


Use java's ArrayList.subList() function to obtain the sublist of the specified range of ArrayList
In Java programming, ArrayList is a very commonly used data structure, which can be used to store a set of data, and can Add or remove elements dynamically. Sometimes, we need to get some elements in the ArrayList, which can be achieved using the subList() function.
The definition of the subList() function is as follows:
List<E> subList(int fromIndex, int toIndex)
Among them, fromIndex
represents the starting index (inclusive) of the sublist, toIndex
represents the sublist Ending index of the list (exclusive). By calling this function, we can get the sublist from fromIndex
to toIndex-1
. The returned sublist is a new List instance, which still points to the original ArrayList, and modifications to the sublist will be reflected in the original ArrayList.
The following is a sample code for sublist operation on ArrayList:
import java.util.ArrayList; import java.util.List; public class ArrayListExample { public static void main(String[] args) { // 创建一个包含整数的ArrayList ArrayList<Integer> numbers = new ArrayList<>(); numbers.add(1); numbers.add(2); numbers.add(3); numbers.add(4); numbers.add(5); // 获取ArrayList的子列表 List<Integer> subList = numbers.subList(1, 4); System.out.println("原始ArrayList中的元素:"); for (Integer num : numbers) { System.out.print(num + " "); } System.out.println(); System.out.println("子列表中的元素:"); for (Integer num : subList) { System.out.print(num + " "); } System.out.println(); // 修改子列表中的元素 subList.set(0, 10); subList.set(1, 20); System.out.println("修改过后的原始ArrayList中的元素:"); for (Integer num : numbers) { System.out.print(num + " "); } System.out.println(); } }
Run the above code, the output result is:
原始ArrayList中的元素: 1 2 3 4 5 子列表中的元素: 2 3 4 修改过后的原始ArrayList中的元素: 1 10 20 4 5
As can be seen from the running results, through subList The sublist obtained by the () function has an impact on the original ArrayList. When we modify an element in the sublist, the corresponding element in the original ArrayList will also be modified.
It should be noted that the sublist returned by subList() cannot be added or deleted, otherwise an UnsupportedOperationException
exception will be thrown.
As a result, the use of the ArrayList.subList() function allows the ArrayList data to be accurately valued according to a certain range, which facilitates subsequent operations. When using it, you need to pay attention to the range of the index and its impact on the original ArrayList in order to correctly obtain, modify and process elements in the sublist.
The above is the detailed content of Use java's ArrayList.subList() function to obtain the sublist of the specified range of ArrayList. For more information, please follow other related articles on the PHP Chinese website!
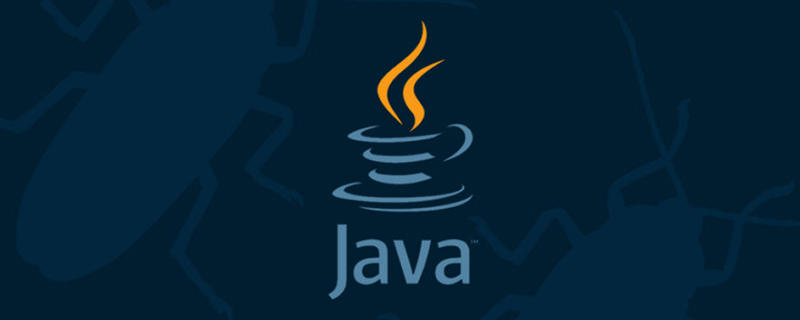
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
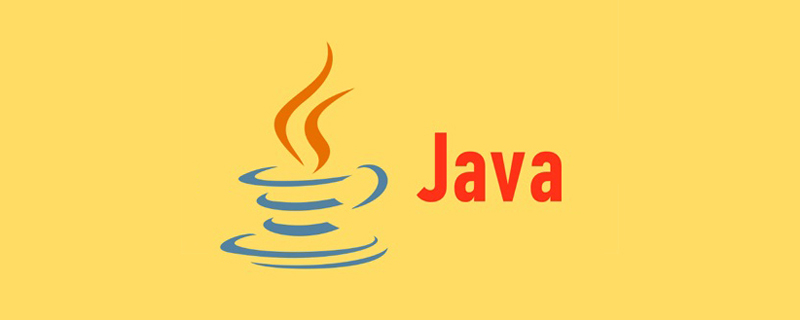
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
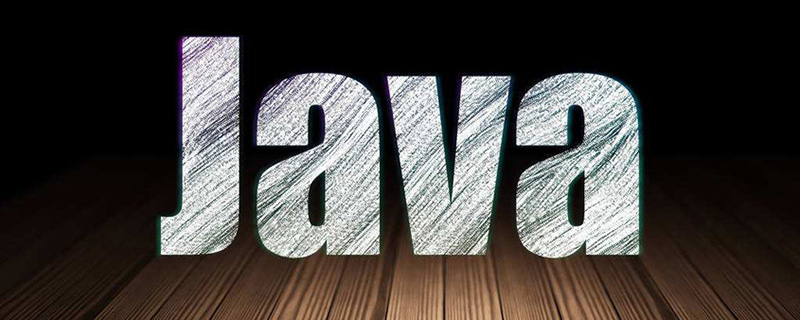
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
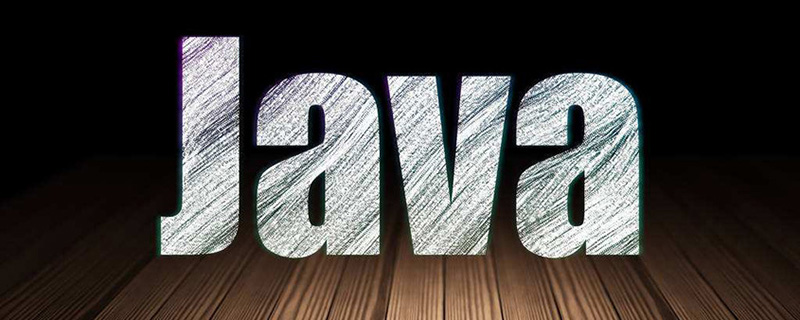
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
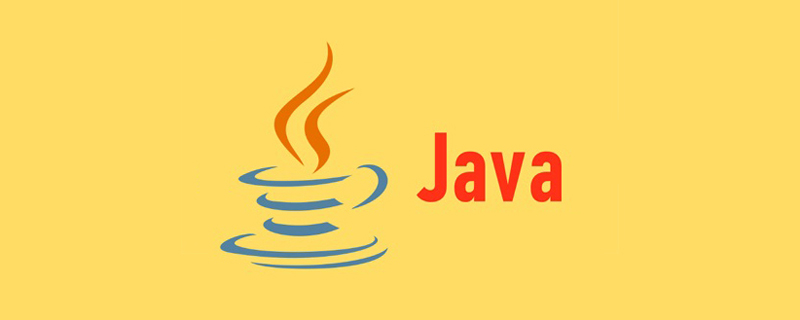
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
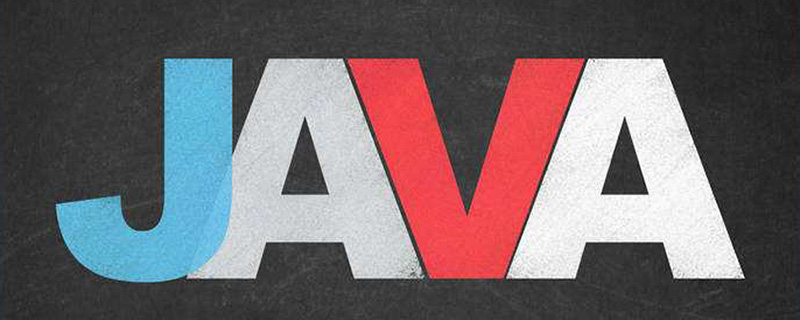
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
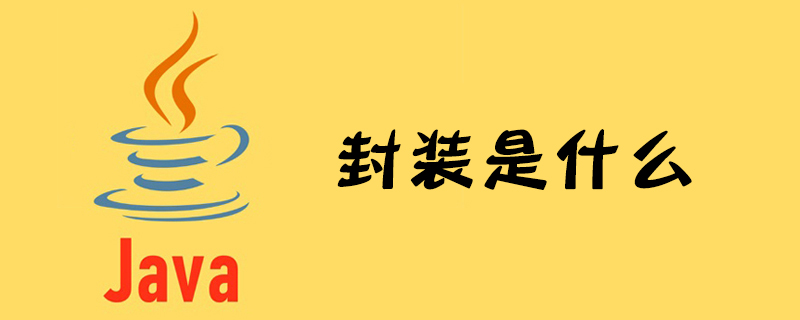
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
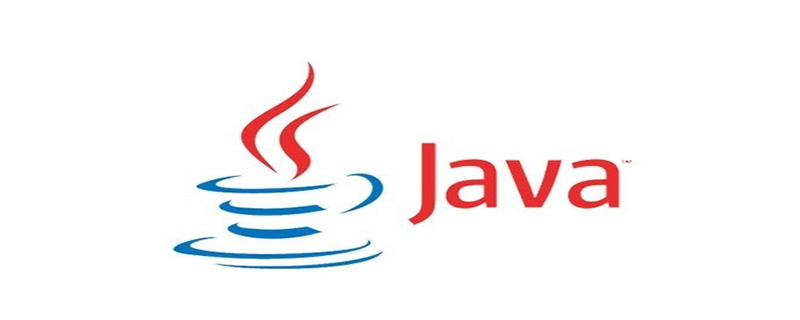
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
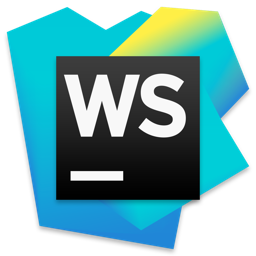
WebStorm Mac version
Useful JavaScript development tools
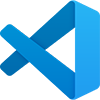
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
