreamweaver CS6
Visual web development tools
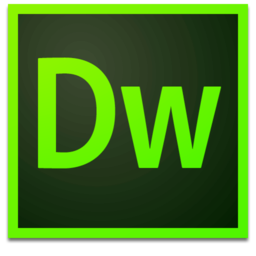
Dreamweaver Mac version
Visual web development tools
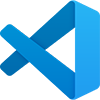
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
