


Use the ioutil.ReadFile function to read the file content and return a string and file information object list
In the Go language, we can use the ioutil.ReadFile function to read the file content. This function returns a byte array of the file contents. This function is very convenient, but may have some performance issues, especially when the file is large. If we want to obtain file information (such as file name, size, modification time, etc.) at the same time, we can use the os.Stat function to obtain the file information object.
The following is a sample code that demonstrates how to use the ioutil.ReadFile function to read the file content and return a list containing file information objects:
package main import ( "fmt" "io/ioutil" "log" "os" ) func main() { files, err := ioutil.ReadDir("/path/to/directory") if err != nil { log.Fatal(err) } for _, file := range files { if !file.IsDir() { fileContent, err := ioutil.ReadFile(file.Name()) if err != nil { log.Fatal(err) } fmt.Printf("文件名:%s ", file.Name()) fmt.Printf("文件大小:%d 字节 ", file.Size()) fmt.Printf("修改时间:%s ", file.ModTime().String()) fmt.Printf("文件内容:%s ", string(fileContent)) fmt.Println("--------------") } } }
In the above code, we first use The ioutil.ReadDir function reads files and directories in the specified directory and returns a list of file information objects. Then, we traverse the file information object list, and for each non-directory file, we use the ioutil.ReadFile function to read the file content, and print the file information and content through the fmt.Printf function.
It should be noted that "/path/to/directory" in the above example code should be replaced with the specific directory path. In addition, some error handling code may need to be added to the code to handle possible errors.
By using the ioutil.ReadFile function, we can easily read the contents of the file; and by using the os.Stat function, we can obtain the file information at the same time. The combination of the two can help us better handle the needs of file reading and information acquisition. I hope the sample code in this article can be helpful to you.
The above is the detailed content of Use the ioutil.ReadFile function to read the file content and return a string and a list of file information objects. For more information, please follow other related articles on the PHP Chinese website!
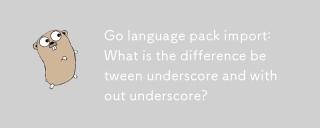
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
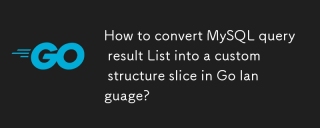
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
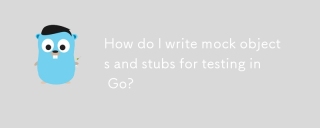
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
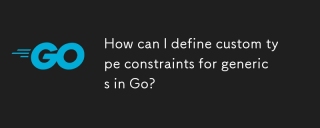
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
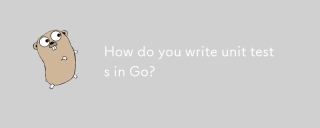
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
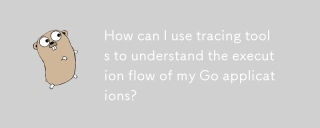
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
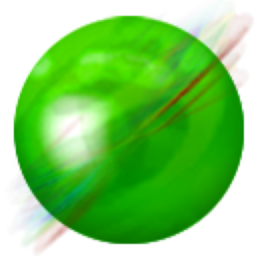
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
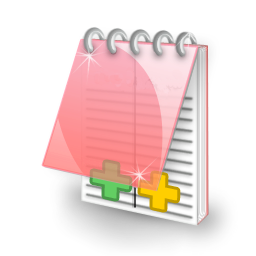
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
