


Convert multidimensional arrays to strings using the deepToString() method of the Arrays class in Java
Use the deepToString() method of the Arrays class in Java to convert multidimensional arrays into strings
In Java programming, processing multidimensional arrays is a common task. When we want to convert a multidimensional array into a string, we can do it using the deepToString() method in the Arrays class. The deepToString() method can convert a multi-dimensional array into a string representation, which is convenient for us to output or debug.
In the following example, I will demonstrate how to use the deepToString() method to convert arrays of different dimensions.
First, let us define a one-dimensional array:
int[] arr = {1, 2, 3, 4, 5};
The sample code for converting a one-dimensional array to a string using the deepToString() method is as follows:
String arrString = Arrays.deepToString(arr); System.out.println(arrString);
The output result will be: [1, 2, 3, 4, 5]
Next, we define a two-dimensional array:
int[][] matrix = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} };
Use the deepToString() method to convert the two-dimensional array The sample code for a string is as follows:
String matrixString = Arrays.deepToString(matrix); System.out.println(matrixString);
The output result will be: [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
We can see that the deepToString() method also converts each element of the two-dimensional array into a string, ultimately forming a string representation of a multi-dimensional array.
Next, let’s look at an example of a higher-dimensional array:
int[][][] cube = { { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} }, { {10, 11, 12}, {13, 14, 15}, {16, 17, 18} } };
The example code for using the deepToString() method to convert a three-dimensional array into a string is as follows:
String cubeString = Arrays.deepToString(cube); System.out.println(cubeString);
The output result will be: [[[1, 2, 3], [4, 5, 6], [7, 8, 9]], [[10, 11, 12], [13, 14, 15] , [16, 17, 18]]]
Similarly, the deepToString() method also converts each element of the three-dimensional array into a string, ultimately forming a string representation of a multi-dimensional array.
Through these examples, we can see that it is very convenient to use the deepToString() method of the Arrays class to convert multi-dimensional arrays into strings. Whether it is a one-dimensional array, a two-dimensional array, or a higher-dimensional array, it can be implemented using the deepToString() method. This is useful both for output and debugging.
It should be noted that the deepToString() method recursively converts all elements of a multi-dimensional array into strings, so it may cause performance problems when processing large-scale multi-dimensional arrays. Therefore, in actual projects, it may be necessary to choose an appropriate conversion method according to the situation.
The above is the detailed content of Convert multidimensional arrays to strings using the deepToString() method of the Arrays class in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
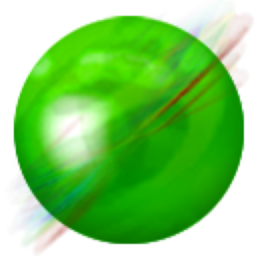
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
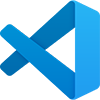
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.